Bash Date Invalid Date
1. Overview of the “Invalid Date” Error
The “Invalid Date” error is a common error message encountered when using the bash date command. It occurs when the input provided to the date command does not conform to the expected date and time format. This error can prevent the successful execution of scripts and commands that rely on accurate date and time information.
2. Common Causes of the “Invalid Date” Error
The “Invalid Date” error can be caused by several factors. One common cause is an incorrect date format provided as input to the date command. If the input does not match the expected format, the command will fail to interpret it as a valid date. Another cause could be incorrect system date and time settings, which can lead to the date command being unable to correctly parse the input.
3. How the Bash date Command Handles Invalid Dates
When the bash date command encounters an invalid date, it typically produces an error message containing the string “Invalid Date.” This error message serves as an indication that the input provided could not be interpreted as a valid date. It is then up to the user or the script to handle this error and find a resolution.
Troubleshooting the “Invalid Date” Error
1. Checking the Input Format for Validity
To troubleshoot the “Invalid Date” error, it is essential to ensure that the input provided to the date command is in the correct format. The date command expects date and time values in a specific format, such as “YYYY-MM-DD” for dates and “HH:MM:SS” for time. Verifying that the input adheres to these formats can help prevent the error.
2. Verifying the System Date and Time Settings
In some cases, the “Invalid Date” error may be caused by incorrect system date and time settings. To resolve this, it is necessary to verify that the system’s date and time settings are accurate. The date command relies on the system’s settings to interpret the input correctly. Adjusting the system’s date and time settings to the correct values can help resolve the error.
3. Handling Different Date Formats in Bash
Bash provides various tools and functions that can aid in handling different date formats. By utilizing these tools, it is possible to convert the input into a valid format before passing it to the date command. Functions like date parsing, date formatting, and date arithmetic can be used to manipulate the dates and ensure they are recognized correctly.
Resolving the “Invalid Date” Error
1. Modifying the Date Input to a Valid Format
If the input provided to the date command is in an incorrect format, it can be modified to match the expected format. This can be achieved by using string manipulation techniques or by utilizing tools like awk or sed to reformat the input. By converting the input to a valid format, the “Invalid Date” error can be resolved.
2. Using Date Manipulation Tools to Correct the Error
Bash provides several date manipulation tools, such as date arithmetic and date parsing functions, that can help correct the “Invalid Date” error. By performing date manipulation operations on the input, it is possible to transform it into a valid date and time representation. These tools can handle calculations like finding dates one day ago or one week ago, further aiding in resolving the error.
3. Writing Error Handling Scripts to Avoid the Issue
To prevent encountering the “Invalid Date” error, it is recommended to implement error handling scripts that validate input dates before execution. These scripts can check if the provided date is in a valid format and reject any input that does not conform to the expected format. By incorporating robust error handling strategies, scripts can avoid encountering the error altogether.
Preventing the “Invalid Date” Error
1. Validating Input Dates Before Execution
To prevent the “Invalid Date” error, it is crucial to validate input dates before executing any commands or scripts that rely on them. This can be done by implementing date validation mechanisms that check if the provided date adheres to the expected format. By rejecting invalid input upfront, the error can be avoided altogether.
2. Implementing Robust Error Handling Strategies
In addition to input validation, implementing robust error handling strategies is essential for preventing the “Invalid Date” error. Scripts should incorporate error handling mechanisms that can identify and handle any errors that may occur during the execution. By anticipating potential issues, scripts can gracefully handle errors and avoid encountering the “Invalid Date” error.
3. Utilizing Libraries or APIs to Simplify Date Handling
To simplify date handling and reduce the likelihood of encountering the “Invalid Date” error, it is recommended to utilize libraries or APIs that provide built-in date manipulation functionality. These libraries often offer comprehensive date parsing and formatting capabilities, allowing developers to focus on the logic of their scripts rather than low-level date manipulation operations.
FAQs
Q1. What does the “Invalid Date” error mean?
A1. The “Invalid Date” error occurs when the input provided to the bash date command does not conform to the expected date and time format. It indicates that the input could not be interpreted as a valid date.
Q2. How can I check if a date is valid in bash?
A2. To check if a date is valid in bash, you can use the date command with the -d option. For example, running the command “date -d ‘2022-13-01′” would result in the “Invalid Date” error if the date is not valid.
Q3. How do I format a date in bash?
A3. To format a date in bash, you can use the date command with the + option. For example, running the command “date +’%Y-%m-%d'” would display the current date in the format “YYYY-MM-DD”.
