Cannot Convert Null Literal To Non-Nullable Reference Type
In programming, a null literal represents the absence of a value. It is often used to indicate that a variable or object does not refer to anything. On the other hand, non-nullable reference types are types in C# that cannot have a null value assigned to them. These types guarantee that they will always contain a valid reference to an object.
Understanding the Error Message: “Cannot Convert Null Literal to Non-Nullable Reference Type”
When you encounter the error message “Cannot convert null literal to non-nullable reference type,” it means that you are attempting to assign a null value to a variable or object that is defined as non-nullable. This error occurs because the compiler ensures that non-nullable reference types cannot contain null values, and it detects this violation during compilation.
Causes of the Error: Attempting to Assign Null to a Non-Nullable Reference Type
The error can occur in various scenarios. It may arise when you directly assign a null literal to a variable or parameter that has a non-nullable reference type. For example, if you have a variable of type `string` declared as non-nullable, assigning null to it will trigger the error.
Another common cause is when you perform operations that involve a non-nullable reference type and a nullable reference type, without proper handling of potential null values. In such cases, the compiler cannot guarantee that a null value will not be encountered, resulting in the error.
Nullable Reference Types: A Brief Introduction
Introduced in C# 8.0, nullable reference types enhance nullability tracking in the language. By enabling this feature, you can annotate your reference types to indicate whether they allow null values or not. This feature helps catch potential null reference errors at compile-time, reducing the chances of null-related run-time exceptions.
Avoiding the Error: Using Nullable Reference Types Correctly
To avoid the “Cannot convert null literal to non-nullable reference type” error, it is crucial to correctly annotate your reference types, explicitly indicating whether they allow null or not. By marking a reference type as nullable, the compiler understands that it may contain null values and allows assignments accordingly. Conversely, marking it as non-nullable ensures that you cannot assign null to it.
Utilizing the Null-Forgiving Operator to Avoid the Error
In some cases, you may encounter situations where you need to suppress the nullability warnings even though the variable is marked as non-nullable. The null-forgiving operator (`!`) comes in handy in such scenarios. By using this operator, you essentially tell the compiler that you have manually verified the null safety of the code, overriding the warnings.
Working with Null-Conditional Operators to Handle Nullable Reference Types
When dealing with nullable reference types, it is crucial to handle potential null values appropriately. The null-conditional operators (`?.`, `??`, and `?.[]`) provide concise ways to perform null checks and handle null values. These operators help prevent null-related errors by conditionally executing code only if the reference is not null.
Best Practices for Handling Non-Nullable Reference Types and Null Values
To handle non-nullable reference types and null values effectively, consider the following best practices:
1. Always enable nullable reference types in your projects and diligently annotate your types to reflect their correct nullability.
2. Avoid directly assigning null literals to non-nullable reference types. Instead, use nullable reference types or null-forgiving operators if necessary.
3. Handle nullable reference types with null-conditional operators to correctly handle potential null values.
4. Use proper null checks and defensive coding techniques to ensure that your code does not encounter null reference exceptions during runtime.
5. Regularly review and update your codebase to reflect changes in nullability annotations as your project evolves.
Conclusion
The error “Cannot convert null literal to non-nullable reference type” indicates that you are trying to assign null to a variable or object that is defined as non-nullable. By understanding the causes of this error and using nullable reference types correctly, you can write safer, more robust code that minimizes the chances of null-related errors. Adhering to best practices and staying mindful of how you handle null values will contribute to the overall quality and reliability of your codebase.
FAQs:
Q: How can I convert a null literal to a non-nullable reference type?
A: You cannot directly convert a null literal to a non-nullable reference type. The whole idea behind non-nullable reference types is to ensure that they cannot contain null values. If you need to assign null to a variable, consider using a nullable reference type instead.
Q: Can I turn off nullable reference types in C#?
A: You can disable nullable reference types at the project level by adding the `
Q: What does the error “expression is always false according to nullable reference types annotations” mean?
A: This error typically occurs when the compiler detects a condition that can never be true based on the nullable reference types annotations. It signifies a logical discrepancy in your code. Review the code where the error occurs and ensure that the condition is correctly evaluated.
Q: Can I have a non-nullable list in C#?
A: No, you cannot have a non-nullable list in C#. Lists inherently allow the addition of null elements. If you need to ensure that a list does not contain null values, you can perform null checks or filter out null values programmatically.
Q: What should I do if I encounter the error “possible null assignment to non-nullable entity”?
