Typeerror: Object Of Type Int64 Is Not Json Serializable
When working with JSON (JavaScript Object Notation) in Python, you may encounter a common error message that says “TypeError: object of type ‘int64’ is not JSON serializable.” This error message occurs when you try to convert an ‘int64’ object to JSON format, but it fails because JSON serialization does not support this specific data type.
In Python, ‘int64’ refers to a 64-bit signed integer data type represented by the NumPy library. It is commonly used in data analysis and scientific computing, but when it comes to serializing data into JSON format, it presents a challenge.
JSON natively supports basic data types like strings, numbers, booleans, lists, and dictionaries. However, it does not have a built-in support for more complex data types like ‘int64’. Therefore, when you try to convert a ‘int64’ object to JSON, it throws the TypeError because it cannot directly serialize this data type.
Reasons behind the TypeError: object of type ‘int64’ is not JSON serializable
There are several reasons why you might encounter this TypeError:
1. Complex Data Structures: The ‘int64’ data type is often used to represent large numerical values or arrays in scientific computing. These complex data structures are not compatible with JSON serialization.
2. Third-Party Libraries: If you are using a third-party library in your code that returns ‘int64’ objects, those objects may not be JSON serializable. In such cases, you would need to find a workaround to handle the serialization.
3. Data Type Mismatch: Sometimes, this error can occur due to a mismatch between the expected data type and the actual data type. For example, if you mistakenly assign an ‘int’ value to an ‘int64’ variable, it can cause this error when trying to serialize it to JSON.
Handling the TypeError: object of type ‘int64’ is not JSON serializable
Fortunately, there are several ways to handle this type of error:
1. Convert to Compatible Types: One solution is to convert the ‘int64’ object to a compatible data type before serializing it to JSON. You can use the `astype()` method provided by the NumPy library to convert ‘int64’ to ‘int’ or other supported data types.
2. Custom Serialization: Another approach is to write custom serialization functions that convert ‘int64’ objects to JSON serializable types. You can create a custom function that maps ‘int64’ objects to their desired JSON serializable form.
3. Convert to native Python types: If you don’t specifically require the ‘int64’ data type, you can convert them to native Python types like regular ‘int’. This can be done using the `item()` method provided by NumPy or by converting the ‘int64’ object to a regular ‘int’ using the `int()` function.
Converting ‘int64’ objects to JSON serializable types
To convert ‘int64’ objects to JSON serializable types, you can use the following techniques:
1. Using astype() method: The `astype()` method provided by the NumPy library allows you to convert ‘int64’ objects to other types. For example, you can convert an ‘int64’ object to a regular ‘int’ using the following code:
“`python
import numpy as np
int64_value = np.int64(42)
int_value = int64_value.astype(int)
“`
2. Using item() method: The `item()` method provided by NumPy allows you to convert a single element ‘int64’ object to a regular ‘int’. Here’s an example:
“`python
import numpy as np
int64_value = np.int64(42)
int_value = int64_value.item()
“`
Custom serialization for ‘int64’ objects
If you need to handle ‘int64’ objects in a specific way during serialization, you can write custom serialization functions. These functions convert ‘int64’ objects to their desired JSON serializable form.
Here’s an example of a custom serialization function for converting ‘int64’ objects to regular ‘int’:
“`python
import numpy as np
import json
def custom_serializer(obj):
if isinstance(obj, np.int64):
return int(obj)
raise TypeError(f”Object of type {obj.__class__.__name__} is not JSON serializable”)
int64_value = np.int64(42)
json_value = json.dumps(int64_value, default=custom_serializer)
“`
In this example, the `custom_serializer()` function checks if the object is of type ‘int64’. If it is, it converts it to a regular ‘int’ before serializing it to JSON. If the object is not an ‘int64’, it raises a TypeError.
Troubleshooting common causes of the TypeError: object of type ‘int64’ is not JSON serializable
Here are some common causes of this TypeError and their troubleshooting steps:
1. Object of type int64 is not JSON serializable: This error occurs when you directly try to convert an ‘int64’ object to JSON. To resolve this, use the techniques mentioned earlier to convert it to a compatible data type.
