Trying To Access Array Offset On Value Of Type Bool
What is an array offset?
An array offset refers to the index or key of an element in an array. In PHP, arrays can be indexed using numerical indexes or associative keys. Array offsets are used to access and manipulate individual elements within an array. It allows programmers to retrieve values from arrays based on their positions or keys.
Understanding the concept of type bool
In PHP, bool is a data type that represents a boolean value, which can be either true or false. It is commonly used to store logical values and control program flow based on conditions. Bool values are often used in conditional statements and loops to make decisions or perform certain actions based on the evaluation of an expression.
The error: “trying to access array offset on value of type bool”
The error message “trying to access array offset on value of type bool” is triggered when a programmer tries to access an array element using a non-existent or incorrect index, and the value at that index is of type bool instead of an array. This error indicates that the programmer is attempting to index a non-array value, which is not allowed.
Common scenarios leading to this error
There are several common scenarios that can lead to this error:
1. Using an incorrect or non-existent index: If an incorrect or non-existent index is used to access an array element, the error will occur.
2. Overwriting an existing array with a bool value: If a programmer assigns a bool value to an existing array variable, it will result in the error when attempting to access elements using array offsets.
3. Incorrectly assigning a bool value to an array element: If a bool value is mistakenly assigned to an array element instead of an array, it can cause this error.
The potential causes of the error
The error “trying to access array offset on value of type bool” can be caused by several factors, including:
1. Programming mistakes: Incorrectly referencing array elements or assigning bool values to arrays can result in this error.
2. Inconsistent data structures: If the structure of the data is not properly maintained, it may lead to situations where array offsets are erroneously used on bool values.
3. Improper error handling: If error handling is not implemented correctly, it may lead to this error being displayed without clear identification of the root cause.
Strategies for preventing the error
To prevent the occurrence of the error “trying to access array offset on value of type bool,” programmers can follow these strategies:
1. Validate array structures: Before accessing array elements, make sure the array is properly initialized and populated. Check if the array is empty or has the expected structure to avoid potential errors.
2. Use conditional statements: Implement conditional checks to verify whether the accessed element is an array or bool value before attempting to use array offsets.
3. Debug and test code: Thoroughly test the code and use debugging techniques to identify any potential issues related to array offsets and bool values.
4. Update error handling: Improve error handling routines to provide more specific error messages when this error occurs, enabling easier identification and resolution.
How to debug and fix the error
When encountering the error “trying to access array offset on value of type bool,” follow these steps to debug and fix it:
1. Identify the source of the error: Determine the line of code where the error is being triggered.
2. Review the code logic: Check if there are any invalid array accesses or assignments to bool values that may have led to this error.
3. Validate array structures: Make sure the accessed array has the expected structure and that the requested offset exists.
4. Implement conditional checks: Use if statements or other conditional constructs to verify the type of value being accessed before using array offsets.
5. Debug the code: Step through the code using a debugger or use print statements to track the values of variables and identify any inconsistencies or unexpected behavior.
6. Update error handling: Enhance error handling to provide more informative error messages, helping to identify the root cause and facilitating resolution.
Best practices for working with array offsets and bool values
To ensure efficient and error-free coding when working with array offsets and bool values, consider the following best practices:
1. Properly initialize arrays: Initialize arrays with the correct structure and values before accessing or modifying their elements.
2. Use meaningful array keys: Assign meaningful and relevant keys to array elements to improve code readability and avoid potential confusion or errors.
3. Validate input data: Validate any external or user-provided input before using it as an array offset or value to prevent unexpected behavior.
4. Implement error handling: Develop robust error handling mechanisms to catch and handle errors related to array offsets and bool values effectively.
5. Write clear and explicit code: Clearly define the purpose and intent of your code, making it easier to identify and prevent potential issues with array offsets and bool values.
6. Adopt defensive programming: Anticipate potential errors and edge cases when working with arrays and bool values, and include appropriate error checking and handling mechanisms in your code.
Examples and case studies of the error in PHP code
To illustrate the occurrence of the error “trying to access array offset on value of type bool,” let’s consider a few examples:
Example 1:
“`
$data = true;
$value = $data[0];
“`
In this example, the variable `$data` is assigned a bool value. However, the programmer attempts to access its first element using the array offset `[0]`. Since `$data` is of type bool and not an array, the error “trying to access array offset on value of type bool” will occur.
