C# Foreach With Index
The foreach loop is a powerful construct in C# that allows you to easily iterate over elements in a collection. In many cases, you may find the need to access both the elements and their corresponding indexes while iterating. This is where the “foreach with index” comes into play.
In this article, we will explore the basic syntax of the foreach loop, learn how to iterate over different types of collections, access elements and their indexes, and delve into advanced topics such as nesting loops, using break and continue statements, and performance considerations.
Basic Syntax:
The basic syntax of the foreach loop in C# is as follows:
foreach (var element in collection)
{
// Code to be executed on each element
}
In this structure, the “element” variable represents the current element being examined, and the “collection” is the collection of items being iterated. The foreach loop automates the process of iterating through a collection, allowing you to focus on the logic specific to each element.
Iterating a List:
To iterate over a List using foreach with index, you can obtain the index of each element by using the `List
List
foreach (string fruit in fruits)
{
int index = fruits.IndexOf(fruit);
Console.WriteLine($”Element at index {index}: {fruit}”);
}
This will output:
Element at index 0: Apple
Element at index 1: Orange
Element at index 2: Banana
Iterating an Array:
When iterating over an array using foreach with index, you can directly access the index of each element using the built-in C# index operator ([]). Here’s an example:
string[] colors = { “Red”, “Green”, “Blue” };
foreach (string color in colors)
{
int index = Array.IndexOf(colors, color);
Console.WriteLine($”Element at index {index}: {color}”);
}
This will output:
Element at index 0: Red
Element at index 1: Green
Element at index 2: Blue
Iterating a String:
To iterate over a string using foreach with index, you can convert the string to a character array and then iterate over it. Here’s an example:
string message = “Hello World”;
foreach (char c in message.ToCharArray())
{
int index = message.IndexOf(c);
Console.WriteLine($”Character at index {index}: {c}”);
}
This will output:
Character at index 0: H
Character at index 1: e
Character at index 2: l
Character at index 3: l
Character at index 4: o
Character at index 5:
Character at index 6: W
Character at index 7: o
Character at index 8: r
Character at index 9: l
Character at index 10: d
Nested foreach loops with index:
You can nest foreach loops with index to iterate over collections within collections. Here’s an example:
int[,] matrix = { { 1, 2 }, { 3, 4 } };
foreach (int element in matrix)
{
int rowIndex = Array.IndexOf(matrix, element) / matrix.GetLength(1);
int colIndex = Array.IndexOf(matrix, element) % matrix.GetLength(1);
Console.WriteLine($”Element at [{rowIndex}, {colIndex}]: {element}”);
}
This will output:
Element at [0, 0]: 1
Element at [0, 1]: 2
Element at [1, 0]: 3
Element at [1, 1]: 4
Using break and continue statements with foreach loop:
Like in traditional for loops, you can use the break statement to exit the foreach loop prematurely, and the continue statement to skip the rest of the current iteration and move to the next element. Here’s an example:
string[] names = { “Alice”, “Bob”, “Charlie”, “Dave”, “Eve” };
foreach (string name in names)
{
if (name.Length % 2 == 0)
continue;
Console.WriteLine(name);
if (name.Contains(“l”))
break;
}
This will output:
Alice
Charlie
Performance Considerations:
While foreach with index can be convenient, it may introduce a slight performance impact compared to using a traditional for loop. However, the difference is generally negligible unless you’re dealing with massive collections. When choosing between foreach with index and for loop, consider factors such as readability, maintainability, and the specific requirements of your code.
Other Use Cases and Applications:
Foreach with index can be useful in a variety of scenarios. Some examples include:
1. Finding the first occurrence of an element matching a specific condition in a collection.
2. Implementing custom logic based on the index and value of each element.
3. Performing operations on multiple collections in parallel using nested loops.
4. Updating elements in a collection without modifying their indexes.
Advanced Techniques and Tips:
When working with foreach with index, here are some additional tips:
1. Be cautious when modifying the collection being iterated. This may result in unexpected behavior.
2. If you’re dealing with a large collection and performance is critical, consider using a for loop instead.
3. Take advantage of LINQ to simplify complex queries and transformations on collections.
4. Familiarize yourself with the various collection types in .NET to reap the benefits of foreach with index in different contexts.
In conclusion, foreach with index is a powerful feature in C# that allows you to iterate over collections while accessing both elements and their indexes. It simplifies the process of working with collections and enables you to write cleaner and more readable code. By understanding the syntax and exploring different use cases, you can leverage this functionality to its fullest potential in your C# programs.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# foreach with index
Categories: Top 66 C# Foreach With Index
See more here: nhanvietluanvan.com
Images related to the topic c# foreach with index
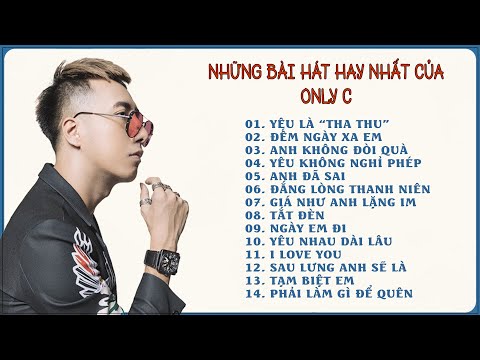
Article link: c# foreach with index.
Learn more about the topic c# foreach with index.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- C – Wiktionary tiếng Việt
See more: blog https://nhanvietluanvan.com/luat-hoc