Q4. How do I calculate a date one week ago in bash?
A4. To calculate a date one week ago in bash, you can use the date command with the -d option and the “-7 days” argument. For example, running the command “date -d ‘7 days ago'” would display the date from one week ago.
Q5. How do I check if a date is between two dates in bash?
A5. To check if a date is between two dates in bash, you can convert the dates to Unix timestamps using the date command. You can then compare the timestamps using conditional statements to determine if the date falls within the specified range.
Q6. How do I check yesterday’s date in bash?
A6. To check yesterday’s date in bash, you can use the date command with the -d option and the “-1 day” argument. For example, running the command “date -d ‘yesterday'” would display yesterday’s date.
Q7. How do I parse a date in bash?
A7. To parse a date in bash, you can use the date command with the -d option and specify the date in a format that matches the input. For example, running the command “date -d ‘2022-01-01′” would parse the date “January 1, 2022.”
Q8. How do I convert a date to YYYYMMDDHHMMSS format in bash?
A8. To convert a date to the YYYYMMDDHHMMSS format in bash, you can use the date command with the + option and specify the desired format. For example, running the command “date +’%Y%m%d%H%M%S'” would result in the date in the format “20220101120000.”
In conclusion, understanding and troubleshooting the “Invalid Date” error in bash can help ensure the successful execution of scripts and commands that rely on accurate date and time information. By verifying input formats, checking system date and time settings, utilizing date manipulation tools, and implementing robust error handling strategies, it is possible to avoid and resolve this common error. Additionally, by validating input dates, implementing error handling scripts, and leveraging libraries or APIs, the likelihood of encountering the “Invalid Date” error can be significantly reduced.
Unix \U0026 Linux: Bash : Date -D Throws \”Invalid Date\” When Date Is Parameterized (2 Solutions!!)
How To Insert Date In Bash?
Bash (Bourne Again SHell) is the default command-line interpreter in most Linux distributions. It is a powerful scripting language that allows users to automate tasks and perform various operations. One common operation is inserting the date into a script or shell command. In this article, we will explore different methods and techniques to achieve this in bash.
Method 1: Using the `date` command
The most straightforward way to insert the current date into a bash script is by utilizing the `date` command. This command allows you to format the output according to your requirements. Here is an example:
“`bash
#!/bin/bash
current_date=$(date +”%Y-%m-%d”)
echo “Today’s date is: $current_date”
“`
In the above script, we use command substitution (`$(…)`) to store the output of the `date` command in the `current_date` variable. The format `%Y-%m-%d` represents the year, month, and day respectively. Feel free to modify the format to suit your needs.
Method 2: Using the `printf` command
Another way to insert the date in bash scripting is by using the `printf` command. This command enables you to format and print data. Consider the following script:
“`bash
#!/bin/bash
current_date=$(printf “%(%Y-%m-%d)T”)
echo “Today’s date is: $current_date”
“`
In this script, we utilize the `%(…)T` format specifier with `printf`. The `%(…)` syntax allows us to specify the format as an argument to `printf`. Again, you can modify the format as per your requirements.
Method 3: Using the backtick notation
The backtick notation (“`) is an older method for command substitution in bash. Although it is less preferred, it can still be used to insert the date into a script. Here’s an example:
“`bash
#!/bin/bash
current_date=`date +”%Y-%m-%d”`
echo “Today’s date is: $current_date”
“`
This script is similar to the first method, but we use backticks instead of `$(…)`. Keep in mind that backticks are considered obsolete, so it is better to use the `$(…)` syntax for command substitution.
FAQs:
Q1. Can I insert the date and time in my bash script?
A1. Yes, you can insert the date and time by modifying the format strings in the above examples. For example, `%H:%M:%S` represents hours, minutes, and seconds respectively. Combining different format strings allows you to display both the date and time.
Q2. How can I display the date in a different time zone?
A2. The `date` command provides the `-u` option to display the date and time in UTC (Coordinated Universal Time). You can also set the `TZ` environment variable to display the date in a specific time zone. For example, `TZ=America/New_York date` will display the date in the Eastern Time Zone.
Q3. How do I insert a custom date rather than the current date?
A3. To insert a specific date, you can pass it as an argument to the `date` command. For instance, `date -d “2021-12-25” +”%Y-%m-%d”` will display “2021-12-25”.
Q4. Can I insert the date in a different language?
