Eoferror Ran Out Of Input
This error can be encountered in various situations, such as reading input from a file, network stream, or even user input. When the end of the input stream is reached unexpectedly, the program raises an EOFError.
Causes of EOFError:
1. Insufficient input data: One of the main reasons for encountering an EOFError is when the input data provided to the program is not sufficient. If the program tries to read more input than actually exists, it leads to the end of file error.
2. Unexpected termination of the input stream: Another cause could be the abrupt termination of the input stream. For example, if a file being read suddenly gets deleted or becomes inaccessible, it will result in an EOFError.
3. Incorrect input format: If the data being read from a stream is not in the expected format or does not adhere to the predefined structure, it can lead to an end of file error. In such cases, the program may not be able to process the input correctly and hence raises an EOFError.
Handling EOFError:
1. Using try-except statement: The most common way to handle an EOFError is by using a try-except statement. By wrapping the code that reads input with a try block and catching the EOFError in the except block, you can handle the exception gracefully. This allows you to handle the error condition and prevent the program from crashing.
“`python
try:
# Code to read input
except EOFError:
# Handle the EOFError
“`
2. Using a while loop to process input: Another approach is to use a while loop to continuously read input until the end of the input stream is reached. By checking for the end of file condition within the loop, you can ensure that the program does not try to read beyond the available data.
“`python
while True:
try:
# Code to read input
except EOFError:
# Handle the EOFError
break
“`
Tips to avoid EOFError:
1. Check input data beforehand: Before reading input from a stream, it is important to check the availability and sufficiency of the input data. By checking the length or size of the input data, you can avoid encountering an EOFError.
2. Implement error handling mechanisms: To handle unexpected termination of input streams or incorrect input formats, it is essential to implement proper error handling mechanisms. This can include validating the input data before processing it and handling any errors that may occur during the reading process.
FAQs:
Q: What is Ran out of input Pytorch?
A: “Ran out of input Pytorch” is not a specific error message, but it could be a general description indicating that the program encountered an EOFError while reading input in a Pytorch framework.
Q: What is EOFError: EOF when reading a line?
A: This error message indicates that an EOFError occurred when the program was trying to read a line of input. It suggests that the end of the input stream was reached unexpectedly, before the program could read a complete line.
Q: What is EOFError trong Python?
A: “EOFError trong Python” translates to “EOFError in Python” in English. It refers to the end of file error encountered in Python while reading input from a stream.
Q: How to read a pickle file in Python?
A: To read a pickle file in Python, you can use the `pickle` module. The `pickle.load()` function is commonly used to load data from a pickle file into a Python object.
Q: What to do when a Pickle load empty file error occurs?
A: If you encounter a “Pickle load empty file” error, it means that the pickle file you are trying to load is empty or does not contain any data. Make sure the file exists and contains valid pickle data before attempting to load it.
Q: What is “AttributeError: can’t pickle local object ‘__init__.
A: This error commonly occurs when trying to pickle a lambda function defined inside a class’s `__init__` method. To resolve this issue, avoid pickling local objects within the class or move the lambda function to a global scope.
Q: Can we append to a pickle file?
A: No, you can’t directly append to a pickle file. Pickle files are serialized objects, and appending data to them can cause corruption. To add more data, you should load the pickle file, modify the data structure, and then save it back to a new pickle file.
Q: What does “io.UnsupportedOperation: read eof” mean?
A: This error indicates that an input stream encountered EOF (end of file) unexpectedly while trying to read data. It often occurs when reading from a closed or inaccessible file or when there is no more data available to read.
In conclusion, encountering EOFError, or “ran out of input,” is a common occurrence in programming, indicating that a program attempted to read more input than was available. By understanding the causes and handling techniques discussed above, developers can effectively manage and prevent EOFErrors in their programs.
Python :Why Do I Get \”Pickle – Eoferror: Ran Out Of Input\” Reading An Empty File?(5Solution)
Keywords searched by users: eoferror ran out of input Ran out of input Pytorch, EOFError: EOF when reading a line, EOFError trong Python, Read pickle file Python, Pickle load empty file, Attributeerror can t pickle local object trainaugmentation __init__ locals lambda, Pickle append to file, io.unsupportedoperation: read
Categories: Top 43 Eoferror Ran Out Of Input
See more here: nhanvietluanvan.com
Ran Out Of Input Pytorch
Introduction (110 words):
PyTorch, a popular deep learning framework, enables the implementation of various complex machine learning models. However, as with any software, developers may encounter errors or exceptions during code execution. One such issue is the “Ran out of input PyTorch” error. In this article, we will delve into this error, understand its causes, and explore potential solutions to mitigate it. By delving into this error in depth, we hope to equip developers with a comprehensive understanding of the issue and empower them to resolve it effectively.
