Javascript Style.Left Not Working
One of the common tasks in JavaScript is to manipulate the style of elements on a webpage. This is often done using the style property, which allows you to modify various CSS properties of an element dynamically. One such property is style.left, which is used to control the horizontal position of an element.
However, there are times when the style.left property may not work as expected, causing frustration for developers. In this article, we will explore some possible reasons why the style.left property may not be working and provide solutions to resolve these issues.
Understanding the style.left property and its purpose in JavaScript
The style.left property is part of the CSSStyleDeclaration interface in JavaScript. It allows you to set or get the value of the “left” CSS property of an element. The “left” property determines the horizontal position of an element relative to its containing element.
When setting the value of style.left, you must provide a unit of measurement for the position, such as pixels (px), percentage (%), or any other valid CSS unit. For example, if you want to move an element 50 pixels to the right, you can set style.left = “50px”.
Possible reasons why the style.left property may not be working
1. Syntax errors and typos: The most common reason for the style.left property not working is a syntax error or a typo in your JavaScript code. Double-check your code for any missing or misplaced characters, such as semicolons or quotation marks.
2. Incorrect positioning: The style.left property only works if an element has a positioning value other than “static”. Elements with a static position are not affected by the style.left property. Make sure the element you are trying to style has a positioning value of “relative”, “absolute”, or “fixed”.
3. Conflicting CSS properties and rules: Another reason why the style.left property may not work is due to conflicting CSS properties or rules. Check if there are any other CSS properties or rules that may affect the horizontal positioning of the element. For example, if there is a CSS rule that sets the “left” property using !important, it will override any changes made through the style.left property.
Checking for syntax errors and typos in the code
Before troubleshooting why the style.left property is not working, it’s important to double-check your code for any syntax errors or typos. Even a small error can prevent the code from executing correctly.
Make sure that you have assigned the correct value to the style.left property and that it includes a valid unit of measurement. For example, style.left = “50px” is a valid assignment, while style.left = “50” or style.left = “50 pixels” are not.
Verifying that the element has the correct positioning
To use the style.left property, the element you want to style must have a positioning value other than “static”. By default, elements have a positioning value of “static”, which means they are not affected by the style.left property.
Make sure that the element you are trying to style has a positioning value of “relative”, “absolute”, or “fixed”. You can set the positioning value using CSS, for example:
element.style.position = “relative”;
Examining conflicting CSS properties and rules that may affect the style.left property
If the style.left property is not working as expected, it’s important to check if there are any conflicting CSS properties or rules that may be affecting the horizontal positioning of the element. These conflicting properties or rules can override the changes made through the style.left property.
Inspect the CSS rules for the element using browser developer tools. Look for any CSS rules that may affect the “left” property. For example, if there is a CSS rule that sets the “left” property using !important, it will override any changes made through the style.left property.
Exploring alternative ways to set the position of an element using JavaScript
If you are still encountering issues with the style.left property, there are alternative ways to set the position of an element using JavaScript. One approach is to use the element.style.setProperty() method, which allows you to set any CSS property, including the “left” property.
Here is an example of setting the “left” property using the setProperty() method:
element.style.setProperty(“left”, “50px”);
By using this method, you can ensure that the “left” property is set correctly, regardless of any conflicting CSS rules or properties.
FAQs
Q: Why is my style.left property not working?
A: There can be several reasons why the style.left property is not working. Some common issues include syntax errors, incorrect positioning of the element, and conflicting CSS properties or rules. Double-check your code for errors and make sure the element has the correct positioning.
Q: Can I use percentages with the style.left property?
A: Yes, you can use percentages as a unit of measurement for the style.left property. For example, style.left = “50%” will position the element halfway across its containing element.
Q: What is the difference between getComputedStyle and style.left?
A: The getComputedStyle() method returns the computed style of an element, while the style.left property allows you to directly set or get the value of the “left” CSS property. The getComputedStyle() method can be useful when you need to retrieve the actual value for the “left” property that may be affected by CSS inheritance or other factors.
Q: How can I change the position of a button using JavaScript?
