Python Datetime Today At Midnight
The datetime module in Python provides several powerful functionalities for handling dates and times. In this article, we will explore the datetime.today() method and learn how to work with dates and times, manipulate datetime objects, convert timezones, compare datetime objects, perform calculations, and handle different date formats.
Accessing the datetime module:
Before we dive into the datetime.today() method, let’s first understand how to access the datetime module in Python. The datetime module is part of the standard library, so you can simply import it using the import statement:
“`python
import datetime
“`
Understanding the datetime.today() method:
The datetime.today() method is used to obtain the current date and time. It returns a datetime object that represents the current local date and time. This method does not accept any arguments.
Getting the current date and time:
To get the current date and time, you can use the datetime.today() method as follows:
“`python
current_datetime = datetime.datetime.today()
print(current_datetime)
“`
This will output the current date and time in the format: “YYYY-MM-DD HH:MM:SS.ssssss”.
Working with the date object:
In many cases, we might only be interested in the date part of the datetime object. We can extract the date from datetime.today() using the .date() method and format it as a string.
“`python
current_date = datetime.datetime.today().date()
formatted_date = current_date.strftime(“%Y-%m-%d”)
print(formatted_date)
“`
This will output the current date in the format: “YYYY-MM-DD”.
Getting today’s date at midnight:
To obtain today’s date at midnight, we can use the replace() method to set the hour, minute, second, and microsecond to zero.
“`python
today_at_midnight = datetime.datetime.today().replace(hour=0, minute=0, second=0, microsecond=0)
print(today_at_midnight)
“`
This will output today’s date at midnight in the format: “YYYY-MM-DD 00:00:00”.
Manipulating the datetime object:
The datetime module allows us to easily perform arithmetic operations on datetime objects, such as adding or subtracting time intervals.
“`python
current_datetime = datetime.datetime.today()
one_hour_later = current_datetime + datetime.timedelta(hours=1)
one_day_ago = current_datetime – datetime.timedelta(days=1)
print(one_hour_later)
print(one_day_ago)
“`
This will output the datetime objects representing one hour later and one day ago respectively.
Converting the datetime object to a specific timezone:
To convert a datetime object to a specific timezone, we can use the pytz module. This module provides functionality for working with timezones.
“`python
import pytz
current_datetime = datetime.datetime.today()
new_timezone = pytz.timezone(“America/New_York”)
updated_datetime = current_datetime.astimezone(new_timezone)
print(updated_datetime)
“`
This will output the datetime object representing the current date and time in the “America/New_York” timezone.
Comparing datetime objects:
We can compare datetime objects to determine if one is greater than or less than another, or if they are equal.
“`python
datetime1 = datetime.datetime(2022, 1, 1)
datetime2 = datetime.datetime(2022, 2, 1)
if datetime1 > datetime2:
print(“datetime1 is greater than datetime2”)
elif datetime1 < datetime2:
print("datetime1 is less than datetime2")
else:
print("datetime1 and datetime2 are equal")
```
This will output "datetime1 is less than datetime2".
Performing calculations with datetime objects:
The datetime module allows us to calculate the time difference between two datetime objects and add or subtract a specific time interval.
```python
datetime1 = datetime.datetime(2022, 1, 1)
datetime2 = datetime.datetime(2022, 2, 1)
time_difference = datetime2 - datetime1
datetime3 = datetime1 + datetime.timedelta(days=7)
datetime4 = datetime2 - datetime.timedelta(hours=12)
print(time_difference)
print(datetime3)
print(datetime4)
```
This will output the time difference between datetime2 and datetime1, datetime1 + 7 days, and datetime2 - 12 hours respectively.
Handling different date formats:
The datetime module provides methods to work with specific date formats in Python. We can convert a string to a datetime object using the datetime.strptime() method.
```python
date_string = "2022-01-01"
date_object = datetime.datetime.strptime(date_string, "%Y-%m-%d")
print(date_object)
```
This will output the datetime object representing the date "2022-01-01".
In conclusion, the datetime module in Python offers a wide range of functionalities for working with dates and times. From retrieving the current date and time to manipulating datetime objects, converting timezones, comparing datetime objects, performing calculations, and handling different date formats, the datetime module has got you covered. So go ahead and explore the power of Python datetime!
FAQs:
Q: What does the datetime.today() method return?
A: The datetime.today() method returns a datetime object representing the current local date and time.
Q: How do I format the date object as a string?
A: You can use the strftime() method to format the date object as a string. For example, current_date.strftime("%Y-%m-%d") will output the date in the format "YYYY-MM-DD".
Q: How can I obtain today's date at midnight?