Understanding the “Ran out of input PyTorch” Error (250 words):
The “Ran out of input PyTorch” error usually occurs when you attempt to iterate beyond the bounds of your data. This error can be disconcerting, especially when you believe your input data is readily available. To effectively tackle this error, it is vital to understand its potential sources:
1. Insufficient Data: One common cause of this error is an insufficient amount of data provided for processing or training. It could be that the dataset is smaller than expected, resulting in the model running out of data to process or learn from.
2. Improper Data Loading: In PyTorch, data is typically loaded into the model using a DataLoader or Dataset. If there are issues with the implementation of these components, such as incorrect indexing or an overlooked end condition, the model can inadvertently run out of input.
3. Training Loop Mistakes: Another possibility is that the error arises due to a mistake or bug in the training or evaluation loop. For example, an incorrect loop termination condition or iteration counter management could lead to the model trying to access nonexistent data.
Troubleshooting the “Ran out of input PyTorch” Error (370 words):
Now that we’ve identified potential sources of this error, let’s explore troubleshooting techniques to address them effectively:
1. Check Data Availability: Begin by ensuring your dataset has sufficient data for processing or training. Verify the dimensions and size of your dataset, ensuring it matches the expected input shape for your model. If the dataset is indeed smaller, consider augmenting it with appropriate techniques like data synthesis or collecting more data.
2. Review Data Loading Implementation: Examine the code responsible for loading data into your PyTorch model. Carefully review the indexing logic, validating that it covers the entire dataset appropriately. Double-check the correctness of loop termination conditions and any implemented shuffling or batching techniques.
3. Debugging Training Loop: Inspect the training or evaluation loop code, focusing on the iteration counter management and loop termination conditions. Ensure that the loop increments the counter correctly and terminates when it reaches the expected number of iterations. Diagnostic print statements or debuggers can be valuable tools for discovering any anomalies in the loop execution.
4. Utilize Debugger Tools: PyTorch provides a powerful debugging tool called “torch.autograd.set_detect_anomaly(True),” which assists in locating the exact problematic line of code leading to the error. Enable this tool to gain insights into potential errors related to gradients, calculations, or model parameters.
5. Seek Community Support: If none of the troubleshooting steps yield satisfactory results, it may be helpful to seek assistance from PyTorch’s community forums or platforms like Stack Overflow. Share the relevant code snippets and explain the specific context in which the error occurs. The PyTorch community is renowned for its helpfulness and expertise, and they can provide insights or solutions to your particular issue.
FAQs (112 words):
Q1. Is the “Ran out of input PyTorch” error common in PyTorch?
A1. While not the most common error, it can occur. Vigilant data handling and loop management can usually prevent this issue.
Q2. How can I prevent running out of input?
A2. Ensure sufficient data, verify data loading code correctness, double-check loop implementation, and use PyTorch debugging tools.
Q3. The error still persists after following the recommended steps. What should I do?
A3. Reach out to the PyTorch community for assistance. Share relevant code snippets, the input size, and context to help community members better understand your problem.
Q4. Can this error occur during inference as well?
A4. While it primarily affects training and evaluation loops, this error can also arise during inference if input data handling is flawed or inconsistent.
Conclusion (30 words):
The “Ran out of input PyTorch” error can be resolved by thoroughly examining the code responsible for data loading, manipulating loops, and utilizing PyTorch’s debugging tools.
Eoferror: Eof When Reading A Line
In the world of programming, errors are inevitable but also hold immense value. They serve as a reminder to developers that there are potential flaws in the code that need to be addressed. One such error that programmers frequently encounter is the EOFError, specifically when reading a line. This article aims to shed light on this error, its causes, and potential solutions.
What is EOFError: EOF when reading a line?
EOFError: EOF when reading a line is an error that occurs when attempting to read from an input stream, such as a file or the standard input (stdin), and encountering an unexpected end of file (EOF) before a complete line is read. This error commonly occurs in programming languages like Python, Ruby, and Java, but its concept can be found in various other languages as well.