A4. Yes, the `date` command supports different locales. You can use the `-l` option followed by the locale name to display the date in a specific language. For instance, `LANG=fr_FR.UTF-8 date -d “today” +”%A”` will display the current day in French.
In conclusion, inserting the date in a bash script is a common requirement for various tasks. Bash provides several methods, such as using the `date` command, `printf`, and backtick notation. Experiment with different formats and explore the options provided by the `date` command to suit your specific needs.
How To Get The Date In Bash?
Working with dates is an essential aspect of many scripting and programming languages. In the context of Bash, retrieving the current date and time, or manipulating dates in various formats, is a common requirement. Whether you need the information for logging, file naming, or any other purpose, understanding how to obtain and manipulate dates in Bash can prove to be highly useful. In this article, we will explore various methods to retrieve the date in Bash and showcase some practical examples.
Getting the Current Date and Time
To begin, let’s start with the most straightforward way to obtain the current date and time in Bash. By using the `date` command, you can easily retrieve the current date and time information. To achieve this, simply enter the following command in your terminal:
“`
date
“`
Executing this command will display the current date and time information, formatted according to your system’s default settings. However, the `date` command provides numerous formatting options to customize the output further. Let’s explore some examples:
1. To display the date in a specific format, utilize the `+%FORMAT` option with the `date` command. For instance, to display the date in the “YYYY-MM-DD” format, enter:
“`
date +’%Y-%m-%d’
“`
2. If you wish to retrieve only the year, month, or day individually, you can use `%Y`, `%m`, or `%d` respectively. For example, the following command will retrieve the current year:
“`
date +’%Y’
“`
3. Adding `%H`, `%M`, or `%S` after the `date` command will retrieve the current hour, minute, or second respectively. Here is how you would obtain the current hour:
“`
date +’%H’
“`
Manipulating Dates
The `date` command also allows us to manipulate dates by adding or subtracting days, months, or years. To do this, we use the `-d` option followed by a specific date. Here are some examples:
1. To retrieve the date of the day before today, run the following command:
“`
date -d “yesterday”
“`
2. You can also add or subtract a specific number of days, months, or years using numerical values. For instance, to find the date ten days from now:
“`
date -d “+10 days”
“`
3. Another useful feature is calculating the date and time difference between two given dates. Here’s an example of how to achieve this:
“`
date -d “2022-01-01” -d “2021-01-01”
“`
4. By combining the various formatting options with date manipulation, we can construct custom date formats. For example, to obtain yesterday’s date in the “YYYY/MM/DD” format:
“`
date -d ‘yesterday’ +’%Y/%m/%d’
“`
FAQs
Q: Can I change the default date format in Bash?
A: Yes, you can modify the default date format in Bash. To do this, you need to modify the environment variable called `LANG`. For example, you can set `LANG` to `en_GB` to adopt the British date format. Alternatively, you can customize the formatting options used with the `date` command to achieve the desired output.
Q: How can I store the current date in a variable?
A: You can store the current date in a variable using command substitution in Bash. Here’s an example:
“`
current_date=$(date +’%Y-%m-%d’)
“`
Now, the value of the `current_date` variable will hold the current date in the “YYYY-MM-DD” format.
Q: Can I retrieve the day of the week using the `date` command?
A: Absolutely! By using the `%A` formatting option with the `date` command, you can get the full name of the day whereas `%a` will give you the abbreviated name of the day.
Q: How do I retrieve the date and time in a specific timezone?
A: The `date` command provides the `-u` option to retrieve the date and time in UTC (Coordinated Universal Time). Additionally, you can set the `TZ` variable to a specific timezone to get the date and time in that particular timezone.
In conclusion, obtaining and manipulating dates in Bash can be easily achieved using the `date` command. By incorporating various formatting options and date calculations, you can retrieve dates in different styles and perform custom operations. The flexibility and simplicity of Bash make it a powerful tool for working with dates in a scripting environment.
Keywords searched by users: bash date invalid date bash date 1 day, bash check if date is valid, bash date parse, bash date format, bash date 1 week ago, bash yesterday date, check if date is between two dates bash, linux date yyyymmddhhmmss
Categories: Top 21 Bash Date Invalid Date
See more here: nhanvietluanvan.com
Bash Date 1 Day
Introduction:
Bash scripting is an essential skill for any Linux system administrator or developer. One common task that often arises is manipulating dates. Whether you need to calculate a future or past date, extract specific parts of a date, or format dates for display, the “date” command in Bash is your go-to tool. In this article, we will explore the versatile “date” command and cover its various options and usage scenarios.