Example 2:
“`
$users = array(
‘John’ => true,
‘Jane’ => false,
);
foreach ($users as $user) {
$name = $user[‘name’];
echo “Name: $name”;
}
“`
In this example, the programmer mistakenly assumes that `$user` is an array in the foreach loop. However, the variable `$user` represents the bool values `’true’` and `’false’` stored in the array. When the programmer attempts to access `$user[‘name’]`, the error “trying to access array offset on value of type bool” will be triggered.
In conclusion, the error “trying to access array offset on value of type bool” is a common mistake made by programmers when working with arrays in PHP. This error occurs when an attempt is made to access an array element using an incorrect or non-existent index, where the value at that index is a bool instead of an array. By understanding the concept of array offsets and the nature of type bool, following prevention strategies, debugging and fixing techniques, and adhering to best practices, programmers can effectively avoid and resolve this error in their PHP code.
Warning :- Trying To Access Array Offset On Value Of Type Null Php Error
What Does Trying To Access Array Offset On Value Of Type Bool Mean?
If you have ever encountered the error message “trying to access array offset on value of type bool” while working with PHP, don’t worry—you’re not alone. This error is encountered when you try to access an array using array offset syntax, but the value you are trying to access is of type bool (boolean).
To understand this error more clearly, let’s break down the various components involved:
Array: An array is a data structure in PHP that can hold multiple values at once. Each value in an array is associated with a specific index.
Array offset: Array offset refers to the index of an element within an array. In PHP, arrays are zero-indexed, meaning the first element has an index of 0, the second element has an index of 1, and so on.
Value of type bool: In PHP, bool (boolean) is a data type that represents two possible values: true and false.
When you try to access an array element using array offset syntax, PHP expects the value at the given offset to be an array or an object that can be treated as an array. However, if that value is of type bool instead, PHP throws the “trying to access array offset on value of type bool” error.
Now that we understand what the error message means, let’s explore some common causes and possible solutions for this issue.
Causes of the error:
1. Misuse of array assignment: One of the most common causes is mistakenly assigning a boolean value to an array offset. For example:
$array = true; // Incorrect assignment
In this case, $array is assigned a boolean value instead of an array. When you try to access any element using array offset syntax ($array[0]), PHP throws the error.
2. Incorrect return value from a function: If you are calling a function that is expected to return an array or an object, but it instead returns a boolean value, this error will occur.
function getArray() {
return true; // Incorrect return value
}
$array = getArray();
echo $array[0]; // Error!
Here, the getArray() function is incorrectly returning a boolean value instead of an array, resulting in the error.
3. Logic errors: This error can also occur due to logical mistakes in your code. For instance, if you accidentally set a boolean value to an array element, such as:
$array[0] = true; // Accidentally setting a boolean value
Later, when you try to access $array[0], the error will be triggered.
Solutions:
1. Double-check your assignments: Make sure you are assigning arrays to variables instead of boolean values. For example:
$array = [1, 2, 3]; // Correct assignment
2. Review function return values: Ensure that functions return the expected array or object, rather than a boolean value.
3. Debug your code: If the error persists, consider using debugging techniques to identify where the boolean value is being assigned or returned incorrectly. Tools like var_dump() or print_r() can help you inspect the values and types of variables.
FAQs:
Q1. Can this error be encountered in other programming languages?
A1. No, this specific error is unique to PHP. Other programming languages may have similar error messages related to type-mismatch or invalid array access, but the exact wording will differ.
Q2. How can I prevent this error from occurring?
A2. To prevent this error, ensure that you properly assign and manipulate arrays instead of boolean values. Double-check your code for any logical errors that may cause incorrect assignments or returns.
Q3. Can this error occur when accessing other data types?
A3. No, this error specifically occurs when trying to access an array offset on a boolean value. If you encounter similar errors with other data types, they will have different error messages.
Q4. Is there a way to handle this error gracefully?
A4. Yes, you can use error handling techniques, such as try-catch blocks, to catch and handle this error. By catching the error, you can display a custom error message or take appropriate actions based on the specific situation.