A: This error indicates that the compiler is unable to guarantee that a variable will always have a non-null value. Review the code where the error occurs and ensure that all code paths assign a non-null value. If necessary, consider using nullable reference types or handle potential null values with null-conditional operators.
Cs8600: Converting Null Literal Or Possible Null Value To Non-Nullable Type. C#
Can We Assign Null To Reference Type?
In the world of programming, assigning null to a variable is a common practice. Null represents the absence of an object and is primarily used with reference types. However, there is some confusion regarding whether it is valid to assign null to reference types. In this article, we will delve into this topic, exploring what reference types are, discussing the concept of null, and answering frequently asked questions to provide a comprehensive understanding.
Understanding Reference Types:
Before diving into the question of assigning null, it is crucial to understand what reference types are. In programming languages like Java, C#, and Python, reference types are used to create objects. Unlike value types (e.g., int, float, bool), which hold their actual values, reference types store references to objects in memory.
In simpler terms, when we declare a reference type variable, we can think of it as creating a box capable of holding an object. The variable only stores a memory address pointing to the actual object in memory. This differs from value types, which store the actual value in the variable itself.
What is null?
The concept of null comes into play when we want to signify that a reference type variable does not point to any object in memory. Null is a special value assigned to reference types that indicates the absence of an actual object. It serves as a placeholder, representing a variable that is not currently referencing any data.
Assigning Null to Reference Types:
Now that we understand the basics, let’s address the main question: Can we assign null to reference types? The answer is a resounding yes. Null can be assigned to any reference type variable because it indicates the absence of an object or the lack of a valid value.
When assigning null to a reference type, we are effectively stating that the variable does not point to anything valid in memory. This can be useful in many scenarios, such as when declaring a variable that will later be assigned an object, but currently has no specific value.
For example, let’s consider a variable named “person” of type “Person” defined in a programming language like C#. By assigning null to this variable, we are indicating that it does not currently reference any instance of the “Person” class. Later on, we can assign an actual person object to make it reference a specific instance.
Is Assigning Null to Reference Types Safe?
Now you might wonder if assigning null can have any adverse effects on your program. In most cases, assigning null is completely safe and considered a standard practice. However, it is essential to handle null references appropriately to prevent null-reference exceptions.
Careless handling of null values can lead to runtime errors when attempting to access properties or methods on a null reference. Therefore, it is essential to perform null checks before accessing any member of a reference type variable, using conditional statements like ‘if (variable != null)’ to ensure no null-reference exceptions occur.
Using Null with Structures:
While null is primarily associated with reference types, it is important to note that it cannot be assigned to value types or structures. Value types, unlike reference types, store their actual values, and there is no concept of null for them. If you attempt to assign null to a value type variable, you will encounter a compilation error.
FAQs:
Q: Can I assign null to a string variable?
A: Yes, you can assign null to a string variable, as a string is a reference type in most programming languages. Assigning null to a string variable indicates that it does not currently hold a valid string object.
Q: What happens if I access a member of a variable assigned null?
A: If you attempt to access a member (property or method) of a variable assigned null, you will encounter a null-reference exception at runtime. It is crucial to handle null references appropriately by performing null checks before accessing members.
Q: Can I assign null to a class instance?
A: Yes, you can assign null to a class instance by assigning null to the reference type variable holding the instance. Null signifies that the variable does not currently reference any specific instance of the class.
Q: Is assigning null necessary when declaring a reference type variable?
A: No, it is not necessary to assign null when declaring a reference type variable. By default, reference type variables are assigned null if not explicitly initialized.
Q: Can I assign null to an array?
A: Yes, you can assign null to an array variable, as an array is also a reference type. Assigning null indicates the absence of any valid array object.
In conclusion, assigning null to reference types is a standard practice and serves the purpose of indicating the absence of an object or the lack of a valid value. It is crucial, however, to handle null references appropriately to prevent null-reference exceptions. By understanding the concepts covered in this article and employing proper null checks, you can effectively utilize null in your programming endeavors.
How To Disable Nullable Reference Types In C#?
C# 8.0 introduced a significant improvement to the language: Nullable Reference Types. This feature enhances the type system to make it more expressive and helps developers avoid NullReferenceExceptions at runtime. However, there might be situations where you want to disable nullable reference types and opt-out of this new behavior. In this article, we will delve into the details of nullable reference types, discuss when and why you might want to disable them, and explore how to do so effectively.