2. Object of type is not JSON serializable: If you encounter this error without a specific type mentioned, it means the object you are trying to serialize is not supported by JSON serialization. Make sure you are passing the correct object type.
3. Object of type int is not json serializable: This error occurs when you mistakenly assign an ‘int’ value to an ‘int64’ variable. Convert the ‘int’ value to ‘int64’ using `np.int64()` to resolve this issue.
4. Object of type int32 is not JSON serializable: The ‘int32’ data type also faces the same issue as ‘int64’. Handle it in a similar way by converting it to a compatible type before serialization.
5. Object of type float32 is not JSON serializable: Similar to ‘int64’, ‘float32’ is not supported by JSON serialization. Convert the ‘float32’ object to a compatible data type before serializing.
Conclusion
In conclusion, the “TypeError: object of type ‘int64’ is not JSON serializable” occurs when you try to convert an ‘int64’ object to JSON, but JSON serialization does not support this data type. To resolve this error, you can convert ‘int64’ objects to compatible types, write custom serialization functions, or convert them to native Python types. Understanding the reasons behind this error and the available solutions will help you handle this issue effectively.
FAQs
Q: What does the “object of type ‘int64’ is not JSON serializable” error mean?
A: This error message indicates that you are trying to convert an ‘int64’ object to JSON, but this data type is not directly supported by JSON serialization.
Q: Can I convert ‘int64’ objects to JSON serializable types?
A: Yes, you can convert ‘int64’ objects to JSON serializable types by using the `astype()` method provided by the NumPy library or by writing custom serialization functions.
Q: How do I handle the “object of type ‘int64’ is not JSON serializable” error?
A: To handle this error, you can convert ‘int64’ objects to compatible types, write custom serialization functions, or convert them to native Python types like regular ‘int’.
Q: What are some common causes of the “object of type ‘int64’ is not JSON serializable” error?
A: Common causes of this error include using complex data structures, third-party libraries returning ‘int64’ objects, and mismatches between expected data types and actual data types.
Q: How do I troubleshoot the “object of type ‘int64’ is not JSON serializable” error?
A: Troubleshoot this error by ensuring you are passing the correct object type, converting ‘int64’ objects to compatible types, or writing custom serialization functions for specific handling.
Python : Python – Typeerror: Object Of Type ‘Int64’ Is Not Json Serializable
Keywords searched by users: typeerror: object of type int64 is not json serializable Object of type int64 is not JSON serializable, Object of type is not JSON serializable, Object of type int is not json serializable, Object of type int32 is not JSON serializable, Object of type float32 is not JSON serializable, Astype int64 Python, Numpy int64 to int, Int64 to int python
Categories: Top 50 Typeerror: Object Of Type Int64 Is Not Json Serializable
See more here: nhanvietluanvan.com
Object Of Type Int64 Is Not Json Serializable
JSON (JavaScript Object Notation) is a widely used data interchange format that is lightweight, easy to read, and easy to parse. It is commonly used for transmitting data between a server and web application, as well as for storing configuration data. However, one common error that developers encounter when working with JSON is the “Object of type int64 is not JSON serializable” error.
This error occurs when attempting to serialize or encode an int64 object in JSON format. Int64 is a data type used to represent signed 64-bit integers in many programming languages, including Python. JSON, however, natively supports only a few basic data types such as strings, numbers, boolean values, arrays, and objects. Therefore, when we try to convert an int64 object to JSON, it throws an error because int64 is not one of the supported data types.
There are a few different scenarios in which this error might occur. Let’s take a closer look at each of them:
1. Serialization of int64 in Python:
Python is a popular programming language that is widely used for web development, data analysis, and scientific computing. When working with Python, you may encounter this error when using the built-in json module to serialize an int64 object.
To resolve this issue, you can simply convert the int64 object to a supported JSON data type before serializing. For example, you can convert the int64 object to a regular integer using the int() function.
Here’s an example:
“`python
import json
import numpy as np
int64_value = np.int64(42)
json_value = int(int64_value) # Convert int64 to int
json_string = json.dumps(json_value) # Serialize to JSON string
print(json_string)
“`
2. Interaction between different programming languages:
When working with multiple programming languages or platforms, you may come across this error when trying to send data that includes int64 values from one language to another.