Understanding the “date” command:
The “date” command in Bash allows you to display or manipulate the current date and time. To get started, open your terminal and enter “date” followed by hitting the “Enter” key. You will see the current system date and time displayed in a default format. However, the true power of the “date” command lies in its ability to accept numerous options for customizing the output.
Basics of manipulating dates in Bash:
To manipulate dates in Bash, we primarily rely on the date arithmetic feature of the “date” command. By using arithmetic operators (+, -) along with numeric values, we can perform various operations on dates.
For example, to calculate the date one day ahead of the current date, we can use the following command:
“`
date -d “+1 day”
“`
This command instructs the “date” command to add one day (“-d “+1 day””) to the current date. Depending on when and where you run this command, it will display the date in your local timezone.
Using relative values with “date” command:
In addition to using numeric values for date manipulation, the “date” command also allows us to use relative values such as “yesterday”, “tomorrow”, or “next Sunday”. This feature is particularly useful when you do not want to perform specific date calculations.
For instance, to obtain the date one day prior to the current date, you can use the following command:
“`
date -d yesterday
“`
The “yesterday” keyword instructs the “date” command to deduct one day from the current date.
Manipulating specific parts of a date:
The “date” command supports a variety of format options to extract specific parts of a date. Some common formats include day, month, year, hour, minute, and second. By combining these format options with the current date, we can obtain any specific date part.
For example, to extract the current year, we use the “%Y” format option. The following command will display the current year:
“`
date +%Y
“`
Frequently Asked Questions (FAQs):
Q1. Can I change the format in which the date is displayed?
Absolutely! The “date” command provides a flexible way to format the date output. By using format options preceded by a “%” sign, you can customize the output. For example, to display the current date in YYYY-MM-DD format, use the command “date +%Y-%m-%d”.
Q2. How can I calculate the date one week from now?
You can achieve this by using the “date” command with the “+7 days” argument. Here’s the command:
“`
date -d “+7 days”
“`
Q3. Is it possible to display the date in a different timezone?
Yes, you can specify the timezone using the “+%Z” format option. For example, to display the current date and time in the Pacific Time Zone, use the command “TZ=America/Los_Angeles date”.
Q4. Can I perform date calculations with specific dates instead of the current date?
Certainly! By modifying the reference date using the “-d” option, you can perform date arithmetic with specific dates. For example, to calculate the same day next month, use the following command:
“`
date -d “2022-08-12 +1 month”
“`
Q5. How can I display the date in a different language?
You can change the locale to display dates in a different language. Set the “LC_TIME” environment variable to the desired locale, and the “date” command will display the date in that language. For example, to display the date in French, use the command:
“`
LC_TIME=fr_FR.UTF-8 date
“`
Conclusion:
Manipulating dates in bash scripting is a crucial skill for Linux system administrators and developers. The “date” command provides a powerful toolset for performing various date calculations and formatting. By understanding the options available and combining them creatively, you can achieve precise date manipulations tailored to your specific needs. With the knowledge gained from this article, you now have a solid foundation to handle dates effectively in your bash scripts.
Bash Check If Date Is Valid
One of the key functionalities that Bash scripting offers is the ability to validate various types of data, including dates. Validating dates is particularly important when dealing with user input or when processing data that relies on the correct representation of date values. In this article, we will explore how to check if a date is valid using Bash scripting, discuss common challenges, and provide practical examples for you to follow along.
Understanding Date Formats:
Before diving into the specifics of Bash date validation, it’s crucial to understand the different date formats commonly used.
1. Standard Date Format (YYYY-MM-DD): The standard format for representing dates is “YYYY-MM-DD,” where YYYY represents the four-digit year, MM represents the two-digit month (ranging from 01 to 12), and DD represents the two-digit day (ranging from 01 to 31).
2. Custom Date Formats: Apart from the standard format, dates can also be represented in various custom formats, such as “MM/DD/YYYY” or “DD-MM-YY”. It’s important to consider these formats while validating dates to ensure comprehensive verification.
Let’s now explore how to check if a date is valid using Bash scripting.
Bash Date Validation:
To validate a date, we can utilize two approaches: using the date command and performing manual checks within our script. Let’s examine both methods.