Understanding Nullable Reference Types
Nullable Reference Types aim to address one of the most common pitfalls in programming: null references. NullReferenceExceptions occur when we attempt to access members or invoke methods on variables that are null. To mitigate this problem, C# 8.0 introduced nullable reference types to provide better compile-time guarantees about null safety.
With nullable reference types, the compiler is more strict in enforcing non-nullability constraints and encourages developers to handle null cases explicitly. By default, all reference types are considered nullable, meaning they can hold null values, unless explicitly marked as non-nullable with the “!” operator.
When Do You Want to Disable Nullable Reference Types?
Although nullable reference types offer significant benefits, there are scenarios where disabling them might be desirable. Here are a few reasons why you might want to opt-out of this feature:
1. Legacy Codebases: If you are working on a large codebase that wasn’t originally designed with nullable reference types in mind, enabling them might become overwhelming. Disabling the feature ensures compatibility with existing code and avoids potential breaking changes.
2. Compiler Warnings: The strictness of nullable reference types can lead to a barrage of compiler warnings, particularly in larger codebases or older projects. Disabling them can help reduce noise during development, allowing you to focus on critical issues.
3. Performance Considerations: Although nullable reference types primarily impact compile-time safety, there might be situations where runtime performance is more critical. Disabling nullable reference types can help minimize the overhead introduced by additional null checks.
Disabling Nullable Reference Types
To disable nullable reference types, you need to modify your project settings. Here’s how you can do it:
1. Visual Studio: If you’re using Visual Studio, open the project properties and navigate to the “Build” tab. Under the “Errors and warnings” section, you will find the nullable reference types option. Set it to “Disable” or “Warnings” as per your preference.
2. .NET Core CLI: For command-line enthusiasts, you can disable nullable reference types by adding the following property to your project file (.csproj):
“`
“`
3. MSBuild: For advanced customization, you can edit the MSBuild configuration file to disable nullable reference types. Add the following lines to your .csproj:
“`
“`
Potential Pitfalls and FAQs
While disabling nullable reference types may seem like a quick solution, it’s important to consider a few potential pitfalls and frequently asked questions before proceeding:
Q: Will disabling nullable reference types affect my existing codebase?
A: No, disabling nullable reference types will not introduce any breaking changes to your existing codebase. It will merely revert the compile-time behavior to the pre-C# 8.0 style.
Q: Can I disable nullable reference types for specific parts of my codebase?
A: Unfortunately, disabling nullable reference types is a global configuration setting affecting the entire project. There is no built-in mechanism to selectively disable the feature for specific parts of the code.
Q: Disabling nullable reference types reduces compile-time safety. Does it mean I lose earlier detection of null-related bugs?
A: Yes, disabling nullable reference types eliminates some of the compile-time guarantees provided by the feature. However, it does not automatically lead to more runtime null-related bugs. Diligent testing and defensive programming practices can still mitigate such issues.
Q: Should I permanently disable nullable reference types?
A: It’s generally recommended to enable nullable reference types and embrace the improved null safety guarantees they offer. Only consider disabling them in exceptional cases where the constraints outweigh the benefits.
Conclusion
Nullable Reference Types in C# 8.0 enhance the language by providing compile-time guarantees about null safety. However, there might be situations where you need to disable them, such as working with legacy codebases or minimizing compiler warnings. By adjusting project settings in Visual Studio or editing the project file directly, you can disable nullable reference types. Remember, careful consideration should be given before disabling this feature, as it might introduce potential risks to the correctness and maintainability of your codebase.
Keywords searched by users: cannot convert null literal to non-nullable reference type converting null literal or possible null value to non-nullable type, cannot convert null value to non nullable type, Cannot convert null literal to non nullable reference type, jsonconvert.deserializeobject converting null literal or possible null value to non-nullable type, c# turn off nullable reference types, expression is always false according to nullable reference types annotations, c# non nullable list, possible null assignment to non nullable entity
Categories: Top 88 Cannot Convert Null Literal To Non-Nullable Reference Type
See more here: nhanvietluanvan.com
Converting Null Literal Or Possible Null Value To Non-Nullable Type
In the world of programming, null is a commonly used value that represents the absence of a valid reference or value. It is often used to indicate that a variable has not been assigned any value or does not exist. However, null values can present challenges when you’re working with non-nullable types, which have strict rules that they must be assigned a valid value before they can be used. In this article, we will explore the concept of converting null literals or possible null values to non-nullable types and how this process can be handled effectively.