In conclusion, the error message “trying to access array offset on value of type bool” occurs when you attempt to access an array element using array offset syntax, but the value at that offset is of type bool (boolean). To resolve this error, ensure correct assignments of arrays, validate function return values, and debug any logic issues. By understanding the causes and solutions, you can successfully overcome this error and enhance the reliability of your PHP programs.
How To Solve Trying To Access Array Offset On Value Of Type Null?
When developing or working with PHP scripts, it is not uncommon to encounter various error messages or issues. One such error, which often leaves developers scratching their heads, is the “Trying to Access Array Offset on Value of Type Null” error. This error typically occurs when trying to access or retrieve a value from an array, but that particular value has a null value assigned to it. In this article, we will delve into this error, understand its causes, and discuss potential solutions to effectively resolve it.
Understanding the Error
To begin with, it is essential to understand what this error message is indicating. The error “Trying to Access Array Offset on Value of Type Null” specifically suggests that we are attempting to access an array offset, or a particular element within an array, that has a null value assigned to it. In programming terms, an array is a data structure used to store multiple values under a single variable name. Array offsets are the keys used to identify and access elements within the array.
Causes of the Error
There are a few likely causes that can result in this error message. Firstly, it could be due to the uninitialized or undefined nature of the array or the specific element being accessed. If the array has not been properly initialized or the element is not set, a null value may be assigned to it by default.
Another common cause is directly trying to access or retrieve a non-existent or non-numeric array offset. For instance, if the array has ten elements, trying to access element eleven will result in this error message. Similarly, using a non-integer or non-numeric offset will also lead to this error.
Solutions to Resolve the Error
1. Validate the Array and Array Offset:
First and foremost, it is vital to ensure that the array and the specific array offset being accessed exist and are properly initialized. Use conditional statements, such as “isset()” or “empty()”, to check if the array exists and if the specific offset is set before attempting to access it. This step helps avoid trying to access null values.
2. Use isset() Function:
In PHP, the isset() function checks if a variable or array has been set and is not null. Employing this function before accessing the array offset can prevent this error. A sample code snippet demonstrating its usage is as follows:
“`php
if(isset($array[$offset])) {
// Accessing array offset is safe here
}
“`
3. Validate Array and Array Offset Types:
It is crucial to ensure that the array and the array offset are of the expected types. Ensure that the array contains the elements expected, and the offset is a valid numeric or integer value. Performing type checks using functions like “is_array” or “is_numeric” beforehand can help avoid encountering this error.
4. Use the ternary operator or null coalescing operator:
Consider utilizing the ternary operator or null coalescing operator to handle null values gracefully. These operators allow us to provide default values or perform alternative actions when encountering null values in the array. Here’s an example using the null coalescing operator:
“`php
$element = $array[$offset] ?? ‘Default value’;
“`
Frequently Asked Questions (FAQs)
Q1. What is an array offset?
An array offset is a key used to identify and access elements within an array. Each element in the array has an associated offset which can be a numeric or string value.
Q2. Why am I encountering this error message?
The “Trying to Access Array Offset on Value of Type Null” error occurs when trying to access an array element that has a null value assigned to it. This can happen due to uninitialized arrays, non-existent offsets, or incorrect type usage.
Q3. How can I prevent this error in my code?
To prevent this error, ensure that the array and array offset are properly initialized and set. Implement proper validation checks using functions like isset(), empty(), or type checks beforehand. Additionally, consider using default values or alternative actions using the ternary or null coalescing operators.
Q4. Are there any PHP version-specific considerations for resolving this error?
No, the “Trying to Access Array Offset on Value of Type Null” error is not version-specific. However, it is always best practice to keep your PHP version updated to benefit from bug fixes and enhancements.
Keywords searched by users: trying to access array offset on value of type bool Trying to access array offset on value of type bool, Notice in libraries displayresults php 1229 trying to access array offset on value of type bool, warning: trying to access array offset on value of type bool php 8, Trying to access array offset on value of type bool magento 2, Trying to access array offset on value of type bool codeigniter, Trying to access array offset on value of type int in file, Trying to access array offset on value of type int yii2, Trying to access array offset on value of type int PHPExcel
Categories: Top 88 Trying To Access Array Offset On Value Of Type Bool
See more here: nhanvietluanvan.com
Trying To Access Array Offset On Value Of Type Bool
Introduction:
As developers, we often encounter various errors while working with programming languages. One common error message that frequently arises, particularly in PHP, is the infamous “Trying to access array offset on value of type bool” error. In this article, we will explore the causes, potential solutions, and best practices to help you effectively handle this error.