1. Using the date Command:
Bash offers the date command, which can be used to parse and format dates. By attempting to format a given date according to a specific format, we can determine whether it’s valid. Consider the following example:
“`bash
#!/bin/bash
input_date=”2022-06-15″
if [ “$(date -d $input_date +%Y-%m-%d 2>/dev/null)” == “$input_date” ]; then
echo “Date is valid.”
else
echo “Date is invalid.”
fi
“`
In the above code snippet, we assign a date “2022-06-15” to the input_date variable. Then, we use the date command to format the input_date according to the “%Y-%m-%d” format. If the formatted date matches the original input_date, we conclude that it’s valid; otherwise, it’s considered invalid.
2. Manual Date Validation:
Another approach is to manually validate the date components. This method is particularly useful if we need to support various date formats. Consider the following example:
“`bash
#!/bin/bash
input_date=”20-06-22″
IFS=’-‘ read -r -a date_array <<<"$input_date" day=${date_array[0]} month=${date_array[1]} year=${date_array[2]} if [ ${#day} -eq 2 ] && [ ${#month} -eq 2 ] && [ ${#year} -eq 2 ]; then if ((day >= 1 && day <= 31)) && ((month >= 1 && month <= 12)) && ((year >= 00 && year <= 99)); then echo "Date is valid." else echo "Date is invalid." fi else echo "Date is invalid." fi ``` In this example, we split the input_date using the "-" delimiter and store the individual day, month, and year components in the respective variables. We then perform manual checks on the length and range of these components to validate the date. Common FAQs: Q1. Can I validate the current system date using Bash scripting? Yes, you can validate the current system date using any of the approaches mentioned above. Simply assign the output of the date command (which represents the current date) to the input_date variable and apply the validation logic accordingly. Q2. How can I check if a date is within a specific range? To check if a date falls within a specific range, you can modify the validation logic accordingly. For instance, within the manual validation approach, you can introduce additional checks to ensure the date