Understanding Non-Nullable Types
Before delving into the process of converting null values, it’s essential to have a clear understanding of non-nullable types. A non-nullable type is a data type that cannot contain a null value. This means that you must always provide a valid value to the non-nullable type before it can be used or accessed. This characteristic helps to ensure that variables are not used in an invalid or uninitialized state, reducing the chances of runtime errors and bugs in your code.
Converting Null Literal to a Non-Nullable Type
One of the scenarios where null values can pose a problem is when you want to assign a null literal to a non-nullable type. In languages like C# or Java, this operation will result in a compilation error. The compiler recognizes that the value you are trying to assign is null, which violates the non-nullable type’s rules. To resolve this issue, you need to either change the non-nullable type to a nullable type or provide a non-null value.
If you want to change the non-nullable type to a nullable type, you can add a question mark (?) after the data type. This modification allows the variable to accept null values, making it compatible with assigning null literals. However, you need to be aware of the implications of changing a non-nullable type to a nullable one, as it might require additional null checks in your code to handle potential null values.
On the other hand, if modifying the type to a nullable type is not a viable solution, you will need to provide a non-null value. In some cases, you may have a default value that can be assigned when a null value is encountered. However, if a default value does not exist, you will need to consider alternate approaches, such as throwing an exception or using conditional statements to handle the null value explicitly.
Converting Possible Null Values to Non-Nullable Types
Another scenario that developers often encounter is when dealing with variables that have the potential to be null. This situation can arise when you receive data from external sources, such as user inputs, databases, or APIs. While you may have validation mechanisms in place, it’s challenging to guarantee that the value will never be null.
To convert possible null values to a non-nullable type, you can utilize techniques like null-coalescing or null-conditional operators. Null-coalescing (??) is an operator that evaluates the expression to the left of it and returns the value if it is not null. If the expression is null, it evaluates the expression to the right of it and returns that value instead. This technique allows you to provide a fallback value if the original value is null.
The null-conditional operator is represented by a question mark (?.) and can be used in conjunction with null-coalescing or other operators. It allows you to access properties or methods of an object only if the object itself is not null. If the object is null, the expression short-circuits, and the overall value becomes null.
By utilizing these operators effectively, you can retrieve non-null values from potentially null variables, ensuring compatibility with non-nullable types. However, it’s crucial to exercise caution when using these operators, as they might introduce new complexities and potential null reference errors if not implemented correctly.
FAQs:
Q: Why does a non-nullable type not allow null values?
A: Non-nullable types are designed with the intention of ensuring that variables are always in a valid and initialized state before being used. This feature helps to reduce runtime errors caused by null reference exceptions and promotes safer programming practices.
Q: What are some common scenarios where null values can cause problems?
A: Null values can be problematic when assigning null literals to non-nullable types or when dealing with variables that have the potential to be null, such as user inputs or data from external sources.
Q: How can I convert a null literal to a non-nullable type?
A: To convert a null literal to a non-nullable type, you can either change the non-nullable type to a nullable type by adding a question mark (?) after the data type or provide a non-null value explicitly.
Q: Can I assign null values to non-nullable types without modifying the type?
A: No, assigning null values to non-nullable types without modifying the type will result in a compilation error. The language compiler recognizes that null is not a valid value for non-nullable types.
Q: How can I handle possible null values when converting to non-nullable types?
A: Techniques like null-coalescing (??) and null-conditional operators (?.) can be used to convert possible null values to non-nullable types. These operators provide mechanisms to handle null values effectively and provide fallback values or null checks when necessary.
In conclusion, converting null literals or possible null values to non-nullable types requires careful consideration and understanding of the language’s syntax and features. By utilizing techniques like modifying the type to a nullable type, providing non-null values, or leveraging null-coalescing and null-conditional operators, you can ensure compatibility and handle null values effectively in your code.
Cannot Convert Null Value To Non Nullable Type
When working with programming languages like C#, you may come across an error message that says “cannot convert null value to non-nullable type.” This error typically occurs when you are trying to assign a null value to a variable of a type that doesn’t allow null values. In this article, we will dive deep into this error, understand its causes, and explore different ways to handle it effectively.
Understanding the Error:
In C# and other statically-typed languages, each variable must be explicitly declared with a specific type. Some types, such as int, double, and bool, are considered non-nullable types because they don’t allow null values. This means that if you try to assign a null value to a variable of a non-nullable type, you will encounter the “cannot convert null value to non-nullable type” error.