A: To change the position of a button or any other element using JavaScript, you can modify the value of the style.left property or use alternative methods like setProperty(). Make sure the element has the correct positioning and there are no conflicting CSS properties or rules affecting the position.
Q: How can I get the computed height of an element using JavaScript?
A: To get the computed height of an element using JavaScript, you can use the getComputedStyle() method and retrieve the value of the “height” property. For example:
const element = document.getElementById(“myElement”);
const computedHeight = window.getComputedStyle(element).height;
console.log(computedHeight);
Html : Javascript Element.Style.Left Not Working
How To Set Left Using Javascript?
JavaScript is a powerful programming language that enables developers to add interactive elements and dynamic behavior to websites. One key aspect of JavaScript is manipulating the positioning of HTML elements on a webpage. One commonly used property for positioning is the “left” property, which allows you to set the distance between the element’s left edge and the left edge of its containing element. In this article, we will delve into the details of setting the left property using JavaScript, providing you with a comprehensive guide to help you master this skill.
Understanding the Left Property:
Before diving into how to set the left property using JavaScript, it is essential to have a basic understanding of how this property works. The left property is used for positioning elements within a webpage and is usually applied to elements with a position value of “fixed”, “absolute”, or “relative.” The left property accepts any valid CSS length value, such as pixels (px), percentages (%), or em units.
Setting the Left Property Using JavaScript:
To set the left property using JavaScript, you need to access the DOM (Document Object Model), which represents the structure of your webpage. Here are the steps to accomplish this task:
Step 1: Select the element you want to manipulate:
Before setting the left property, you need to select the HTML element to which you want to apply the positioning. This can be done using various DOM manipulation methods such as getElementById, querySelector, or getElementsByClassName.
Step 2: Assign a value to the left property:
Once you have selected the desired HTML element, you can set its left property by assigning a value to it. For example, if you want to set the left property of an element with the id “myElement” to 200 pixels, you can use the following code:
document.getElementById(“myElement”).style.left = “200px”;
Step 3: Apply a position value:
Before setting the left property, make sure that the element has a position value of either “fixed”, “absolute”, or “relative”. For example, if you want to set the left property of an element with the id “myElement” to 200 pixels, you can modify the previous code as follows:
var element = document.getElementById(“myElement”);
element.style.position = “relative”;
element.style.left = “200px”;
This code will set the position property to “relative” and then assign a value of 200 pixels to the left property.
FAQs:
Q: Can I use the left property with elements having a position value of “static”?
A: No, the left property only works with elements that have a position value of either “fixed”, “absolute”, or “relative”. Elements with a position value of “static” will ignore the left property.
Q: Can I set the left property using a percentage value?
A: Yes, the left property can be set using percentage values. For example, if you want to set the left property to 50% of its containing element’s width, you can use the following code:
var element = document.getElementById(“myElement”);
element.style.position = “relative”;
element.style.left = “50%”;
Q: How can I dynamically change the left property using JavaScript?
A: To dynamically change the left property, you can use JavaScript to calculate the desired value based on user input or certain conditions. For example, you can use event listeners to trigger a function that modifies the left property based on user interactions.
Q: Can I set the left property for multiple elements at once?
A: Yes, you can set the left property for multiple elements by selecting them using a method like getElementsByClassName or querySelectorAll, and then loop through the selected elements to apply the desired left value.
In conclusion, setting the left property using JavaScript provides developers with a powerful tool to position elements on a webpage dynamically. By understanding the basic concepts and following the steps outlined in this guide, you can easily manipulate the left property of HTML elements to create visually appealing and interactive web experiences. Happy coding!
How To Change Left Position Of Element In Javascript?
Positioning elements on a web page is a crucial aspect of web development. In JavaScript, the left position of an element can be easily modified to achieve the desired layout and visual effects. This article will guide you through the process of changing the left position of an element using different techniques. We will delve into the concepts of absolute and relative positioning, as well as demonstrate how to adjust left position dynamically.
Understanding Absolute and Relative Positioning
Before we dive into changing the left position of elements, let’s first understand the two primary forms of positioning in CSS: absolute and relative.