A: You can use the replace() method to set the hour, minute, second, and microsecond to zero. For example, datetime.datetime.today().replace(hour=0, minute=0, second=0, microsecond=0) will give you today's date at midnight.
Q: How can I add or subtract time intervals from a datetime object?
A: You can use the timedelta class from the datetime module. For example, datetime.datetime.today() + datetime.timedelta(hours=1) will give you the datetime object representing one hour later.
Q: How can I compare datetime objects?
A: You can use the greater than (>) and less than (<) operators to check if one datetime object is greater than or less than another. You can also use the equality (==) operator to determine if two datetime objects are equal.
Q: How can I convert a datetime object to a specific timezone?
A: You can use the astimezone() method in combination with the pytz module. For example, current_datetime.astimezone(pytz.timezone("America/New_York")) will convert the datetime object to the "America/New_York" timezone.
Q: How can I convert a string to a datetime object?
A: You can use the datetime.strptime() method to convert a string to a datetime object. For example, datetime.datetime.strptime("2022-01-01", "%Y-%m-%d") will give you the datetime object representing the date "2022-01-01".
Ultimate Guide To Datetime! Python Date And Time Objects For Beginners
How To Get Today Date At Midnight In Python?
Python, being a versatile and powerful programming language, offers several methods to work with dates and times. If you ever find yourself in a situation where you need to obtain the current date at midnight, Python provides a straightforward solution. In this article, we will explore various approaches to accomplish this task and discuss their advantages and limitations. Let’s dive in!
Method 1: Using the datetime module
To obtain today’s date at midnight, we can utilize the `datetime` module in Python. The `datetime` module provides classes to work with dates and times efficiently. Here’s an example code snippet that demonstrates how to achieve the desired result:
“`python
from datetime import datetime
today = datetime.now().replace(hour=0, minute=0, second=0, microsecond=0)
“`
In this code snippet, we use the `datetime.now()` function to get the current date and time. We then use the `replace()` method to set the hour, minute, second, and microsecond values to zero, effectively obtaining the date at midnight.
Method 2: Using the date module
Python also offers the `date` module, which is a lightweight alternative to the `datetime` module if you only need to work with dates. Here’s an example code snippet that shows how to obtain today’s date at midnight using the `date` module:
“`python
from datetime import date
today = date.today()
“`
In this code snippet, we import the `date` class from the `datetime` module and utilize the `today()` method to retrieve the current date. Since the `date` class does not have time-related attributes, we automatically obtain the date at midnight.
FAQs:
Q1. Why would I need to get today’s date at midnight in Python?
A1. There are several scenarios where obtaining today’s date at midnight can be useful. For example, if you need to compare dates without considering the time component, or if you are working with time-sensitive data and want to align it to a specific reference point.
Q2. Can I get the current date at midnight in a specific time zone?
A2. Yes, you can modify the code snippets mentioned earlier to account for time zone differences. You can leverage the `pytz` library in Python, which provides support for various time zones. By using `pytz`, you can adjust the obtained date at midnight according to the desired time zone.
Q3. How can I format the obtained date at midnight?
A3. Python offers the `strftime()` method to format date and time objects. You can apply this method to the obtained date at midnight to format it as per your requirements. For example, you can format it as a string following the ISO 8601 standard (‘YYYY-MM-DD’) or any other custom format.
Q4. Is it possible to obtain the date at midnight for any historical date?
A4. Yes, Python allows you to manipulate historical dates as well. With appropriate modification to the code snippets mentioned earlier, you can obtain the date at midnight for any specific date in history.
Q5. Are there any performance considerations when obtaining the date at midnight?
A5. The approaches we discussed are efficient and have negligible performance impact. However, if you require to repeatedly obtain the date at midnight within a time-sensitive application, you can store the result in a variable to avoid repetitive calculations.
In conclusion, obtaining today’s date at midnight in Python is a straightforward task thanks to the functionalities provided by the `datetime` and `date` modules. By following the approaches covered in this article, you can effortlessly retrieve the desired result. Additionally, addressing the frequently asked questions clarifies potential doubts and helps you make the most out of Python’s date and time capabilities.
What Is The Utc Midnight Timestamp?
The UTC midnight timestamp, also known as the Coordinated Universal Time (UTC) midnight timestamp, is a standardized measure of time that represents the precise moment when the clock strikes midnight in the UTC time zone. UTC is the primary time standard used across the globe, providing a universal reference for timekeeping and coordination of activities worldwide.
Understanding UTC:
To comprehend the concept of the UTC midnight timestamp, it is essential to grasp the fundamentals of UTC itself. Coordinated Universal Time is a high-precision atomic time scale based on International Atomic Time (TAI) with leap seconds added at irregular intervals to compensate for the Earth’s slowing rotation. It is regulated and maintained by the International Bureau of Weights and Measures (BIPM).