Understanding the Error:
The error message “Trying to access array offset on value of type bool” indicates that you are attempting to access an array offset on a value that is actually a boolean, rather than an array. This error is typically encountered in PHP, but the underlying concept is applicable to other languages as well.
Causes:
1. Incorrect Array Assignment: This error often occurs when a variable, designed to hold an array, is mistakenly assigned a boolean value instead. Consequently, when the array-indexing operation is attempted, it results in this error.
2. Misconfiguration of Conditional Statements: It is possible to encounter this error due to misconfigured conditional statements. If a condition only evaluates to either true or false and not an array, this error can be triggered.
3. Incorrect Database Interaction: In scenarios where database queries or API calls return boolean values instead of the expected array, attempting to access array offsets can result in this error.
Solutions:
1. Debugging Through Error Reporting: Enable error reporting in your development environment to receive detailed error messages. This will include the line numbers where the error originates, aiding debugging efforts. To enable error reporting, add the following lines to your code:
“`
error_reporting(E_ALL);
ini_set(‘display_errors’, 1);
“`
2. Validate Array Assignments: Double-check your code to ensure that you are assigning values of the correct type to array variables. Validate variables that should contain arrays explicitly using the `is_array()` function. If necessary, implement conditional checks to prevent assignments of boolean values.
3. Evaluate Conditional Statements: Verify that the condition being evaluated truly expects an array as its result. If the condition is expected to handle boolean results, refactor the code accordingly to handle each case separately.
4. Check Query Results: If the error occurs during database interactions, inspect the queries and ensure that they are returning the expected array results. If necessary, consult relevant API documentation to ensure proper usage and data handling.
5. Defensive Programming: Implement defensive programming techniques such as input validation and exception handling. Thoroughly validate and sanitize all inputs to avoid unexpected boolean returns instead of arrays.
FAQs:
Q1. Can this error occur in programming languages other than PHP?
Yes, although this article primarily focuses on PHP, the general concept of attempting to access array offsets on boolean values can be applicable to other programming languages as well.
Q2. How can I determine the specific line that triggers this error?
By enabling error reporting and displaying errors, you will receive detailed error messages, including the line number where the error occurs. Utilize those line numbers to pinpoint the origin of the error and facilitate troubleshooting.
Q3. I have already checked my code, but I still encounter the error. What should I do?
If you have carefully reviewed and validated your code but the error persists, consider seeking assistance from relevant communities, forums, or programming experts. Sharing your code and explaining the specific scenario will help others provide better insights into the problem.
Conclusion:
Understanding the “Trying to access array offset on value of type bool” error is essential for effective debugging and troubleshooting. By following the suggested solutions in this article, you can mitigate this error and improve the stability and reliability of your codebase. Remember to validate array assignments, evaluate conditional statements thoroughly, and double-check database interactions to prevent this error from impacting your applications.
Notice In Libraries Displayresults Php 1229 Trying To Access Array Offset On Value Of Type Bool
Libraries serve as a haven for knowledge seekers and avid readers, providing a wide range of resources in various formats. Nowadays, with the advancements in technology, libraries have evolved to offer digital services as well. However, just like any other technological system, these libraries encounter occasional issues that can disrupt the smooth functioning of their services. One such issue that may arise is the notice in libraries displayresults php 1229, trying to access an array offset on value of type bool.
In order to understand this notice and its implications, let us delve into the technical aspects associated with it. PHP, which stands for Hypertext Preprocessor, is a popular server-side scripting language widely used for web development. It is highly flexible and allows developers to create dynamic web pages and applications. PHP makes use of arrays, which are variables capable of storing multiple values at once.
In the case of libraries, PHP is often used to handle the display and retrieval of information from their databases. The notice in libraries displayresults php 1229 refers to a specific line of code, specifically line 1229 in the displayresults.php file. This notice indicates that an attempt is being made to access a specific index or offset within an array, but the value at that offset is of type boolean (bool) rather than an expected value such as an integer or string.