Causes of EOFError: EOF when reading a line:
1. Incomplete or corrupted file: One of the main causes of this error is an incomplete or corrupt file. If the input stream being read contains missing or invalid data, it can lead to encountering an unexpected end of file while trying to read a line.
2. Input mismatch: When the expected input format doesn’t match the actual input being read, an EOFError may occur. This can happen if the program is designed to read specific data types, such as integers or strings, but receives a different type of input.
3. End of input stream: Another cause of this error is reaching the end of the input stream before a complete line is read. If there are no more lines or data to be read, the program may throw an EOFError.
Common scenarios where EOFError occurs:
1. Reading from a file: When reading data from a file, programmers often use methods like `readline()` or `readlines()` to consume lines of text. If an unexpected end of file is encountered before a line is fully read, an EOFError is raised.
2. Input via the command line: In languages that support reading input from the standard input, such as Python’s `input()` function, encountering an end of file symbol, typically signaled by pressing Ctrl+D (Unix-based systems) or Ctrl+Z (Windows), could lead to an EOFError when reading a line by line.
Solutions to EOFError: EOF when reading a line:
1. Check file integrity: When working with files, it is crucial to ensure file integrity. You should verify whether the file is complete, not corrupted, or if any additional error handling is required to handle such scenarios.
2. Validate input: To prevent an EOFError caused by an input mismatch, it is essential to validate and sanitize user input. Input validation can include checking for expected data types, range checks, or using regular expressions to enforce specific formats.
3. Handle EOF gracefully: Instead of raising an exception, you can handle the EOF condition gracefully to avoid unexpected program termination. This can be done by catching the EOFError exception and handling it appropriately within the code.
4. Use try-except blocks: Surrounding the code that reads input with try-except blocks allows you to catch the EOFError and handle it properly. By employing try-except blocks, you can gracefully handle this error and provide appropriate feedback to the user.
FAQs:
Q: How do I know if EOFError is the cause of my issue?
A: When encountering an unexpected end of file while reading a line, the program will raise an EOFError exception. The error message typically indicates that an EOFError occurred while reading from the input stream.
Q: Can an EOFError occur while reading binary files?
A: Yes, an EOFError can occur when reading binary files as well. The general concept of encountering an unexpected end of file remains the same, regardless of the type of file being read.
Q: Is it possible to recover from an EOFError?
A: It is challenging to recover from an EOFError without appropriate handling. However, by using try-except blocks and providing proper feedback or error messages to users, you can mitigate its impact and ensure a smoother user experience.
Q: Can an EOFError be prevented altogether?
A: While EOFErrors cannot be completely prevented, careful validation of input, error handling, and ensuring file integrity can significantly reduce the chances of encountering this error.
Q: Are there any tools or libraries available to handle EOFErrors?
A: Yes, many programming languages offer built-in error handling mechanisms, such as try-except blocks, that can be used to handle EOFErrors. Additionally, there are libraries and frameworks available that provide more advanced error handling and input validation functionalities.
Conclusion:
EOFError: EOF when reading a line is a common error encountered by programmers while reading input from files or the standard input stream. By understanding its causes and implementing appropriate solutions, developers can handle this error gracefully, preventing unexpected program termination and ensuring a smoother user experience. Remember to validate input, handle the end of file condition gracefully, and make use of error-handling mechanisms to effectively deal with EOFErrors.
Eoferror Trong Python
Python is a versatile programming language that offers a range of exceptional features, making it a popular choice among developers. However, like any other programming language, Python also has its own set of errors and exceptions that programmers may encounter during development. One such error is the EOFError, which stands for End-of-File Error.
In this article, we will delve into the details of the EOFError in Python, understand its causes, and explore ways to handle it effectively. So, let’s begin by understanding what EOFError actually means.
What is EOFError in Python?
EOFError is an exception raised in Python when built-in functions like `input()` or `raw_input()` encounter the end of a file (EOF) condition without receiving the expected input from the user. Essentially, it occurs when there is an unexpected end of data while the input stream is being parsed.
Causes of EOFError
This error typically occurs when the `input()` function is used to obtain user input, and the user terminates the input stream by sending an end-of-file character (EOF). The specific key combination used to send the EOF character varies depending on the operating system. In Unix-like systems, including macOS and Linux, the EOF character can be sent by pressing `Ctrl+D` in the terminal. On Windows, the combination `Ctrl+Z` followed by pressing Enter signifies the end of a file.