UTC is critical for various global applications, including telecommunications, satellite navigation systems, international aviation, and financial transactions. It ensures consistency and synchronization in these industries, allowing for effective coordination across different time zones.
Determining the Midnight Timestamp:
The midnight timestamp is calculated based on the UTC time zone’s definition of midnight, which refers to the start of a new day. However, it is important to note that in some regions, midnight may not coincide exactly with the beginning of a new calendar day, especially when considering Daylight Saving Time adjustments and variations in local timekeeping conventions.
The UTC midnight timestamp is recorded as the precise moment when the time digits change from one day to the next. This timestamp typically follows the 24-hour clock format, ranging from 00:00:00 to 23:59:59.999. However, it is important to remember that while the midnight timestamp marks the transition to a new day, it does not necessarily reflect the beginning of daily activities or events worldwide.
Applications and Significance:
The UTC midnight timestamp is widely used in various sectors where accurate time synchronization is crucial. Here are a few notable applications:
1. Financial Transactions: In international finance, precise timing is essential for conducting transactions, calculating interest rates, and managing risks. The UTC midnight timestamp allows global financial institutions to accurately record and reconcile transactions across different time zones, ensuring transparency and consistency in financial operations.
2. Global Communication: Telecommunications networks, internet protocols, and messaging systems often rely on timestamps for organizing and delivering information. The UTC midnight timestamp provides a standardized reference point, ensuring effective communication and data coordination across different regions of the world.
3. Satellite Operations: Satellites orbiting the Earth require precise time synchronization to facilitate navigation, data transmission, and coordination with ground stations. The UTC midnight timestamp assists in maintaining accurate timekeeping on satellites, enabling seamless communication and efficient satellite operations.
4. Scientific Research: In scientific experiments and data analysis, timestamping is vital for correlating events accurately. The UTC midnight timestamp aids researchers in aligning measurements, synchronizing data from multiple sources, and conducting experiments with precision.
Frequently Asked Questions:
1. Does the UTC midnight timestamp adjust for Daylight Saving Time changes?
No, the UTC midnight timestamp does not specifically account for Daylight Saving Time adjustments. UTC is a standardized timekeeping system that does not incorporate regional variations such as Daylight Saving Time. The adjustment for Daylight Saving Time is carried out at the local level and may influence the local midnight time but does not affect the UTC midnight timestamp.
2. How is the UTC midnight timestamp different from local midnight?
The UTC midnight timestamp is based on Coordinated Universal Time, which is a globally recognized time standard. On the other hand, local midnight refers to the moment when the clock strikes twelve in a specific time zone or geographical region. While the UTC midnight timestamp provides a universal reference for time synchronization, local midnight varies according to the time zone and may be subject to local conventions and adjustments like Daylight Saving Time.
3. Can the UTC midnight timestamp be used as a reference for local activities?
Yes, the UTC midnight timestamp can be used as a global reference for timekeeping and coordination of activities. However, since it primarily reflects the change in date rather than the beginning of daily activities, it may not align with local customs or practicalities. Local standards and conventions should be taken into account when considering specific events or local activities.
In conclusion, the UTC midnight timestamp plays a vital role in achieving global time consistency and synchronization across various sectors. Its use as a universal reference point facilitates coordination, communication, and accurate timekeeping worldwide. By understanding this timestamp and its significance, businesses, industries, and scientific communities can ensure seamless operations and effective interconnection in the globalized world.
Keywords searched by users: python datetime today at midnight python datetime 00:00:00, python datetime combine, python datetime start of day, python datetime tomorrow midnight, python convert date to datetime, datetime midnight, Date to datetime python, python datetime format
Categories: Top 67 Python Datetime Today At Midnight
See more here: nhanvietluanvan.com
Python Datetime 00:00:00
Introduction:
In Python programming, the datetime module provides various classes and functions to handle dates and times. One commonly used aspect of datetime is the representation of time as 00:00:00, which signifies midnight or the start of a new day. In this article, we will delve into the details of working with the Python datetime 00:00:00 timestamp and explore its various applications.
Understanding the Python datetime 00:00:00 Timestamp:
The datetime module in Python allows us to work with time values down to microseconds. When representing a specific time, the time value can include hours, minutes, seconds, and microseconds. In the case of 00:00:00, it represents the beginning of the day, i.e., midnight.