In this case, you’ll need to convert the int64 value to a suitable data type in the language you’re working with before attempting to serialize it. For example, in JavaScript, you can convert an int64 to a string using the toString() function.
Here’s an example using JavaScript:
“`javascript
var int64Value = BigInt(42);
var stringValue = int64Value.toString(); // Convert int64 to string
var jsonObject = JSON.stringify({ value: stringValue }); // Serialize to JSON
console.log(jsonObject);
“`
3. Usage of libraries or frameworks:
Another situation where this error might occur is when using various libraries or frameworks that internally serialize or encode data in JSON format. Some of these libraries may not provide direct support for int64 types, resulting in the error.
To solve this, you can check if the library or framework provides any specific methods or options to handle int64 values. If not, you may need to perform a manual conversion to a suitable data type before passing it to the library or framework.
FAQs:
Q1. Why am I receiving the “Object of type int64 is not JSON serializable” error when using Pandas?
A: Pandas is a popular data manipulation library in Python, often used for data analysis and cleaning. When attempting to serialize a DataFrame or Series object containing int64 values using the to_json() method in Pandas, the error might occur. To fix this, you can convert the int64 values to regular integers before serializing.
Q2. How can I handle int64 values in Django REST Framework serializers?
A: Django REST Framework is a powerful toolkit for building APIs using Django. When using serializers in Django REST Framework, you may encounter the “Object of type int64 is not JSON serializable” error when serializing models containing int64 fields. One solution is to define a custom serializer field that converts the int64 value to a regular integer before serializing.
Here’s an example:
“`python
from rest_framework import serializers
class CustomInt64Field(serializers.Field):
def to_representation(self, value):
return int(value)
class MyModelSerializer(serializers.ModelSerializer):
my_int64_field = CustomInt64Field()
class Meta:
model = MyModel
fields = (‘my_int64_field’, ‘other_fields’)
“`
In conclusion, the “Object of type int64 is not JSON serializable” error occurs when attempting to serialize or encode an int64 object in JSON format. This error can be resolved by converting the int64 value to a suitable data type, such as an integer or a string, before serializing. Paying attention to the data types you’re working with and understanding the limitations of JSON serialization will help you avoid this error in your code.
Object Of Type Is Not Json Serializable
In the realm of programming, one often encounters the term “JSON serializable” when working with data. JSON, short for JavaScript Object Notation, is a popular data interchange format used for transmitting and storing data. It is widely utilized in web development, APIs, and numerous other applications. However, there may be instances when an error message such as “Object of type is not JSON serializable” pops up, causing frustration and confusion among programmers. In this article, we will delve into this error, understand its causes, and explore possible solutions to rectify it.
Understanding JSON Serialization
Before we delve deeper into the “Object of type is not JSON serializable” error, let’s have a brief overview of JSON serialization. Serialization is the process of converting an object to a format that can be easily stored, transmitted, or persisted. JSON serialization specifically refers to converting an object into a JSON string, which can later be deserialized to obtain the original object.
JSON serialization is facilitated by various programming languages and frameworks, as it enables efficient data transfer and storage. The process of JSON serialization involves traversing the object’s attributes and transforming them into a JSON-compatible format, such as strings, numbers, booleans, arrays, or nested objects. However, not all objects can be serialized into JSON directly, leading to the error message “Object of type is not JSON serializable.”
Causes of the Error
The “Object of type is not JSON serializable” error typically occurs when attempting to serialize an object that does not have a direct JSON representation. Some common causes of this error include:
1. Custom objects without a defined serialization method: When working with custom objects or classes, it is necessary to define the serialization method explicitly. Failure to do so results in the error, as the serialization engine does not know how to handle the object’s attributes.
2. Objects with non-serializable attributes: JSON serialization can handle basic data types like strings, numbers, booleans, and arrays efficiently. However, certain complex data types, such as datetime objects or file objects, are not native to JSON serialization. Hence, attempting to serialize an object containing such non-serializable attributes triggers the error.