1. Absolute Positioning:
When an element is set to an absolute position, it is positioned relative to its nearest positioned ancestor (i.e., an ancestor element with a position value of “relative”, “fixed”, “absolute”, or “sticky”). If no such ancestor exists, the element will be positioned relative to the initial container, which is usually the document’s body. By setting the left property of an element with absolute positioning, you can specify its exact distance from the left side of its containing element.
2. Relative Positioning:
In contrast to absolute positioning, relative positioning allows you to shift an element’s position relative to its default position. By setting the left property of an element with relative positioning, you can move it horizontally from its default location.
Modifying the Left Position using JavaScript:
Now that we have a basic understanding of positioning, let’s explore how to change the left position of an element using JavaScript. We will discuss different scenarios, starting from static placement to dynamically altering the left position:
1. Static Placement:
To statically set an element’s left position in JavaScript, you can access the style property of the element and assign a value to the left property. Here’s an example:
“`
var element = document.getElementById(“myElement”);
element.style.left = “100px”;
“`
In this code snippet, the element with the ID “myElement” is assigned a left position of 100px.
2. Relative Adjustment:
To adjust the left position of an element relative to its current position, you can retrieve the current left position using the offsetLeft property and add or subtract a desired value. Here’s an example:
“`
var element = document.getElementById(“myElement”);
var currentPosition = element.offsetLeft; // Retrieves the current left position
element.style.left = currentPosition + 50 + “px”; // Adjust the left position by adding 50 pixels
“`
In this example, 50 pixels are added to the current left position of the element, resulting in a shift to the right.
3. Dynamic Adjustment:
If you wish to dynamically change the left position of an element in response to a user action or event, you can utilize event listeners in JavaScript. Here’s an example using a button click event:
“`
var element = document.getElementById(“myElement”);
var button = document.getElementById(“myButton”);
button.addEventListener(“click”, function() {
var currentPosition = element.offsetLeft;
element.style.left = currentPosition + 50 + “px”;
});
“`
In this code snippet, the left position of the element is adjusted by 50 pixels whenever the button with the ID “myButton” is clicked.
FAQs:
Q: Can the left position be changed for elements that are not positioned absolutely or relatively?
A: No, in order to change the left position, an element must be positioned using either absolute or relative positioning. Elements with static positioning cannot have their left position modified.
Q: Can negative values be assigned to the left property?
A: Yes, negative values can be assigned to the left property to shift an element to the left of its default or current position.
Q: Can the left position of an element be animated?
A: Yes, the left position of an element can be animated by using JavaScript animations or CSS transitions. By gradually changing the left value over time, smooth animations can be achieved.
Q: Can the left position be set as a percentage value?
A: Yes, the left position can be set as a percentage value (e.g., “50%”) relative to the container element’s width.
Q: How can I reset the left position of an element to its default or initial state?
A: To reset the left position of an element to its default or initial state, you can set the left property to “auto” using JavaScript:
“`
var element = document.getElementById(“myElement”);
element.style.left = “auto”;
“`
Conclusion:
In JavaScript, the left position of elements can easily be modified using various techniques. Whether you prefer static placement, relative adjustment, or dynamic positioning, understanding the principles of absolute and relative positioning is key. By implementing the techniques outlined in this article, you will gain the ability to precisely position elements on your web page, leading to more visually appealing and user-friendly designs.
Keywords searched by users: javascript style.left not working Style left not working, set computed style javascript, style.top javascript, getcomputedstyle vs style, left in javascript, javascript position change, javascript move button position, javascript get computed height
Categories: Top 48 Javascript Style.Left Not Working
See more here: nhanvietluanvan.com
Style Left Not Working
Introduction (90 words)
The world of English grammar can be puzzling, with various rules and exceptions to navigate. One particular aspect that often confuses learners is the concept of “style left.” In some languages, word order can be modified for emphasis, but in English, such modifications rarely result in the desired effect. This article delves deep into why “style left” doesn’t work effectively in English, exploring its idiosyncrasies and clarifying any misconceptions. If you’ve ever been puzzled by this grammatical quandary, read on for a comprehensive breakdown.