When programming in PHP, it is crucial to ensure that the values accessed within an array match the expected data type. Accessing a boolean instead of the expected data type can lead to unexpected behavior and potential errors. In the context of libraries, the notice in libraries displayresults php 1229 highlights that there may be a bug or error within the code responsible for displaying search results from the library’s database.
To resolve this issue, the underlying cause must be identified. Often, this notice occurs when there is an error in the database query or when the result set returned from the database is empty, resulting in a boolean value being returned instead of an array element. By examining line 1229 in the displayresults.php file, developers can trace the code responsible for retrieving and processing the search results.
Developers can apply error handling techniques in PHP to capture and handle such errors gracefully. For instance, by checking if the returned value is of type bool before accessing it as an array element, developers can prevent this notice from occurring. Additionally, implementing proper database query validation and error checking mechanisms can help identify and rectify any issues causing this notice.
Frequently Asked Questions (FAQs):
Q: How does the notice in libraries displayresults php 1229 impact library users?
A: The notice itself does not directly impact library users. However, if left unresolved, it may result in incomplete or erroneous search results being displayed, making it difficult for users to find the desired resources.
Q: Can library staff members address this issue?
A: Yes, library staff members can report this issue to the library’s technical team or the developers responsible for maintaining the library’s website or digital systems. It is advisable to provide them with the specific notice information, such as libraries displayresults php 1229, to expedite the resolution process.
Q: What can library users do if they encounter this notice while searching for resources?
A: If library users encounter this notice, they can try refreshing the page or conducting the search again after some time. If the problem persists, contacting the library’s help desk or technical support team can be helpful in resolving the issue.
Q: Are there any preventive measures libraries can take to avoid such notices in the future?
A: Libraries can proactively implement rigorous testing and quality assurance processes for their web applications to identify and resolve potential bugs or errors. Regular maintenance and updates to the library’s digital systems can also help minimize the occurrence of such notices.
Conclusion:
The notice in libraries displayresults php 1229, trying to access an array offset on value of type bool, is a technical issue that can disrupt the functionality of library websites and digital services. By understanding the cause and employing effective error handling techniques, libraries can resolve this issue and provide seamless access to their resources. With proactive measures in place, libraries can continue to offer a comprehensive and user-friendly experience to their patrons.
Warning: Trying To Access Array Offset On Value Of Type Bool Php 8
The release of PHP 8 brings a range of new features and improvements to the popular programming language. However, along with these enhancements, there are also certain changes that developers need to be aware of. One such change is the warning related to accessing array offsets on a value of type bool.
In earlier versions of PHP, when an array offset was accessed on a bool value, PHP would automatically convert the bool value to an array with the index 0, and return the corresponding value. This behavior allowed developers to easily access elements of an array, even if the initial value was a bool.
However, PHP 8 introduces a more strict type-checking mechanism, which avoids implicit type conversions. As a result, when attempting to access an array offset on a bool value, PHP 8 generates a warning, alerting the developer to a potential issue in the code.
This warning can be triggered in situations where an array is expected, but a bool value is provided. For example, consider the following code snippet:
“`
$flag = true;
echo $flag[0];
“`
In PHP 7 and earlier versions, this code would display the value at index 0 of the converted bool value (which is false). However, in PHP 8, it generates a warning:
“`
Warning: Trying to access array offset on value of type bool in
“`
This change promotes stricter coding practices and helps identify potential errors early on. It encourages developers to have clearer and more defined data types throughout their codebase, enhancing code reliability and reducing unexpected behavior.
Frequently Asked Questions (FAQs):
Q: How can I resolve the warning related to accessing array offsets on a bool value?
A: To resolve the warning, you need to ensure that the variable being accessed is an array, not a bool value. Before attempting to access an array offset, you should perform an appropriate check to ensure the variable is an array.
For example:
“`php
$flag = true;
if (is_array($flag)) {
echo $flag[0];
} else {
// Handle the situation where the variable is not an array
}
“`
By using the `is_array()` function, you can validate whether the variable is an array or not before accessing its offsets. This prevents the warning from being triggered and allows for appropriate handling of the situation.
Q: Why did PHP 8 change this behavior?