3. Circular references: Circular references occur when objects reference each other in a loop-like structure. If such a reference exists within an object being serialized, the serialization process fails, resulting in the error.
Solutions to the Error
Now that we understand the potential causes of the “Object of type is not JSON serializable” error, let’s explore some solutions to rectify it:
1. Implement the `json_serializable` method: If you are working with custom objects, ensure they have a `json_serializable` method defined. This method should return a JSON-compatible representation of the object’s attributes. By implementing this method, you enable the serialization engine to traverse the object correctly and serialize it into a JSON string.
2. Remove non-serializable attributes: If the error occurs due to non-serializable attributes within an object, consider excluding or transforming these attributes before attempting to serialize the object. For example, you could convert a datetime object to a string representation or extract relevant properties from a file object.
3. Use JSON serialization libraries or modules: In many programming languages, there are specific libraries or modules available that handle serialization and deserialization seamlessly. Utilizing these libraries, such as `json` in Python or `Gson` in Java, can simplify the serialization process and handle complex data types automatically.
4. Handle circular references: Circular references can be more complex to resolve, as they require reorganizing or breaking the circular structure. One approach involves identifying and excluding the circular references before serialization. Alternatively, you can employ techniques like lazy loading, where you delay the serialization of specific attributes until after the main serialization process.
FAQs
Q1. What is the purpose of JSON serialization?
JSON serialization is used to convert an object into a JSON string, enabling efficient storage, transmission, and persistence of data. It plays a crucial role in web development, APIs, and inter-process communication.
Q2. Is JSON serialization supported in all programming languages?
JSON serialization is widely supported across different programming languages. Most languages offer native support or provide libraries and modules to handle JSON serialization effectively.
Q3. How can I identify the non-JSON serializable attributes in an object?
By examining the object’s attributes and their data types, you can identify which attributes might cause serialization issues. Complex data types, such as datetime objects or file objects, are often incompatible with JSON serialization.
Q4. Can I customize the serialization process for non-JSON serializable attributes?