Causes of the Error:
There can be several reasons for encountering this error. Let’s take a look at a few common scenarios that can lead to this error:
1. Null Assignment: One of the most common causes of this error is directly assigning null to a variable that doesn’t accept null values. For example:
“`
int a = null; // Error: cannot convert null to ‘int’
“`
2. Null Return: Another possible cause is when a method or function returns a null value, and you attempt to assign it to a non-nullable variable. For example:
“`
string GetString() {
return null;
}
string result = GetString(); // Error: cannot convert null to ‘string’
“`
Handling the Error:
Now that we understand the causes of this error, let’s explore some ways to handle it effectively:
1. Use Nullable Types: One solution is to use nullable types explicitly by appending a question mark (?) after the type name. This allows the variable to accept null values in addition to its normal data type. For example:
“`
int? a = null; // Now ‘a’ can accept null values
“`
2. Check for Null before Assignment: Prior to assigning a value to a non-nullable variable, it is a good practice to first check if the value coming in is null. If it is, you can either assign a default value or handle the null case differently. For example:
“`
string s = GetString(); // Assuming GetString() can return null
if (s != null) {
// handle the non-null value
} else {
// handle the null case
}
“`
3. Use Conditional Operators: Conditional (ternary) operators can be handy when dealing with null values. They allow you to perform conditional assignments based on a condition. For example:
“`
string s = GetString();
string result = (s != null) ? s : “Default Value”;
“`
FAQs (Frequently Asked Questions):
Q: What does the error message “cannot convert null value to non-nullable type” mean?
A: This error occurs when you are trying to assign null to a variable of a type that doesn’t allow null values, such as int, bool, or double.
Q: Why can’t non-nullable types accept null values?
A: Non-nullable types are designed to always have a value assigned. Allowing null values could potentially lead to runtime errors where unexpected null values are encountered.
Q: How can I fix the “cannot convert null value to non-nullable type” error?
A: There are several ways to handle this error, including using nullable types, checking for null before assignment, or using conditional operators.