A: PHP 8 aims to improve the language’s consistency and avoid implicit type conversions. By generating warnings when accessing array offsets on bool values, PHP encourages developers to write more explicit and reliable code. This change fosters better understanding of the data types being used and helps prevent potential coding errors.
Q: What other changes should I be aware of in PHP 8?
A: PHP 8 brings several changes and improvements, including the introduction of JIT compilation, union types, named arguments, attributes, and more. It is important to thoroughly review the PHP 8 documentation and understand the full range of changes, so you can adapt your code accordingly.
Q: Can I disable the warning related to accessing array offsets on bool values?
A: Yes, you can suppress the warning by using the `@` error control operator. However, it is generally recommended to address the underlying issue instead of simply hiding the warning. By ensuring proper coding practices and avoiding accessing array offsets on non-array values, you can write more reliable and maintainable code.
Q: Are there other warnings related to array offsets in PHP 8?
A: Yes, PHP 8 introduces additional warnings related to array offsets, such as warnings when accessing string offsets on arrays and invalid array key types. These warnings help catch potential errors early on and promote proper usage of arrays and offsets.
In conclusion, the warning related to trying to access array offset on a value of type bool in PHP 8 emphasizes the need for stricter type-checking and increased code reliability. By addressing this warning and adopting appropriate coding practices, developers can benefit from a more consistent and error-free programming experience. Remember to review the PHP 8 documentation to understand all the changes and improve your code accordingly.
Images related to the topic trying to access array offset on value of type bool
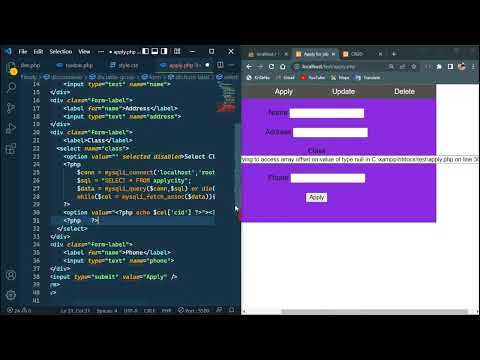
Found 26 images related to trying to access array offset on value of type bool theme




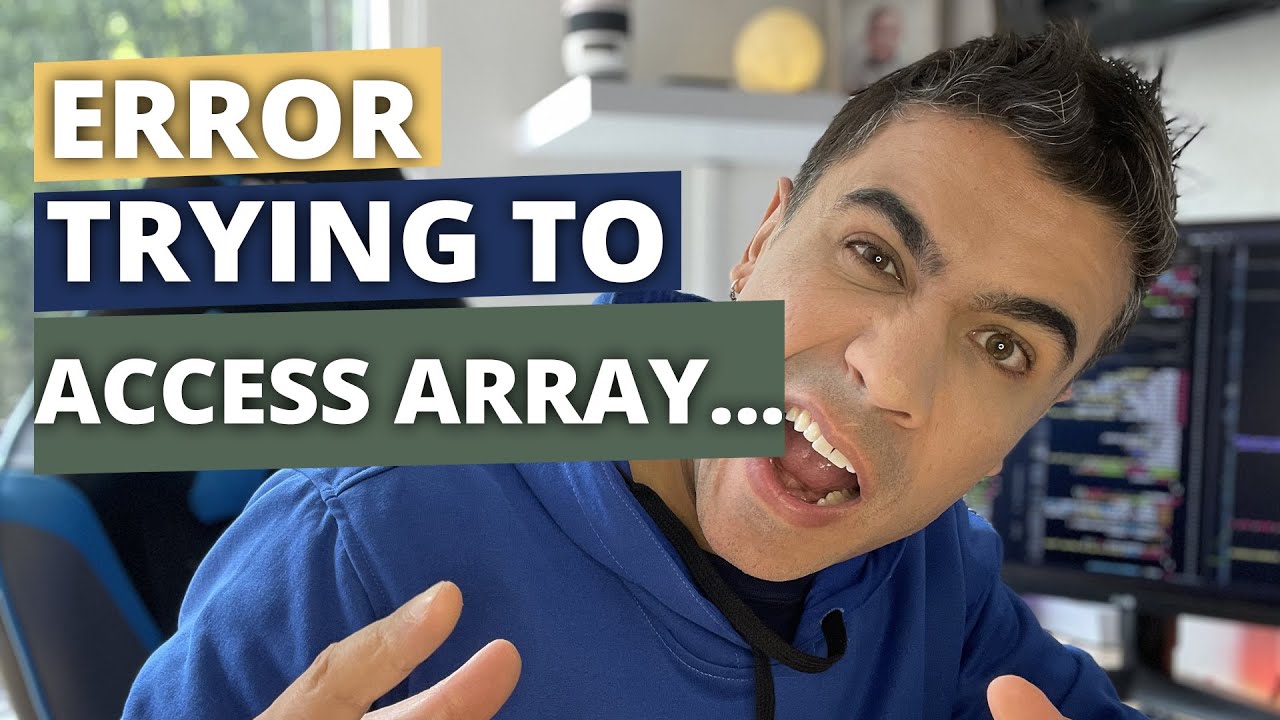