falls within the desired range. Q3. Can I validate dates in a non-English locale using Bash? Yes, the date command supports various locale settings, allowing you to validate dates in different languages. Use the "LANG" environment variable to set the desired locale, such as "LANG=fr_FR" for French. However, be cautious when using varying date formats specific to different locales. In conclusion, validating dates in Bash scripting is crucial to ensure data integrity and accuracy. By utilizing the date command or performing manual checks, you can effectively determine whether a given date is valid. Understanding date formats and considering different scenarios, such as custom formats or date ranges, will further enhance your Bash scripting capabilities.
Images related to the topic bash date invalid date
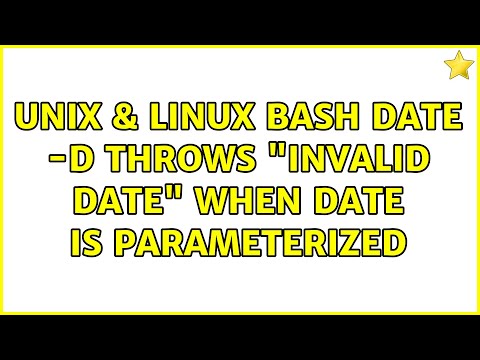
Found 28 images related to bash date invalid date theme
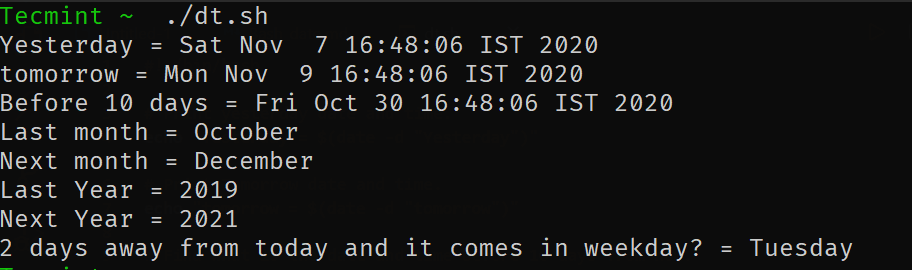
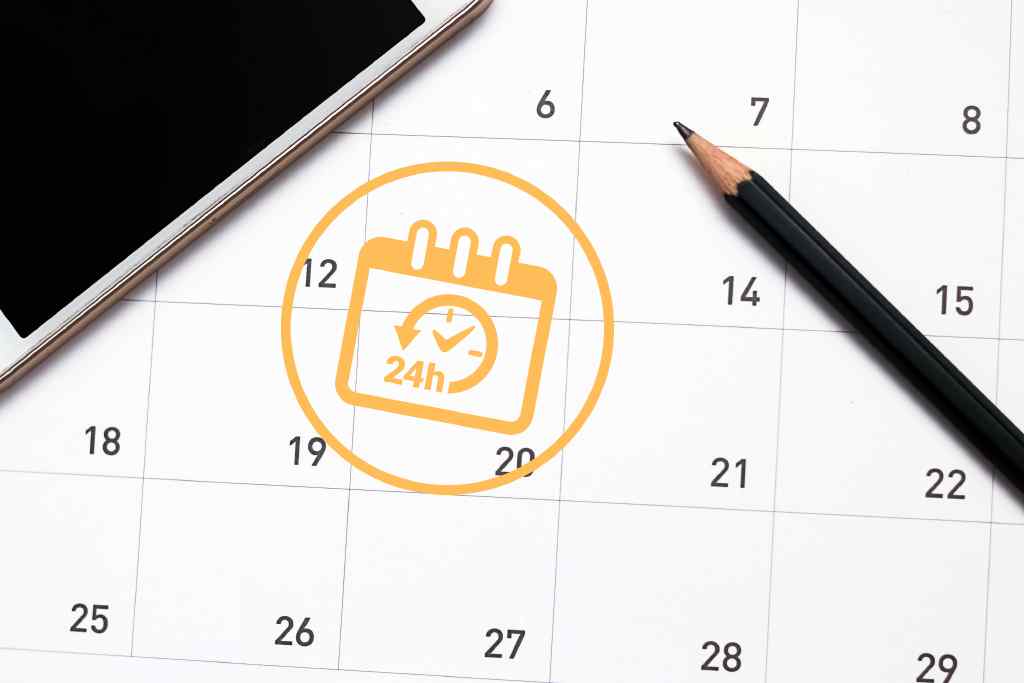
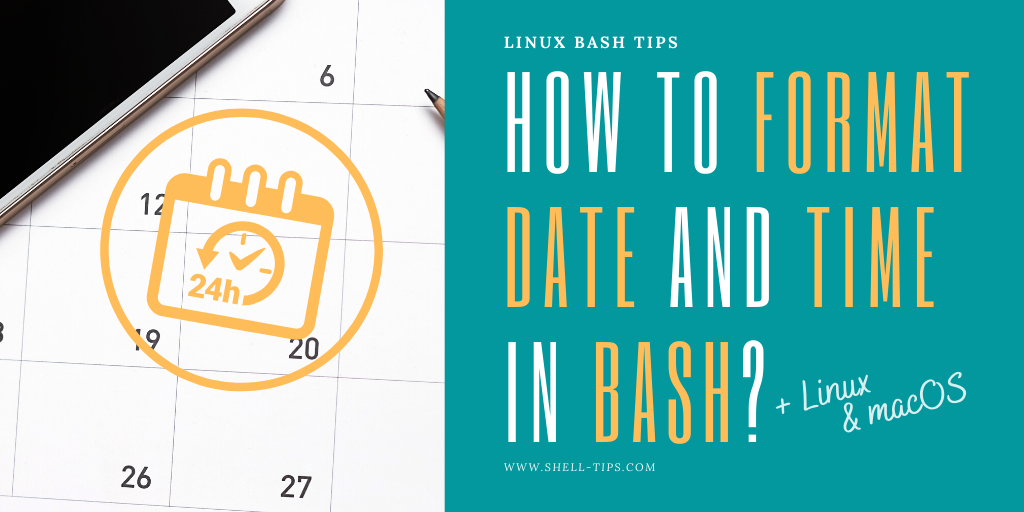
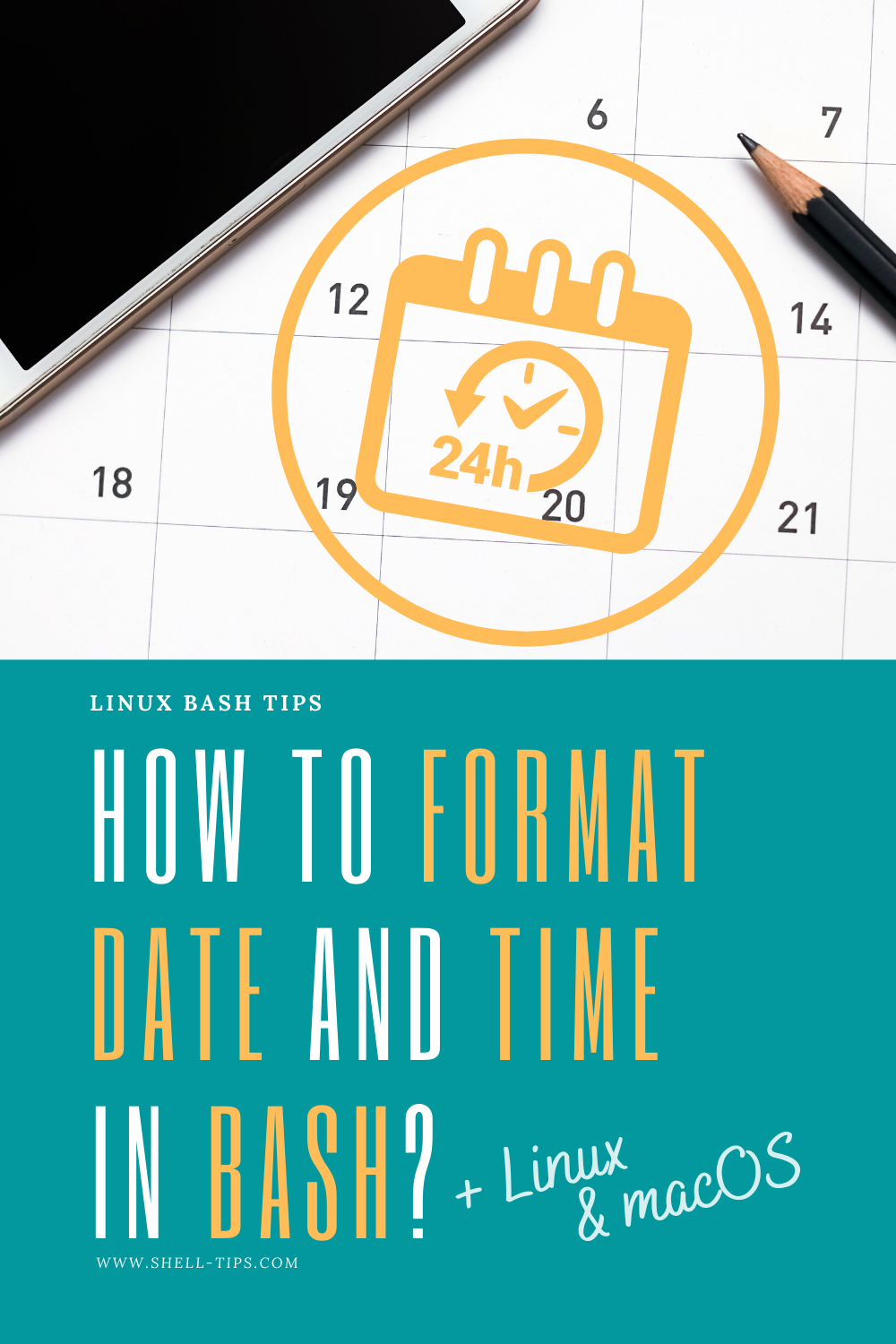