Q: Can I change a non-nullable type to accept null values in C#?
A: No, once a variable is declared as a non-nullable type, it cannot be changed to accept null values directly. You would need to use nullable types explicitly.
Q: Are there any alternative languages or approaches that allow non-nullable types to accept null values?
A: Yes, some programming languages, such as TypeScript, have introduced optional types that allow variables to accept either the assigned type or null.
In conclusion, encountering the “cannot convert null value to non-nullable type” error is a common occurrence when working with statically-typed languages like C#. By understanding the causes and implementing appropriate handling mechanisms, you can effectively deal with this error and ensure the smooth execution of your code. Remember to use nullable types, check for null before assignment, and leverage conditional operators to handle null values appropriately.
Images related to the topic cannot convert null literal to non-nullable reference type
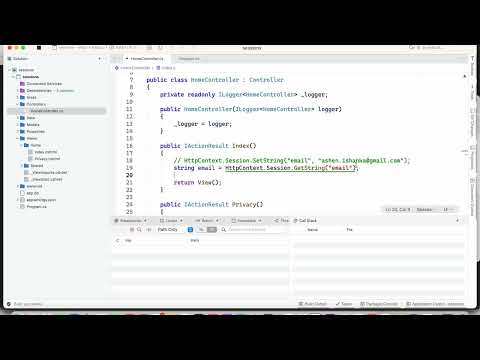
Found 22 images related to cannot convert null literal to non-nullable reference type theme