![Notice: Trying to access array offset on value of type null [#3191984] | Drupal.org Notice: Trying To Access Array Offset On Value Of Type Null [#3191984] | Drupal.Org](https://www.drupal.org/files/issues/2021-03-11/dependencyhelperbroken.png)
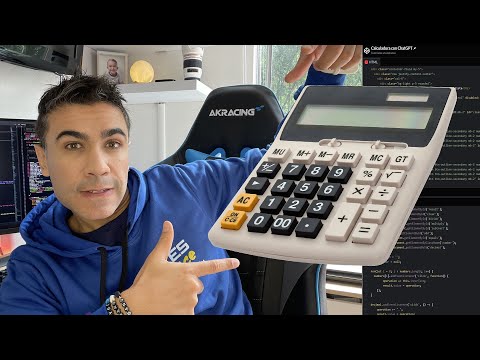
![Trying to access array offset on value of type bool in commerce_price_field_formatter_view() [#3175460] | Drupal.org Trying To Access Array Offset On Value Of Type Bool In Commerce_Price_Field_Formatter_View() [#3175460] | Drupal.Org](https://www.drupal.org/files/issues/2020-10-10/commerce_discount-n3175460-backtrace.png)

![PHP Notice: Trying to access array offset on value of type bool [#3212808] | Drupal.org Php Notice: Trying To Access Array Offset On Value Of Type Bool [#3212808] | Drupal.Org](https://www.drupal.org/files/issues/2022-08-23/screenshoot-test_1.png)

![PHP Notice: Trying to access array offset on value of type bool [#3212808] | Drupal.org Php Notice: Trying To Access Array Offset On Value Of Type Bool [#3212808] | Drupal.Org](https://www.drupal.org/files/issues/2022-09-06/after-patch_0.png)
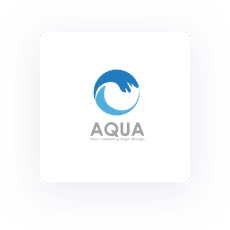

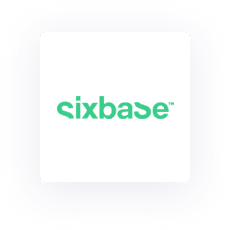
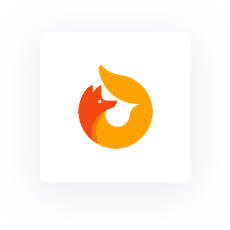
Article link: trying to access array offset on value of type bool.
Learn more about the topic trying to access array offset on value of type bool.
- Trying to access array offset on value of type bool in PHP 7.4
- Error Trying to access array offset on value of type bool in …
- Trying to access array offset on value of type bool : r/PHPhelp
- Message: Trying to access array offset on value of type null – W3docs
- Notice: Undefined Offset : 2 How to Solve? – php – Stack Overflow
- undefined offset PHP error – Stack Overflow
- Notice: Trying to access array offset on value of type bool
- Trying to access array offset on value of type bool | Upload file …
- PHP Warning: Trying to access array offset on value of type bool
- Switch to PHP 8 access array offset value error – Meta Box
- PHP 8 – Trying to access array offset on value of type bool
- Trying to access array offset on value of type bool in Elemntor …
- Trying to access array offset on value of type bool : r/PHPhelp
See more: nhanvietluanvan.com/luat-hoc