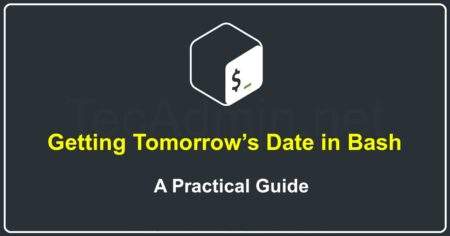

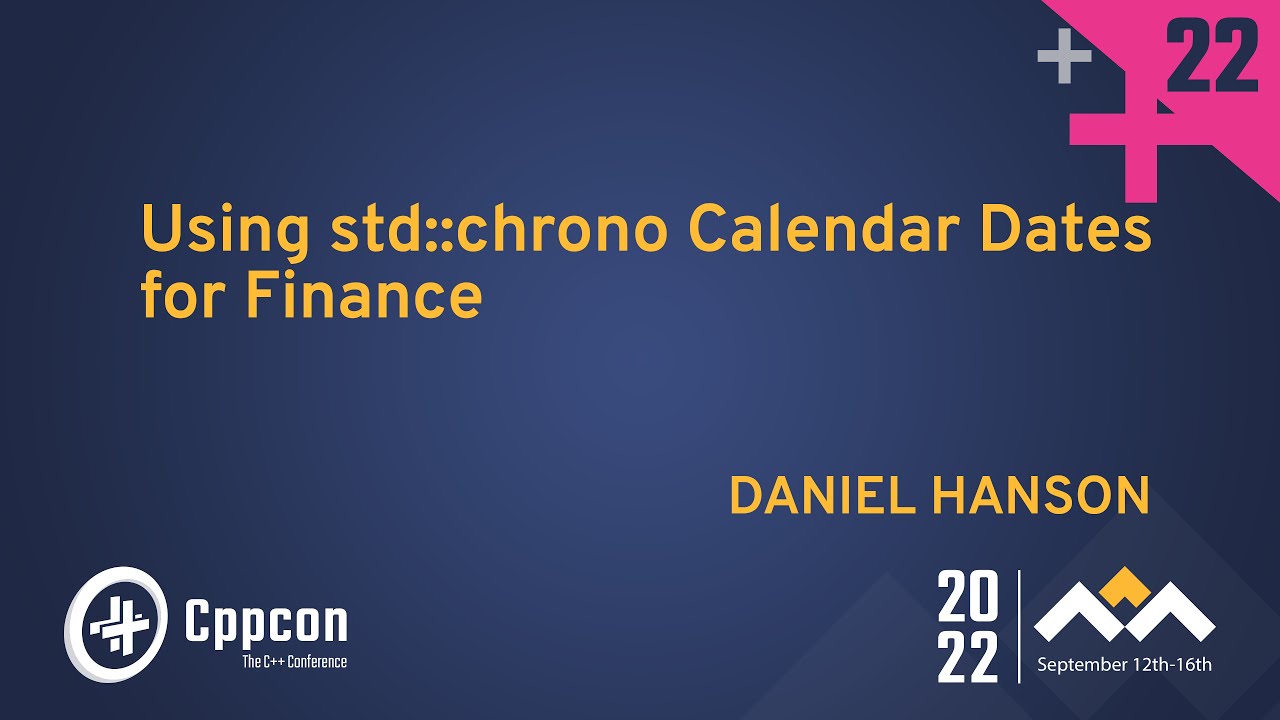
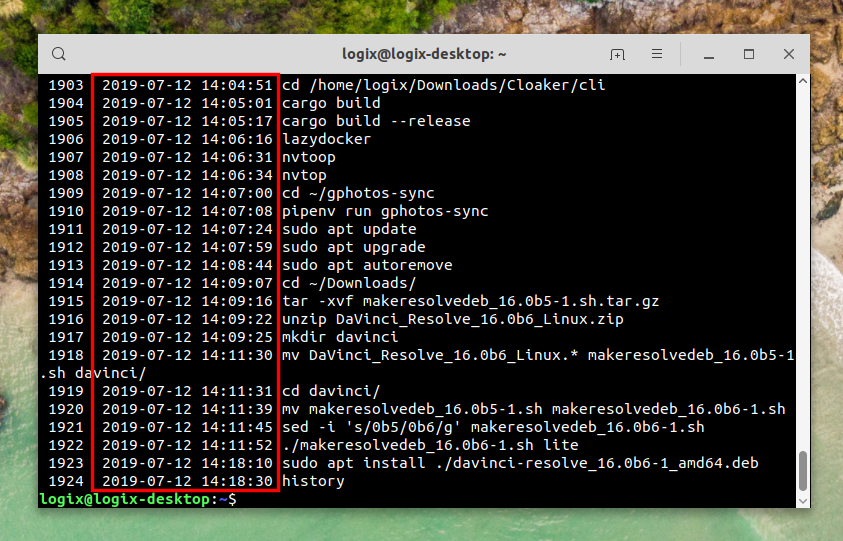
Article link: bash date invalid date.
Learn more about the topic bash date invalid date.
- Bash : date -d throws “invalid date” when date is parameterized
- Date: invalid date trying to set Linux date in specific format
- bash “date” returns “invalid date” error for a specific date string
- Invalid date error by date command – Ask Ubuntu
- How To Format Date and Time in Linux, macOS, and Bash?
- How To Get / Print Current Date in Unix / Linux Shell Script – nixCraft
- How to Get Current Date and Time in Bash Script – TecAdmin
- About a bash date command – DiskInternals
- Bash “1 day ago” results in invalid date – Home Assistant OS
- Bash shell – date: invalid date -d for string in another time zone
- Edgerouter – Adding x minutes to current time
- invalid date ‘+%s’ · Issue #131 · lucascbeyeler/zmbackup
- How to Check Whether Date Argument Is in the yyyy-mm-dd …
- date returns “invalid date” for some timezone’s DST
See more: nhanvietluanvan.com/luat-hoc