The datetime module provides the datetime.time class, which enables the creation of time objects. By default, when creating a time object, if no specific hour, minute, or second values are provided, the time is set to 00:00:00. Here’s an example:
“`python
import datetime
midnight = datetime.time()
print(midnight) # Output: 00:00:00
“`
In the above code snippet, the `datetime.time()` function initializes a time object with a default value of 00:00:00.
Applications of the Python datetime 00:00:00 Timestamp:
1. Date Manipulation:
One common use case for the 00:00:00 timestamp is in manipulating dates. Sometimes, when working with dates representing an entire day, it is necessary to compare or perform operations on specific portions of the day. For example, checking if a date falls within a specific range of hours, minutes, or seconds.
2. Time Difference Calculation:
The 00:00:00 timestamp is handy when calculating the time difference between two DateTime objects. By representing both times as 00:00:00, the difference can be easily calculated without considering the time components. This simplifies the process and provides a cleaner result.
“`python
import datetime
start = datetime.datetime(2022, 1, 1)
end = datetime.datetime(2022, 1, 2)
time_difference = end – start
print(time_difference) # Output: 1 day, 0:00:00
“`
In the above example, both the start and end times are initialized with 00:00:00, allowing a straightforward calculation of the time difference.
3. Scheduling Events:
The 00:00:00 timestamp is commonly used in event scheduling and management systems. By representing the start time of an event as 00:00:00, it ensures that the event is scheduled at the beginning of the day. This simplifies the process of handling and managing events efficiently.
Frequently Asked Questions (FAQs):
Q1. Can I specify a different time other than 00:00:00 using datetime.time()?
A1. Yes, you can specify a different time by passing the hour, minute, and second values to the `datetime.time()` function. For example, `datetime.time(10, 30, 45)` will create a time object representing 10:30:45.
Q2. How can I extract the current date and time from the 00:00:00 timestamp?
A2. To extract the current date and time, you can use the `datetime.datetime.now()` function, which returns a DateTime object representing the current date and time. By default, it includes the current hour, minute, and second values.
Q3. Can I compare DateTime objects with different time values to 00:00:00?
A3. Yes, you can easily compare DateTime objects with different time values to 00:00:00. The comparison will be based on the date component, ignoring the time portion. This allows you to handle date-based operations without considering the specific time.
Q4. How can I convert a string representation of time to the 00:00:00 format?
A4. You can use the `strptime` function from the `datetime` module to parse a string representation of time and convert it to a DateTime object. Once you have the DateTime object, you can extract the time portion and set it to 00:00:00.
Conclusion:
The Python datetime 00:00:00 timestamp serves as a critical component in handling time-related operations in Python programming. By representing the start of the day, it simplifies date manipulation, time difference calculations, and event scheduling. Understanding the applications and potential of this timestamp empowers developers to design efficient and accurate time-related functionalities in their Python projects.
Python Datetime Combine
Python datetime module provides comprehensive tools for working with dates and times. The combine function specifically combines the information from two datetime objects into a new datetime object, creating a unified representation of both date and time. This is particularly useful when you have separate date and time information and need them to be merged into a single unit.
The syntax of the datetime combine function is as follows:
“`python
datetime.combine(date, time)
“`
where `date` is a datetime.date object representing the date to be combined, and `time` is a datetime.time object representing the time to be combined.
It’s important to note that the arguments passed to the combine function must be compatible datetime objects. For example, if you try to combine a date object with a timedelta object, it will raise a TypeError since they are not of the same type.
Let’s now look at some examples to understand the usage of datetime combine in more detail:
Example 1:
“`python
import datetime
date_obj = datetime.date(2022, 1, 1)
time_obj = datetime.time(12, 30)
combined_datetime = datetime.datetime.combine(date_obj, time_obj)
print(combined_datetime)
“`
Output:
“`
2022-01-01 12:30:00
“`
In this example, we create separate date and time objects using the datetime.date and datetime.time constructors. We then combine these objects using the datetime.combine function, resulting in a new datetime object that represents the combined date and time values.
Example 2:
“`python
import datetime
today = datetime.date.today()
current_time = datetime.datetime.now().time()
combined_datetime = datetime.datetime.combine(today, current_time)
print(combined_datetime)
“`
Output:
“`
2022-11-15 18:45:23.746428
“`
In this example, we use the date.today() method to obtain the current date, and the datetime.now().time() method to obtain the current time. We then combine these values using datetime.combine, resulting in a new datetime object that represents the current date and time.
Combining date and time values is particularly useful when dealing with time-sensitive operations, such as event scheduling, data logging, or timestamping. The ability to seamlessly merge date and time information provides a unified representation that can be easily utilized in various computations and calculations.
FAQs about Python datetime combine:
Q: Can I combine date and time values that are stored as strings?