The Nature of Style Left (120 words)
In certain languages, such as Japanese or Turkish, altering word order within a sentence can place emphasis on specific parts of speech, conveying nuances of meaning. In contrast, English has a relatively fixed word order, known as Subject-Verb-Object (SVO), with some minor variations depending on grammatical context. As a result, attempting to utilize “style left” in English, where the focus of the sentence is pushed towards the beginning, often leads to unnatural language constructs that can confuse native speakers and impede effective communication.
The Limitations of “Style Left” in English (180 words)
1. Syntax and Anticipatory Stress: English relies heavily on syntax and anticipatory stress to convey meaning. By adhering to the SVO structure, listeners can anticipate the word that will carry the most importance, making messages clearer. When “style left” is employed, it disrupts our natural anticipation, creating ambiguity and hindering comprehension.
2. Information Structure and Clarity: English provides clarity through its information structure. By placing crucial information towards the end of a sentence, it helps build suspense or introduces a climax. “Style left” disrupts this structure, making messages less coherent, nuanced, and less emotionally impactful.
3. Emphasis Through Alternatives: English has other effective ways to emphasize words without relying on “style left.” Techniques, such as intonation, stress, and modal auxiliary verbs, prove more successful in conveying emphasis and the intended meaning while maintaining grammatical integrity.
Addressing Common Misconceptions (240 words)
1. Isn’t German “style left”? While German does exhibit some flexible word order, akin to “style left,” it is essential to note that German is a more inflected language, and word order is largely guided by strict case and agreement systems, allowing greater flexibility. English is considerably less flexible due to its reliance on other grammatical cues.
2. But what about rhetorical questions? Although rhetorical questions can appear to modify word order in English, they still adhere to the SVO structure. The inversion or reordering in rhetorical questions is a rhetorical device in itself and does not fall under the “style left” concept.
3. Can’t “style left” be used for poetic or creative purposes? While poetry and creative writing may offer some leeway in word order, the principle of “style left” is still relatively ineffective in English. Creative expressions rely more on an array of poetic devices rather than a simple reversal of word order.
FAQs (150 words)
Q: Are there any exceptions to “style left” in English?
A: There are a few minor instances and exceptions, usually occurring in informal spoken English, such as “Good he was not” instead of “He was not good.” However, these examples represent colloquial usage and are not considered standard English.
Q: Can a non-native speaker ever effectively use “style left” in English?
A: While mastering any language includes adopting its grammar rules, a non-native speaker can still convey emphasis through other linguistic aspects. Understanding the cultural and linguistic context is crucial for effectively conveying meaning in English.
Q: Should English learners bother with “style left” at all?
A: It is more advisable for English learners to focus on mastering the complexities of English grammar rules, structures, and expressions, rather than trying to force “style left” into their constructs. Concentrating on nuances, clarity, and effective communication within the confines of standard English will yield better results.
Conclusion (90 words)
“Style left” might be a feasible concept in some languages, but its transferability to English is limited. The fixed SVO structure, heavy reliance on anticipatory stress, and information flow dynamics all contribute to the ineffectiveness of “style left” in English. While linguistic rules continually evolve, understanding why “style left” doesn’t work in English helps learners navigate the complexities of the language with more confidence and clarity.
Set Computed Style Javascript
Styles play a crucial role in creating visually appealing web pages. Cascading Style Sheets (CSS) enable developers to easily control the presentation and layout of HTML elements. However, there may be situations where you need to dynamically modify these styles using JavaScript. In such cases, the setComputedStyle method comes to the rescue. In this article, we will explore the setComputedStyle method in depth, discussing its purpose, syntax, usage, and various examples. So, let’s dive in!
**What is the setComputedStyle method?**
The setComputedStyle method is a JavaScript function that allows developers to directly modify the styles of HTML elements programmatically. Unlike changing styles via the style property, which affects inline styles only, setComputedStyle enables you to modify any style property regardless of its origin — inline, internal, or external CSS.
**Syntax of setComputedStyle**
Before diving into the usage, let’s first understand the syntax of the setComputedStyle method:
“`javascript
element.setComputedStyle(property, value);
“`
Here, `element` refers to the HTML element on which the style needs to be modified. `property` is the style property you want to change, and `value` is the new value assigned to that property.