The occurrence of EOFError can often be a result of users accidentally pressing these key combinations while entering input. It is important to note that EOFError is not a common error and is generally encountered during interactive user inputs rather than in script-based development.
Handling EOFError
To handle the End-of-File Error in Python, we can use try-except blocks to catch the exception and handle it gracefully. Let’s take a look at an example:
“`python
try:
user_input = input(“Enter something: “)
except EOFError:
print(“End of file reached unexpectedly.”)
“`
In the above code snippet, we use a `try-except` block to catch the `EOFError` exception. If the user accidentally triggers the EOF condition while providing input, the code within the `except EOFError:` block will be executed, and a custom error message will be printed to the console.
However, it is essential to remember that EOFError should not be confused with the simplest way to signal the end of file while reading from a file. In that context, Python uses another exception known as `StopIteration` to signify the end of file.
Frequently Asked Questions (FAQs):
Q1. Can EOFError occur in file operations?
A1. No, EOFError is specific to functions that read user input from the console. When reading from files, Python handles the end-of-file condition differently and raises a `StopIteration` exception instead.
Q2. How can I prevent EOFError when asking for user input?
A2. As a developer, you cannot completely prevent users from accidentally triggering EOF. However, you can inform users about the correct way to exit the input stream or consider a different approach that does not involve the input() function altogether.
Q3. Are EOFError and KeyboardInterrupt the same?