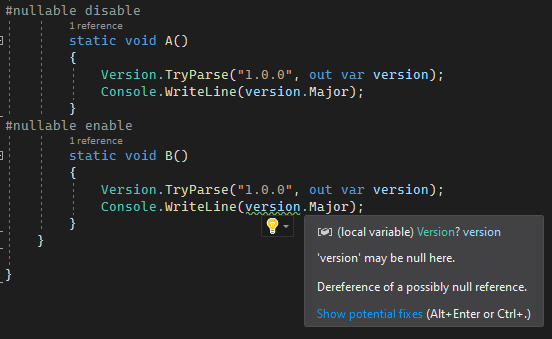
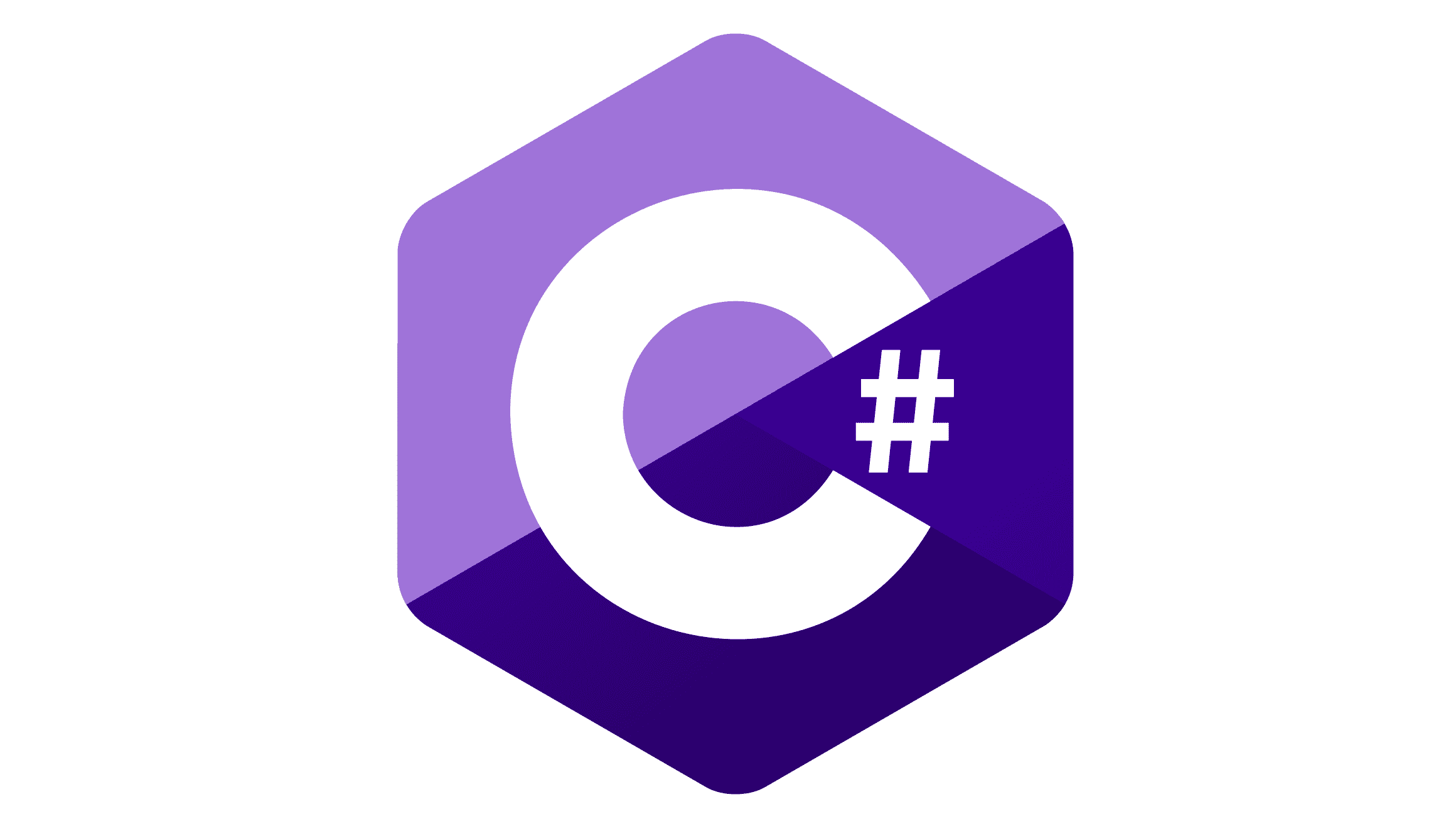
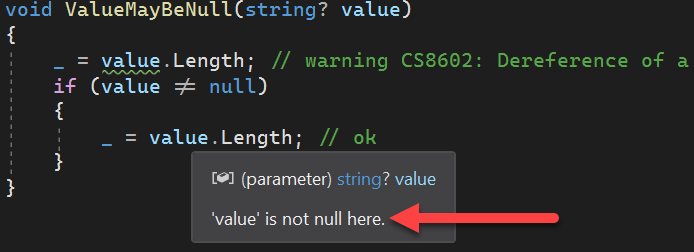

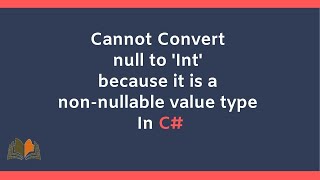
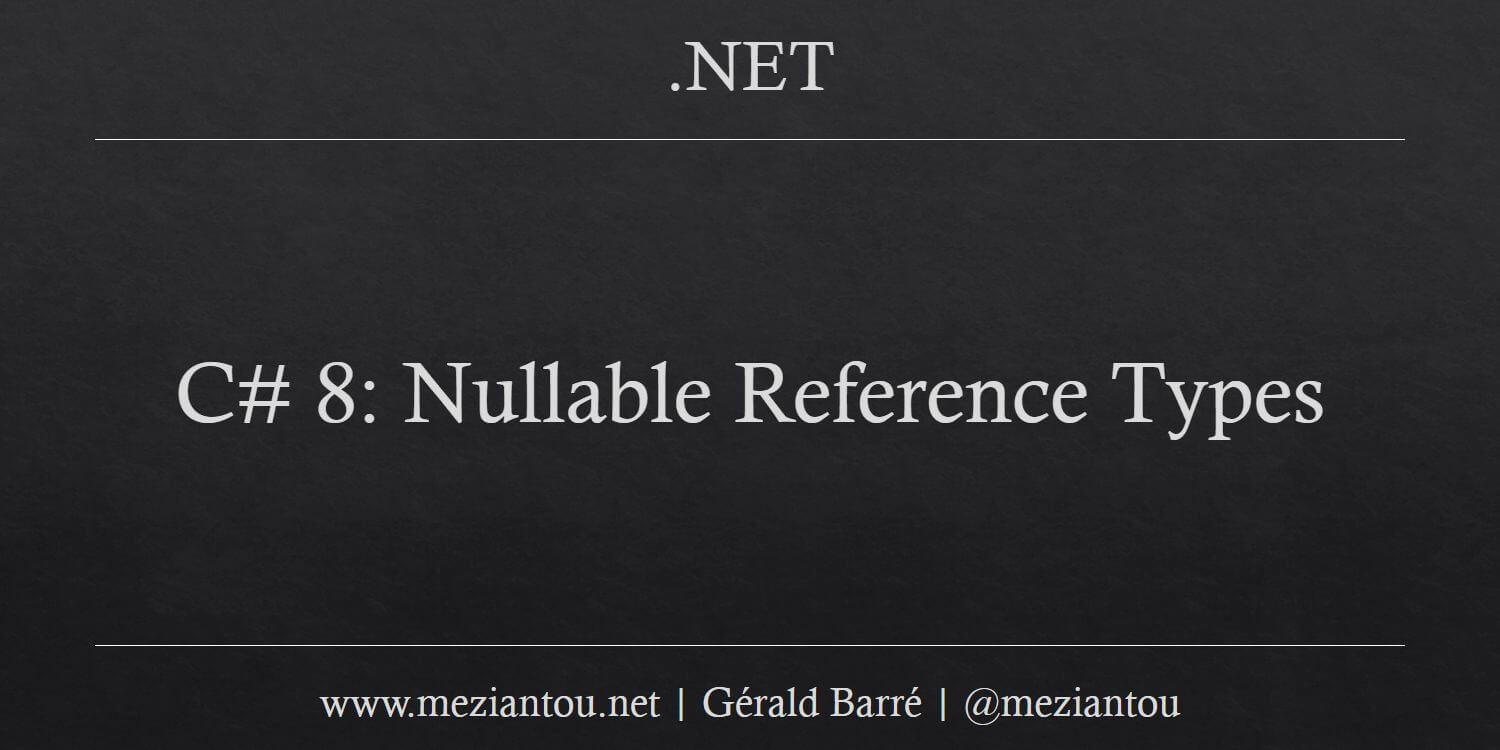



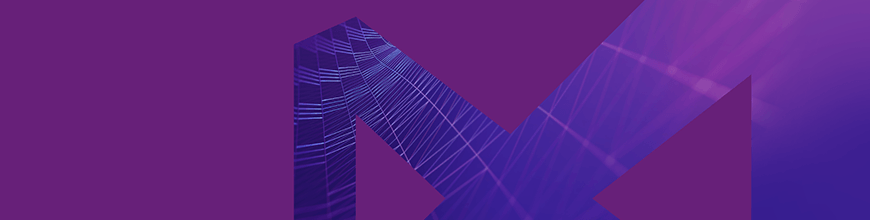






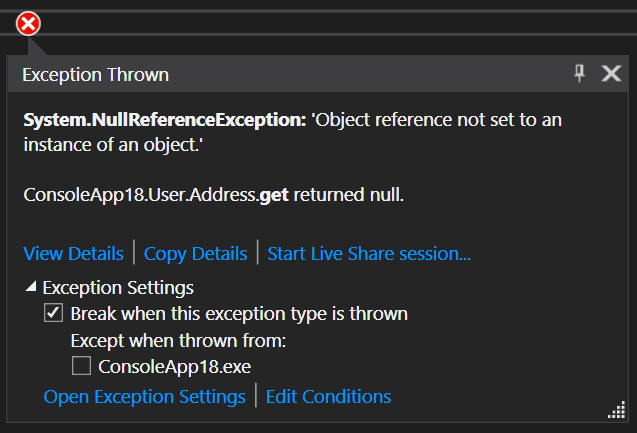





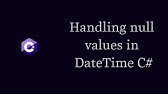
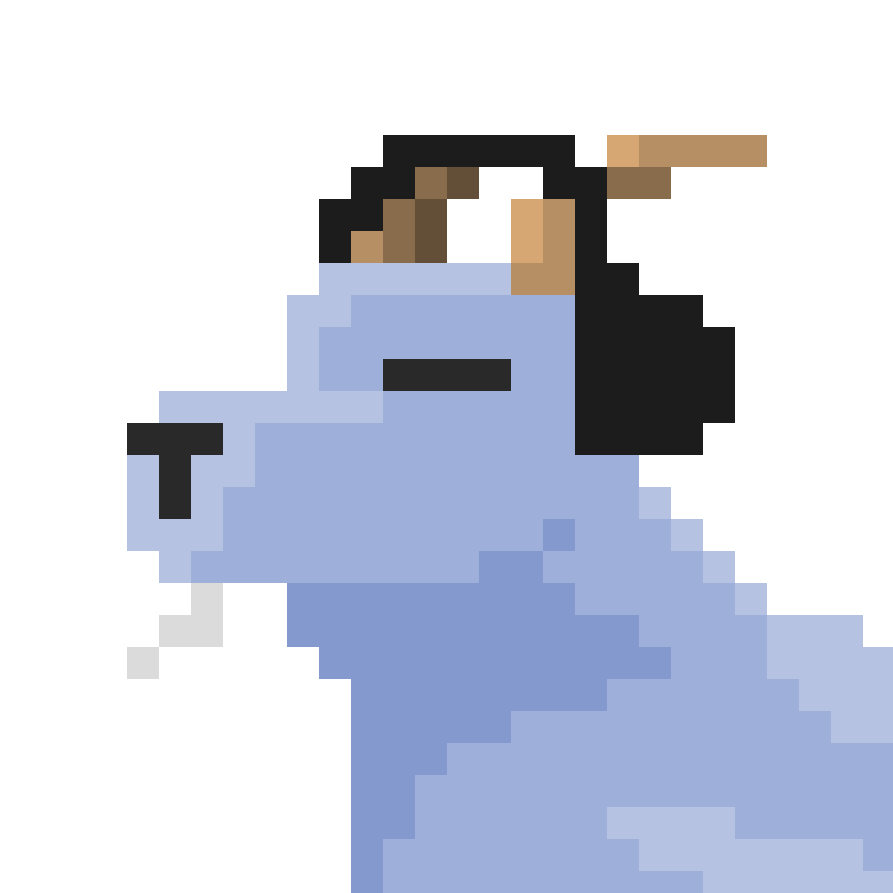


Article link: cannot convert null literal to non-nullable reference type.
Learn more about the topic cannot convert null literal to non-nullable reference type.
- Nullable Reference Types in C# | CodeGuru.com
- “Cannot convert null literal to non-nullable reference type …
- Containing Null with C# 8 Nullable References – praeclarum
- Nullable reference types – C# reference – Microsoft Learn
- Nullable reference types – Microsoft Learn
- Nullable & Non-Nullable Types – Elements Docs
- Disabling Null Checking – The C# Player’s Guide
- False “cannot convert null literal to non-nullable reference …
- [Blazor] CS8625: Cannot convert null literal to non-nullable …
- cannot convert null literal to non-nullable reference type c# …
- Nullable reference types in C# | My Memory
See more: blog https://nhanvietluanvan.com/luat-hoc