**Usage of setComputedStyle**
Now that we understand the syntax, let’s explore some common use cases where the setComputedStyle method can prove handy:
1. **Changing font color dynamically:**
Suppose you have a button on your web page, and you want to change its font color when a user clicks on it. Here’s an example using setComputedStyle:
“`javascript
const button = document.getElementById(‘myButton’);
button.addEventListener(‘click’, () => {
button.setComputedStyle(‘color’, ‘red’);
});
“`
In this case, when the button is clicked, the font color changes to red. You can customize the code to change the color dynamically based on user interactions or any other condition.
2. **Modifying background color with a fade effect:**
Imagine you have a div element with a solid background color. You want to change its background color gradually to another color, creating a fade effect. This can be achieved using setComputedStyle and a timer-based loop like this:
“`javascript
const div = document.getElementById(‘myDiv’);
const startColor = ‘red’;
const endColor = ‘blue’;
const duration = 2000; // Duration of the fade effect in milliseconds
let startTime;
function changeColor(timestamp) {
if (!startTime) startTime = timestamp;
const elapsed = timestamp – startTime;
const progress = Math.min(elapsed / duration, 1);
const r = Math.round(parseInt(startColor.substr(1, 2), 16) * (1 – progress) + parseInt(endColor.substr(1, 2), 16) * progress);
const g = Math.round(parseInt(startColor.substr(3, 2), 16) * (1 – progress) + parseInt(endColor.substr(3, 2), 16) * progress);
const b = Math.round(parseInt(startColor.substr(5, 2), 16) * (1 – progress) + parseInt(endColor.substr(5, 2), 16) * progress);
const color = `rgb(${r}, ${g}, ${b})`;
div.setComputedStyle(‘backgroundColor’, color);
if (progress < 1) {
window.requestAnimationFrame(changeColor);
}
}
div.addEventListener('click', () => {
startTime = null;
window.requestAnimationFrame(changeColor);
});
“`
In this example, when the div is clicked, the background color gradually transitions from red to blue over a duration of 2 seconds. This provides a visually appealing fade effect.
**Frequently Asked Questions (FAQs)**
1. **Can the setComputedStyle method modify styles added via CSS classes?**
Yes, the setComputedStyle method can modify any style property regardless of its origin. Therefore, it can change styles set via inline, internal, and external CSS classes.
2. **What happens if we try to change a non-existent property using setComputedStyle?**
If you attempt to change a non-existent property, the setComputedStyle method will simply ignore it. The function will not throw an error or cause any issues.
3. **Can we use setComputedStyle to change CSS keyframe animations?**
No, the setComputedStyle method is not suitable for modifying CSS keyframe animations. For animating CSS properties, it is recommended to use CSS transitions or animations directly.
4. **How does setComputedStyle differ from the style property?**
The style property is primarily used to modify inline styles, whereas setComputedStyle allows you to change styles regardless of their origin — inline, internal, or external CSS. Furthermore, setComputedStyle is more suitable for dynamic modifications and complex style manipulations.
5. **Does setComputedStyle work with pseudo-elements like ::before or ::after?**
Yes, setComputedStyle can be used to modify styles of pseudo-elements as well. You can easily select the respective pseudo-element and apply the desired style modifications using the method.
In conclusion, the setComputedStyle method provides a powerful tool for dynamically modifying styles of HTML elements using JavaScript. Its ability to change any style property regardless of its origin grants developers increased flexibility and control. By understanding the syntax and usage of setComputedStyle, developers can easily enhance the interactivity and visual appeal of their web pages.