A3. No, EOFError and KeyboardInterrupt are two different exceptions in Python. While EOFError is raised when an end-of-file condition is encountered, KeyboardInterrupt occurs when a user interrupts the execution of a program by pressing `Ctrl+C` on the keyboard.
Q4. Can I catch multiple exceptions including EOFError?
A4. Yes, you can catch multiple exceptions using a single `try` block by separating different exceptions with commas. For example:
“`python
try:
# Code that may raise different exceptions
except (EOFError, ValueError):
# Handling both EOFError and ValueError
“`
Conclusion
In conclusion, EOFError is an exceptional circumstance in Python that occurs when the end of a file is reached unexpectedly while parsing user input. It is not a common error but can be encountered during interactive user input scenarios. By using try-except blocks, developers can gracefully handle the EOFError and prevent their programs from crashing unexpectedly. Understanding these nuances of Python’s EOFError will help you become a more skillful and confident developer. Happy coding!
Images related to the topic eoferror ran out of input
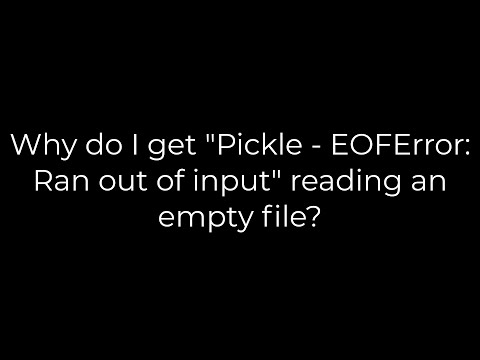
Found 11 images related to eoferror ran out of input theme




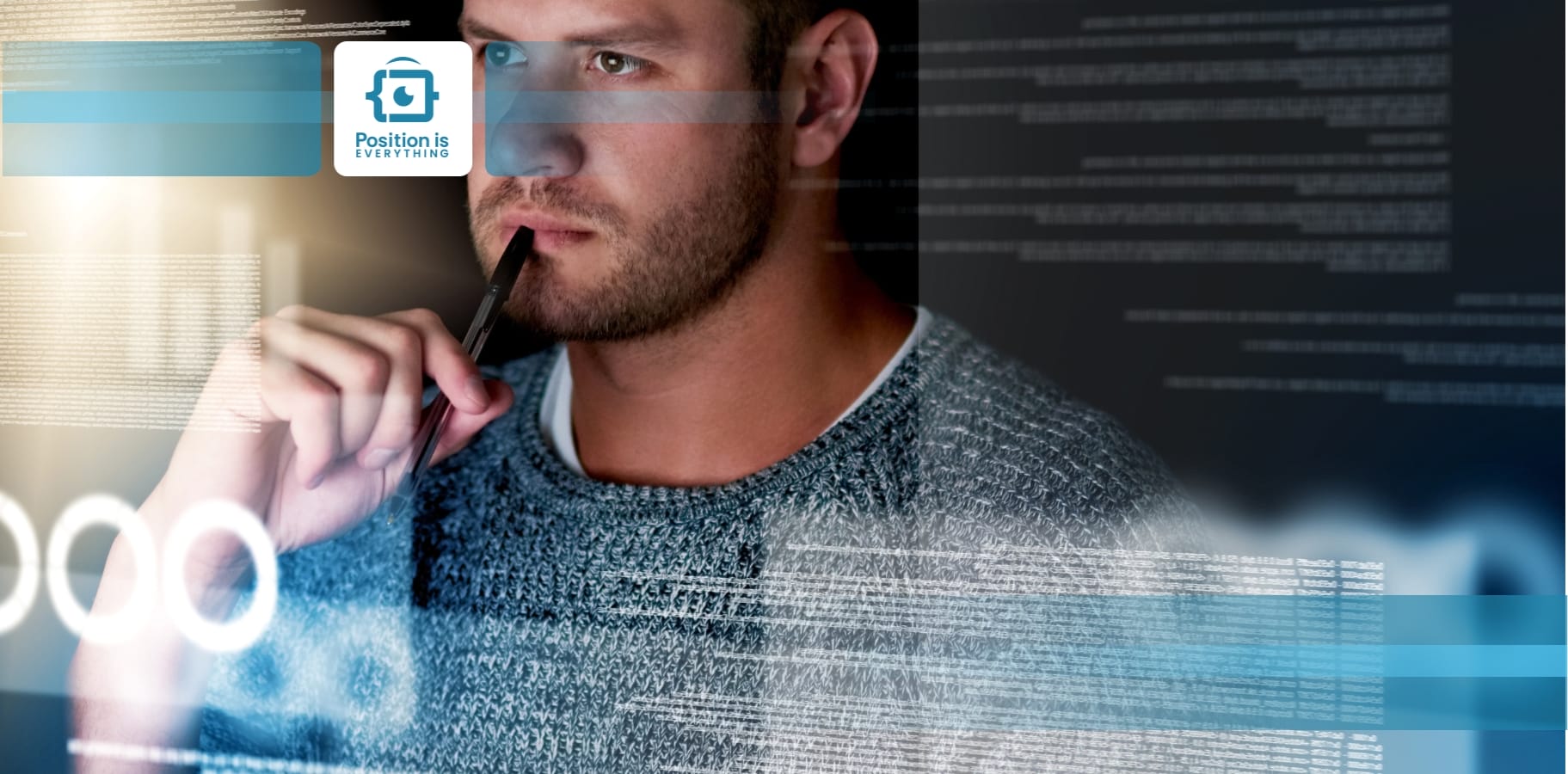

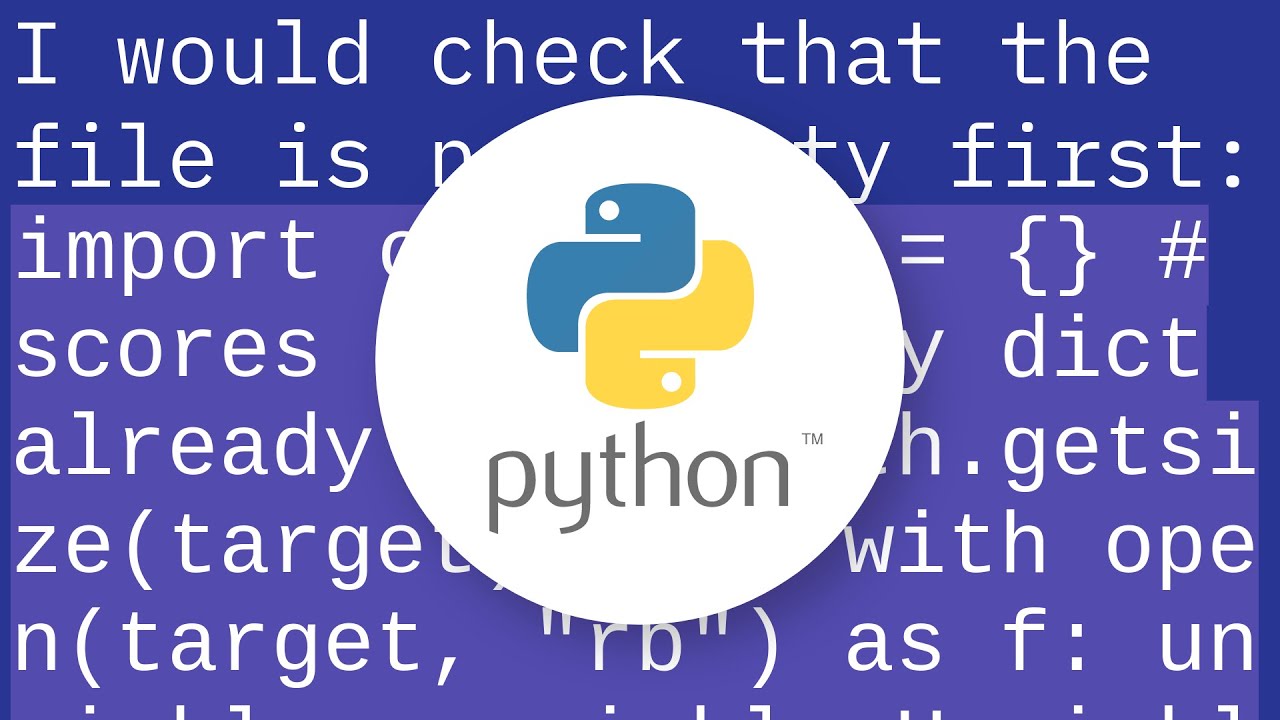

![python报错(pytorch)]——EOFError: Ran out of input_python eoferror: ran out of input_Star星屹程序设计的博客-CSDN博客 Python报错(Pytorch)]——Eoferror: Ran Out Of Input_Python Eoferror: Ran Out Of Input_Star星屹程序设计的博客-Csdn博客](https://img-blog.csdnimg.cn/20210718190122298.png?x-oss-process=image/watermark,type_ZmFuZ3poZW5naGVpdGk,shadow_10,text_aHR0cHM6Ly9ibG9nLmNzZG4ubmV0L3dlaXhpbl80MjA2Nzg3Mw==,size_16,color_FFFFFF,t_70)
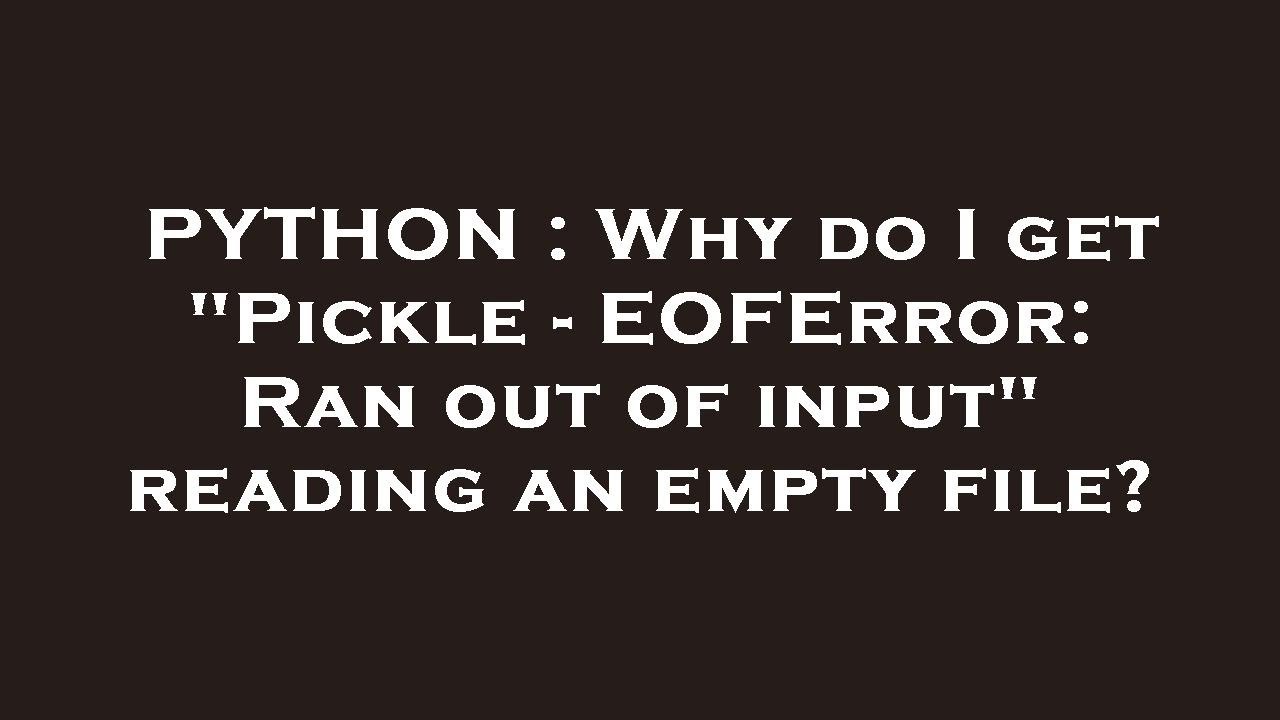