Yes, it is possible to define custom serialization methods for non-JSON serializable attributes. By implementing these methods, you can transform the attributes into a JSON-compatible format before serialization.
Q5. Are there any performance impacts of JSON serialization?
Serialization, in general, incurs a performance cost, as it involves traversing an object’s attributes and transforming them into a serialized format. However, the impact varies depending on factors like object size, complexity, and the efficiency of the serialization implementation.
In conclusion, encountering the “Object of type is not JSON serializable” error can be frustrating, but understanding its causes and implementing the appropriate solutions can help rectify the issue. By defining serialization methods, handling non-serializable attributes, and utilizing serialization libraries, you can overcome this error and ensure smooth JSON serialization in your programming endeavors.
Images related to the topic typeerror: object of type int64 is not json serializable
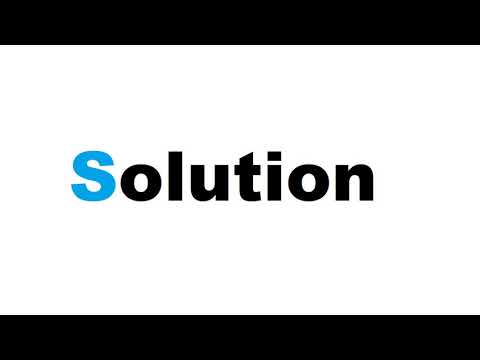
Found 16 images related to typeerror: object of type int64 is not json serializable theme
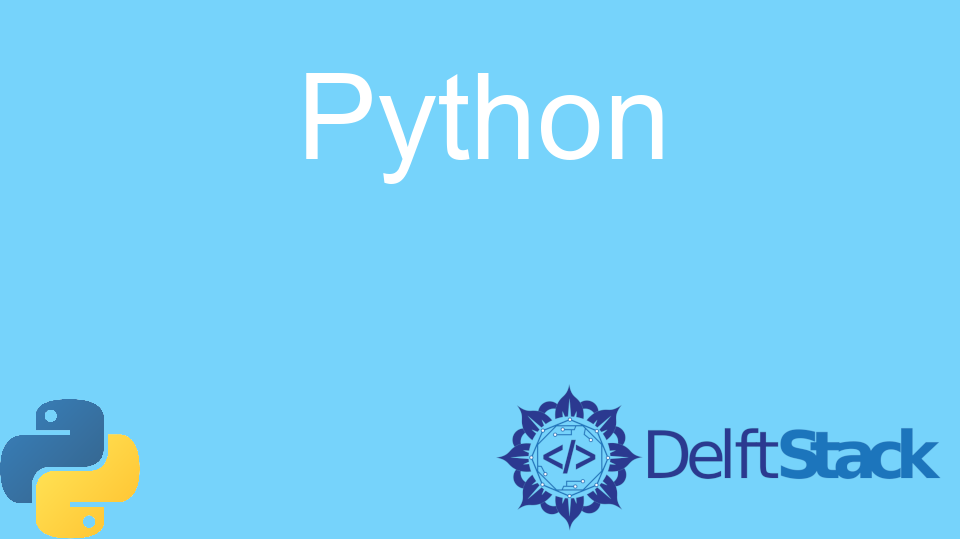



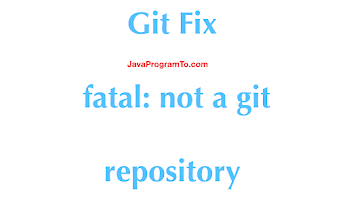
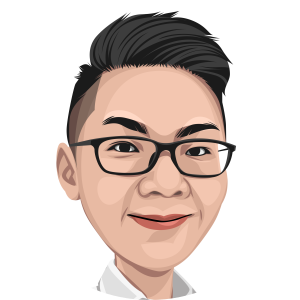
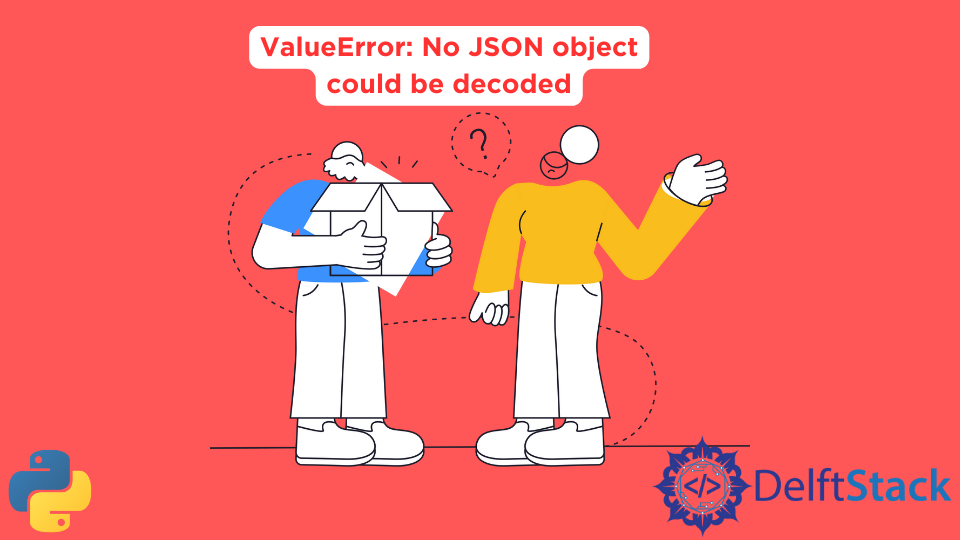
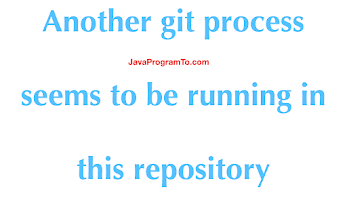

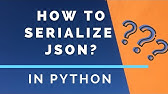