Images related to the topic javascript style.left not working
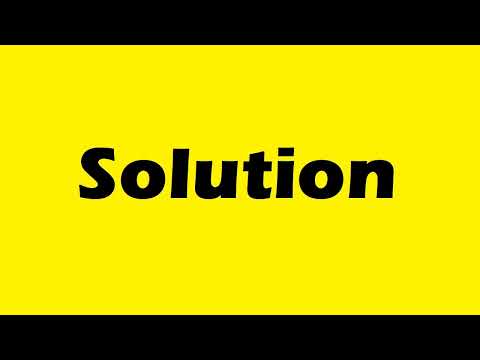
Found 35 images related to javascript style.left not working theme




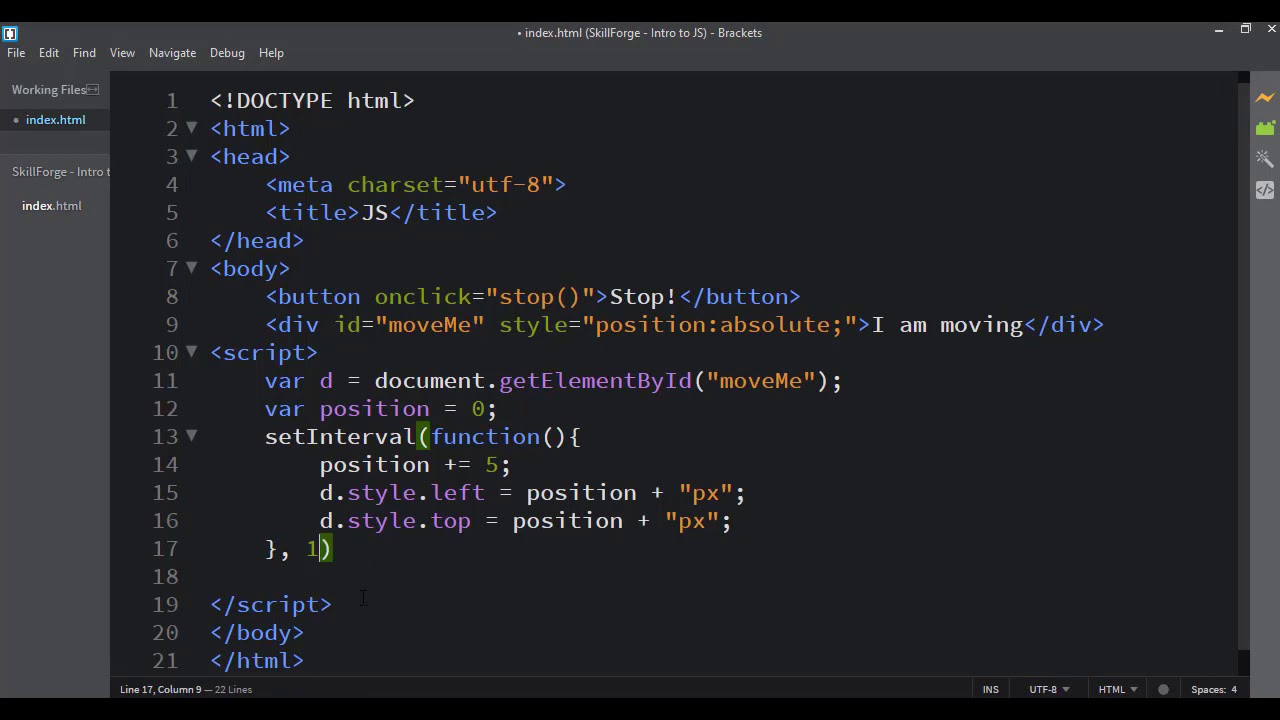

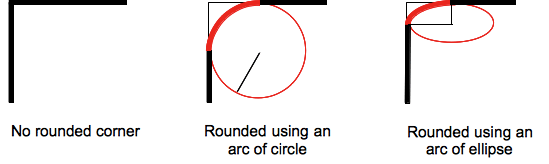
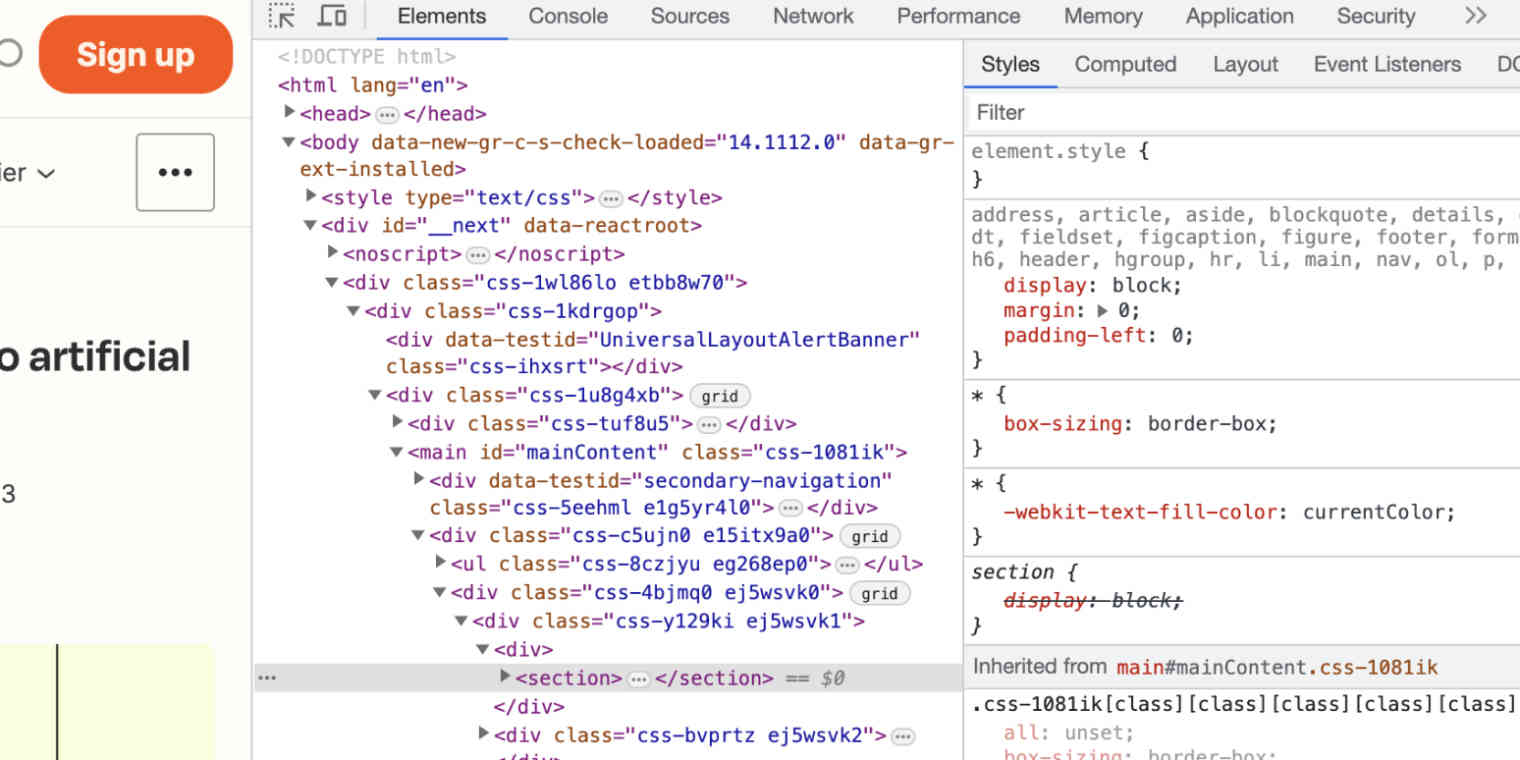
Article link: javascript style.left not working.
Learn more about the topic javascript style.left not working.
- style.top and style.left not working – javascript – Stack Overflow
- HTML DOM Style left Property – W3Schools
- style.left=”40%”; does not work in javascript – SitePoint
- How to set the left margin of an element with JavaScript
- HTML DOM Style left Property – W3Schools
- p5.js | style() Function – GeeksforGeeks
- How to set the top position of an element with JavaScript
- HTML | DOM Style left Property – GeeksforGeeks
- Javascript Reference – HTML DOM Style left Property
- ‘transition-duration’ right: 0; positioning does not work. Help?
- My element.style.top assignment is not working in Firefox – Bytes
See more: nhanvietluanvan.com/luat-hoc