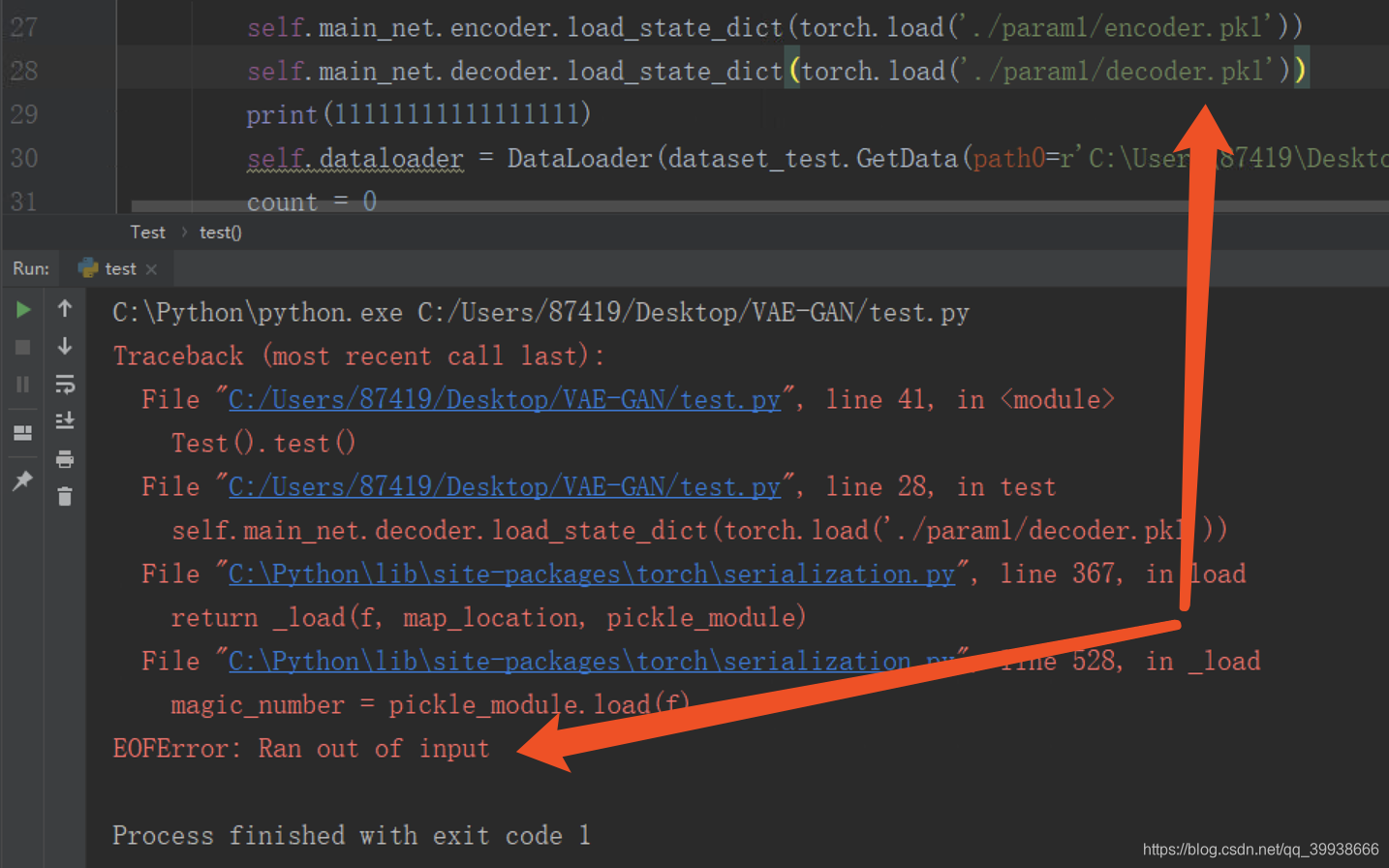

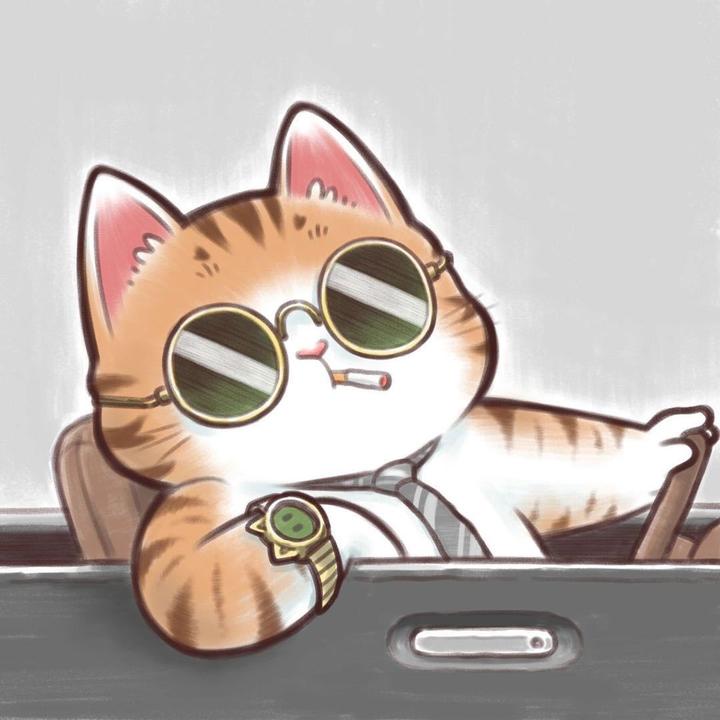

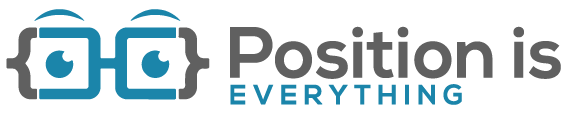







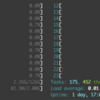
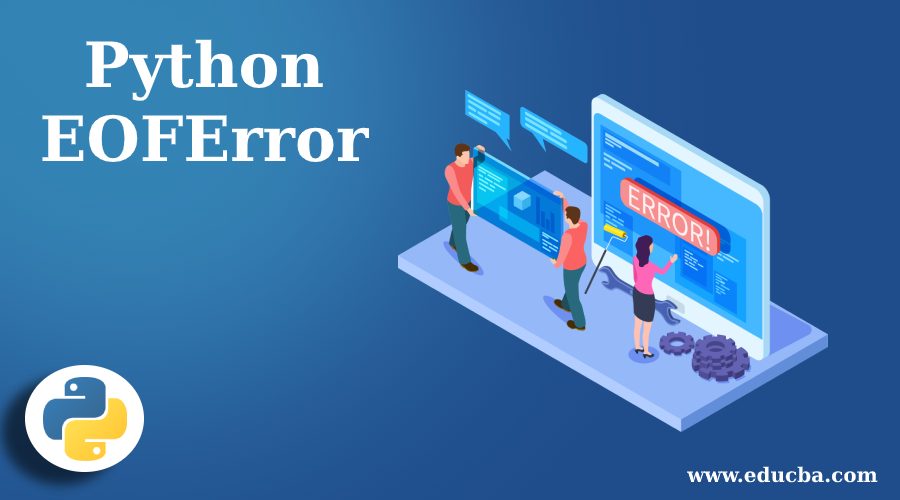

Article link: eoferror ran out of input.
Learn more about the topic eoferror ran out of input.
- Why do I get “Pickle – EOFError: Ran out of input” reading an …
- Ran Out of Input: A Guide To Understand and Solve the Error
- pickle.load(f) eoferror: ran out of input – AI Search Based Chat
- EOFError: Ran out of input · Issue #93 – GitHub
- Pickle EOFError: Ran out of input in Python [Solved] | bobbyhadz
- PyTorch Windows Eoferror: “ran out of input when …
- EOFError: Ran out of input – Lightrun
- Ran out of input when num_workers>0 – #22 by chaslie
- E0FError: Ran out of input, error training object detection
See more: nhanvietluanvan.com/luat-hoc