A: No, the combine function requires datetime objects as arguments. If your date and time information is in string format, you would need to parse them into datetime objects using the datetime.strptime function before using the combine function.
Q: Can I combine only the time values and keep the date intact?
A: Yes, you can combine time objects without changing the date. Simply create a date object with the desired date, and then combine it with the time object.
Q: What happens if I try to combine incompatible datetime objects?
A: If you try to combine objects that are not of the same type, such as a date object with a timedelta object, a TypeError will be raised. Make sure the objects you pass to the combine function are compatible.
Q: Can I combine multiple datetime objects into one?
A: The combine function only allows you to combine two datetime objects at a time. If you need to combine multiple datetime objects, you can use a loop or other iterative methods to combine them one by one.
In conclusion, the datetime combine function in Python provides a powerful tool for combining date and time values from separate datetime objects. It allows you to create a new datetime object that represents the unified date and time information. By understanding the syntax, parameters, and examples provided in this article, you can effectively utilize this function in your Python programs and perform various time-related operations effortlessly.
Images related to the topic python datetime today at midnight
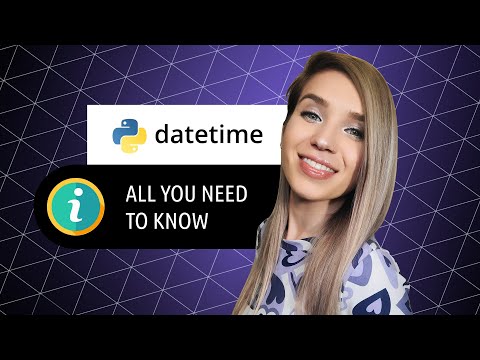
Found 43 images related to python datetime today at midnight theme

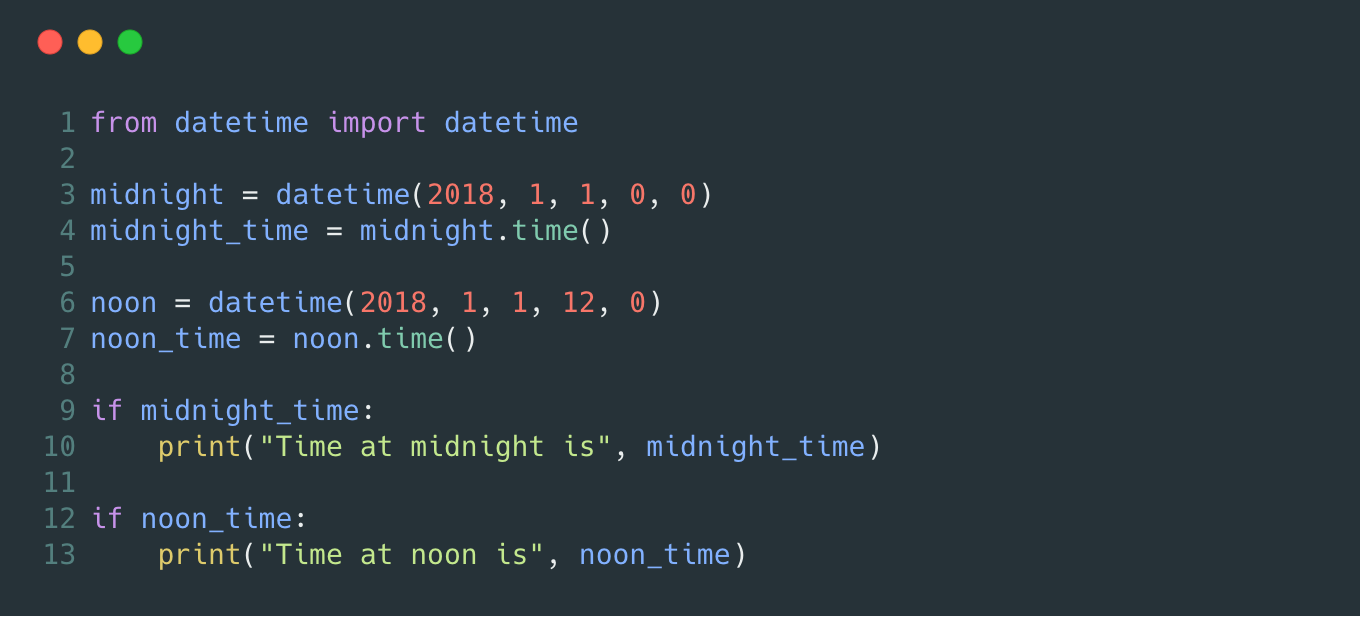

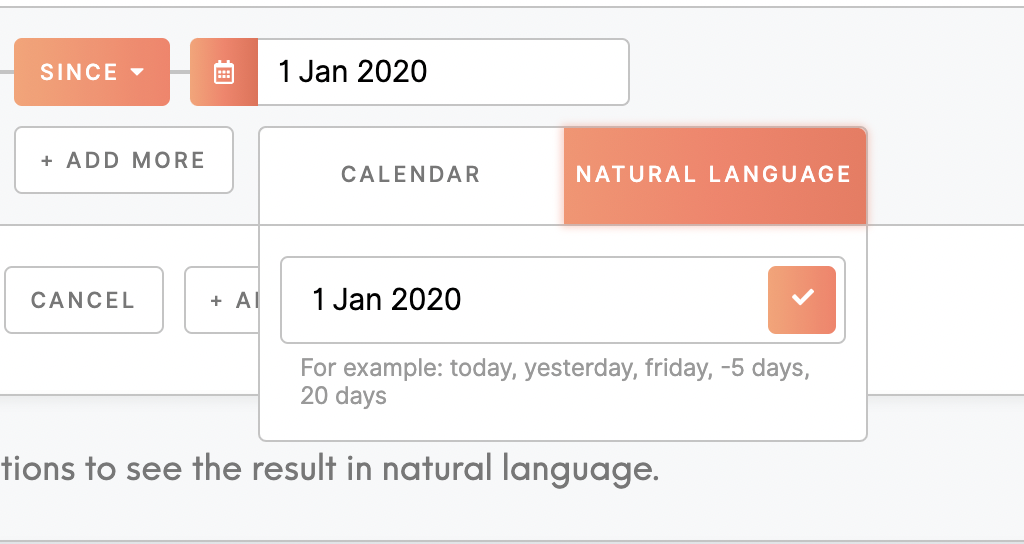
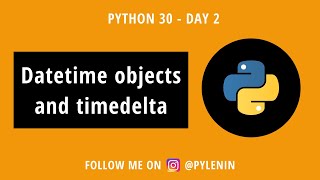
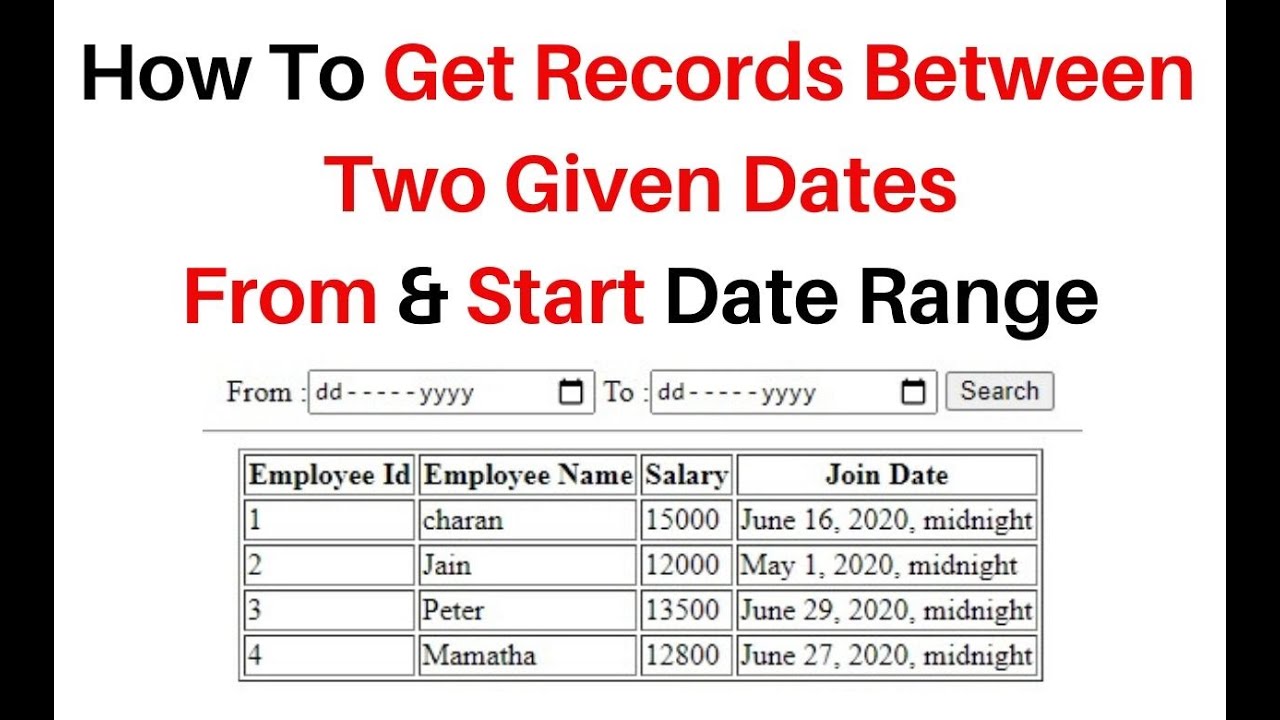
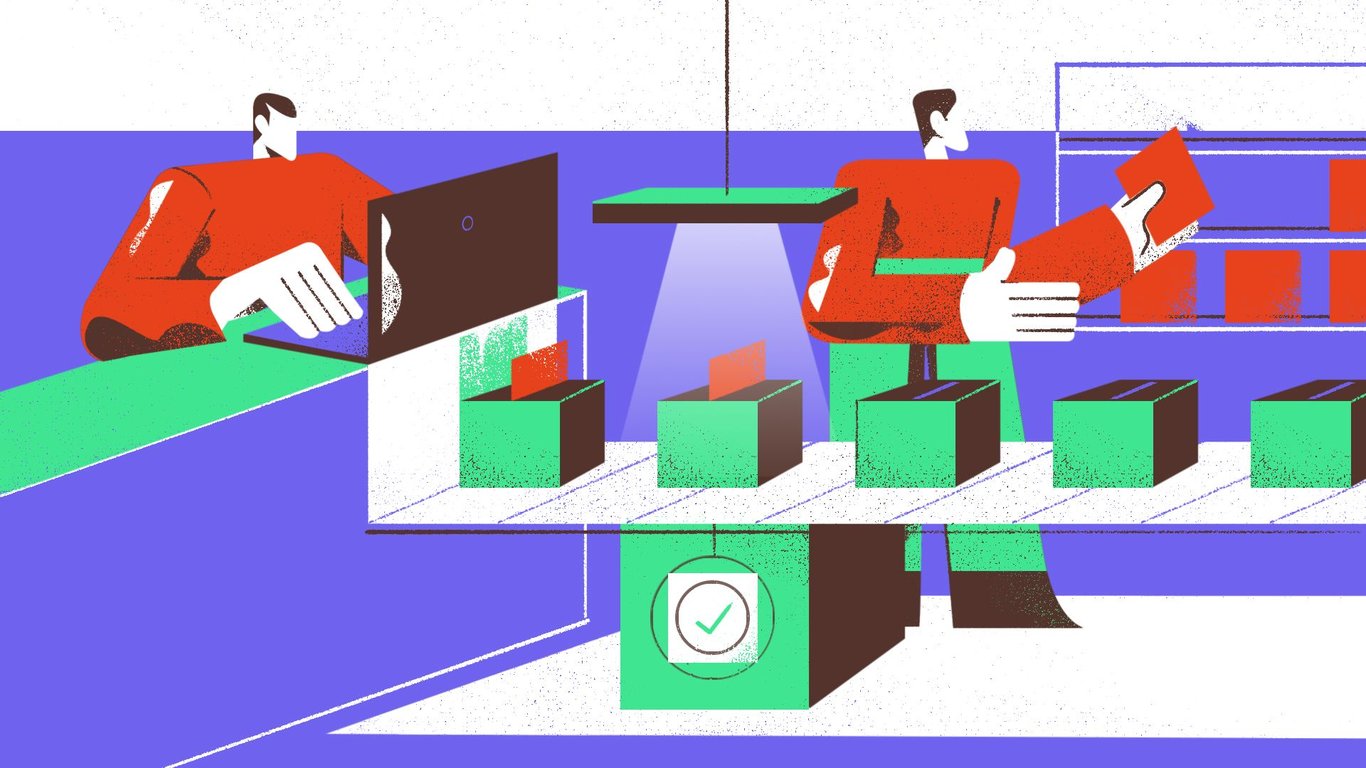
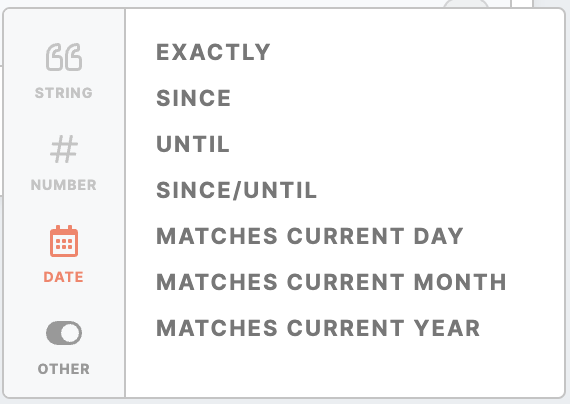

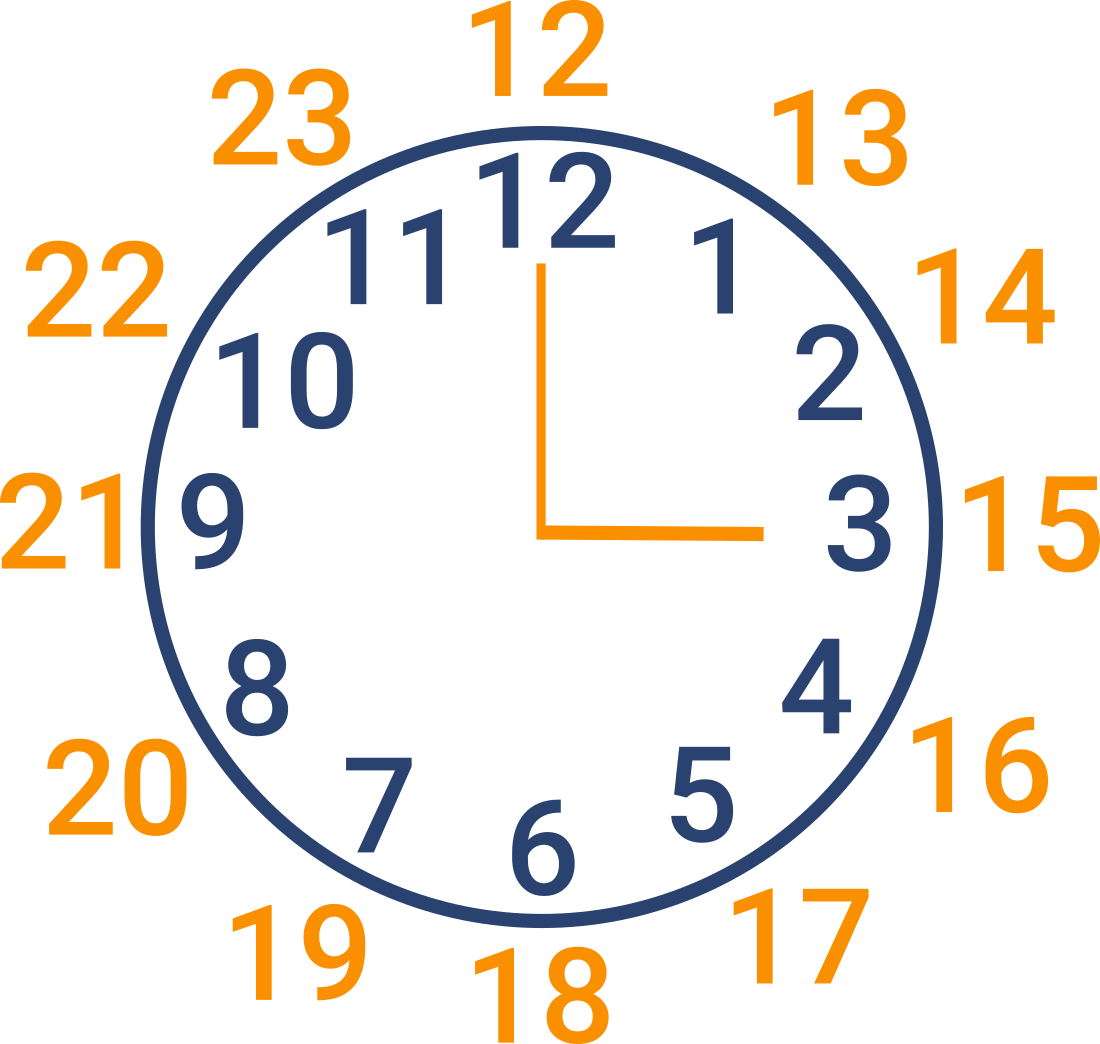
Article link: python datetime today at midnight.
Learn more about the topic python datetime today at midnight.
- Convert the date to datetime (midnight of the date) in Python
- How do I get the UTC time of “midnight” for a given timezone?
- Get Midnight Today | python blog – I Spy Code
- Write a Python program to convert the date to datetime (midnight of the …
- Unix time – Wikipedia
- How to Get Current Date and Time in Python – PYnative
- create date in python without time – Stack Overflow
- Python 3 – timestamp from today morning 00:00:00 – Treehouse
- Python Check datetime With No/Zero/Midnight Time
- Python Pandas – Convert times to midnight in DateTimeIndex
- What was midnight yesterday as an epoch time? – DevPress
- How to Get and Use the Current Time in Python
- time range midnight problem – Python-forum.io
- Work with dates and times in Python using datetime
See more: nhanvietluanvan.com/luat-hoc