![Typeerror: object of type int64 is not json serializable [SOLVED] Typeerror: Object Of Type Int64 Is Not Json Serializable [Solved]](https://itsourcecode.com/wp-content/uploads/2021/01/IT-SOURCECODE_ICON-07.jpg)
![Errors] - TypeError: Object of type int64 is not JSON serializable Errors] - Typeerror: Object Of Type Int64 Is Not Json Serializable](https://blog.kakaocdn.net/dn/XPFji/btq3UG5xo4I/7YmmTPEXDkJAyz38ofes01/img.png)
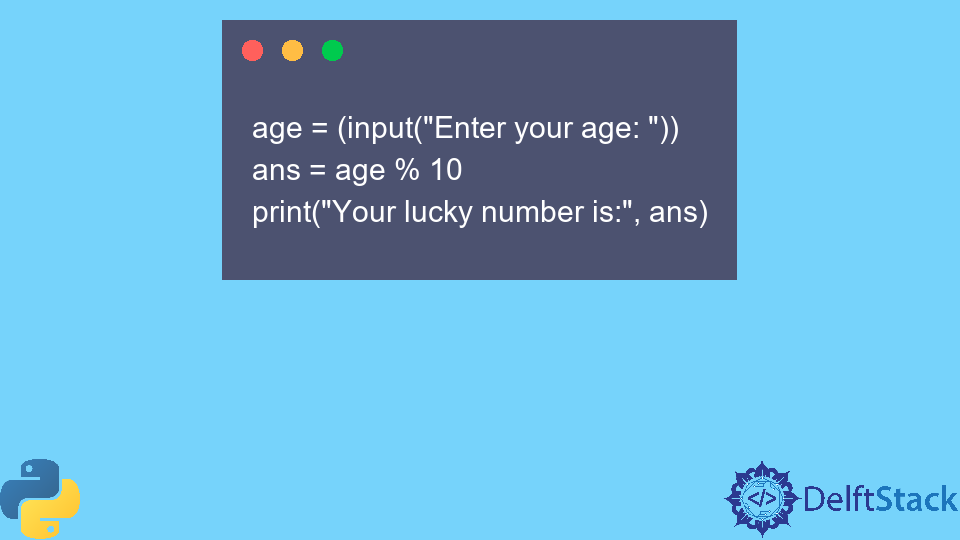
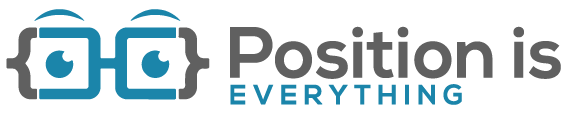
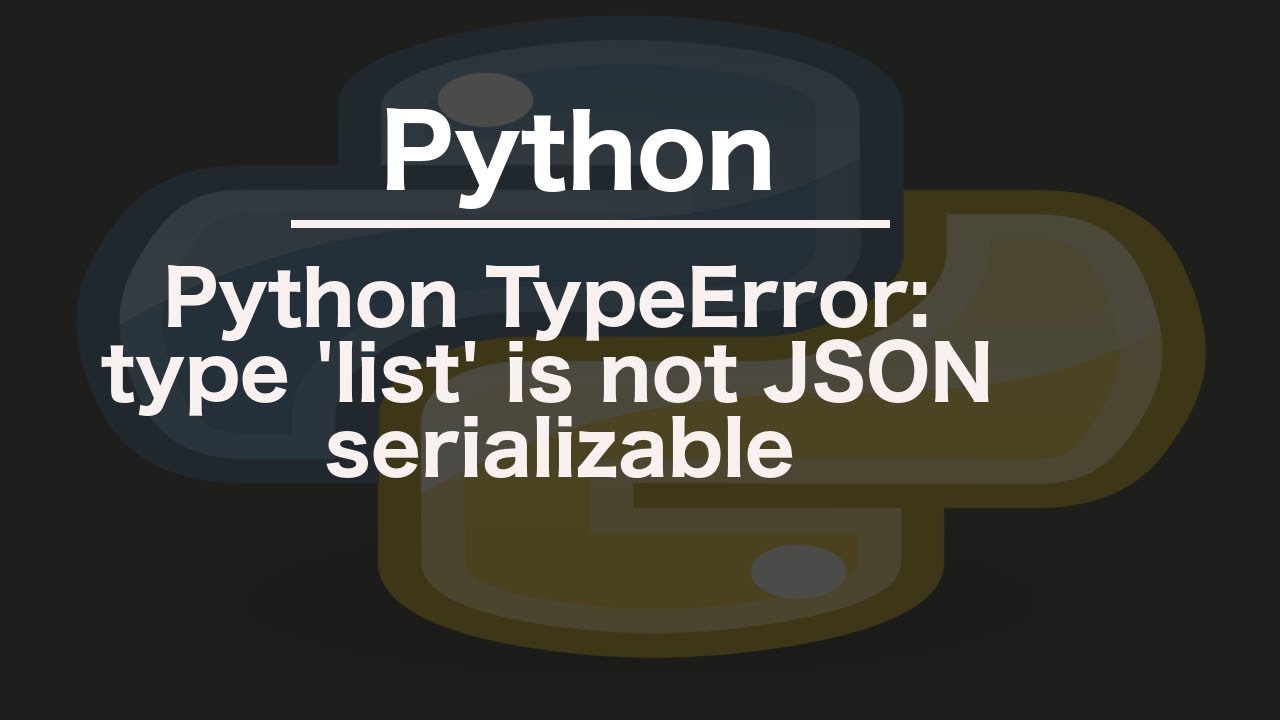
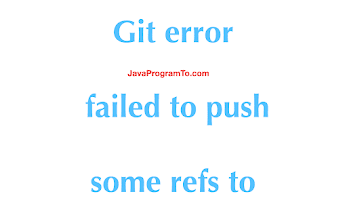




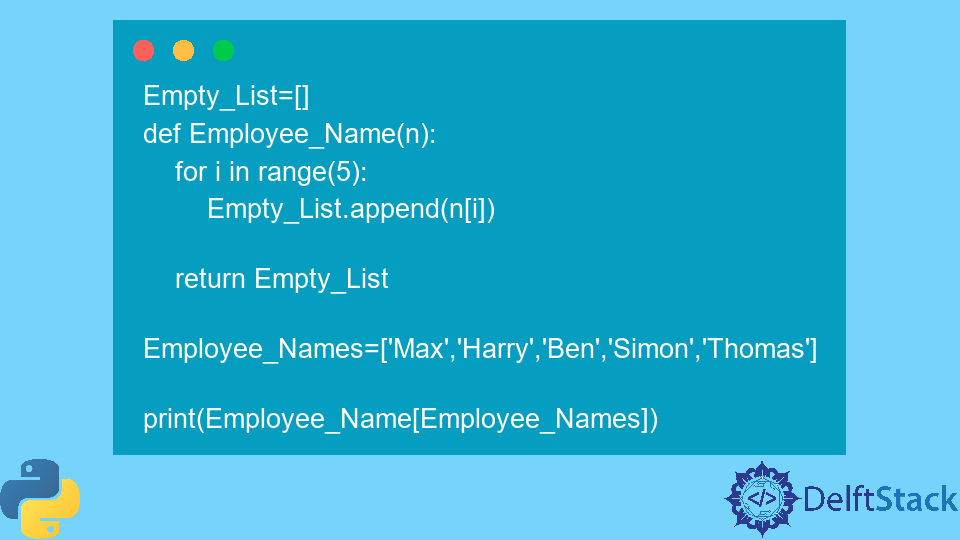

Article link: typeerror: object of type int64 is not json serializable.
Learn more about the topic typeerror: object of type int64 is not json serializable.
- TypeError: Object of type int64 is not JSON serializable
- Python – TypeError: Object of type ‘int64’ is not JSON serializable
- Fix Python TypeError: Object of type ‘int64’ is not JSON …
- How to Fix TypeError: Object of type ‘int64’ is not JSON …
- [Fixed] Object of Type int64 Is Not JSON Serializable
- Object of Type INT64 Is Not JSON Serializable: Debugged
- Typeerror: object of type int64 is not json serializable
- Fix the TypeError: Object of Type ‘Int64’ Is Not JSON Serializable
- [Solved] TypeError: Object of type int64 is not JSON serializable
- TypeError: Object of type \’int64\’ is not JSON serializable …
See more: nhanvietluanvan.com/luat-hoc