This Constructor Was Not Compatible With Dependency Injection.
Introduction
Dependency Injection (DI) is an essential concept in Angular development that promotes loose coupling, reusable code, and testability. However, there are instances where a constructor is not compatible with dependency injection. This article will discuss the reasons behind this incompatibility and provide solutions to overcome these challenges. We will also explore various Angular concepts such as `routertestingmodule`, `angular unit test service with dependencies`, `angular dependency injection with parameters`, and `angular dynamic component inject service`.
Reasons for Incompatibility with Dependency Injection
1. Lack of Interface Implementation
The first reason for a constructor’s incompatibility with dependency injection is the lack of interface implementation. Interfaces specify a contract for classes, allowing them to be interchangeable with other implementations. Without interface implementation, DI cannot determine which class should be injected, leading to compatibility issues.
2. Violation of Single Responsibility Principle
When a constructor violates the Single Responsibility Principle, it becomes challenging to inject dependencies efficiently. If a class has multiple responsibilities, it becomes tightly coupled with various dependencies, making it challenging to manage and maintain.
3. Presence of Non-Injectable Dependencies
Certain dependencies, such as third-party libraries or external services, may not support dependency injection. If a constructor relies on such non-injectable dependencies, it becomes incompatible with DI.
4. Tightly Coupled Dependencies
One of the primary goals of DI is to achieve loose coupling between classes. However, if a constructor has tightly coupled dependencies, it becomes difficult to swap or mock these dependencies during testing or future enhancements. This tight coupling results in the constructor’s incompatibility with DI.
5. Inability to Support Mocking and Testing
DI plays a crucial role in facilitating the mocking and testing of dependencies. A constructor that is not designed with DI in mind may hinder the ability to write effective unit tests or perform mocking. This incompatibility impacts the overall stability and maintainability of the application.
6. Difficulty in Managing and Configuring Dependencies
Lastly, a constructor not compatible with DI can lead to difficulties in managing and configuring dependencies. With DI, dependencies can be easily managed, instantiated, and configured through the framework. Without DI support, developers need to manually handle these dependencies, resulting in more complex and error-prone code.
Solutions and Angular Concepts
To address the incompatibility with dependency injection, the following solutions can be implemented:
1. Implement Interfaces: By introducing interfaces, the constructor can specify which implementation should be injected. This allows DI to resolve the dependencies correctly.
2. Refactor for Single Responsibility: Analyze the constructor and refactor it to adhere to the Single Responsibility Principle. Splitting the class into smaller, focused modules will improve compatibility with DI.
3. Wrapping Non-Injectable Dependencies: If non-injectable dependencies are necessary, create wrapper classes around them. These wrapper classes can then be properly injected into the constructor, enabling compatibility with DI.
4. Introduce Dependency Injection Containers: Consider using dependency injection containers like InversifyJS or Awilix. These containers can handle the complexities of managing dependencies, including support for non-injectable dependencies, and enable more seamless integration with DI.
Frequently Asked Questions (FAQs)
Q1: What is `routertestingmodule` in Angular?
`routertestingmodule` is a module provided by Angular’s testing framework to facilitate unit testing of routing-related functionality. It allows developers to simulate routing scenarios and test the behavior of components, guards, resolvers, and other routing-related features.
Q2: Can we write Angular unit tests for a service with dependencies?
Yes, Angular facilitates the testing of services with dependencies. By using test doubles (mocks or stubs) for the dependencies, services can be isolated and tested independently. `routertestingmodule` can also be used to create a TestBed environment for service testing.
Q3: How can we inject parameters in Angular dependency injection?
In Angular, dependencies are typically injected through the constructor using decorators like `@Inject`, `@Injectable`, or directly specifying the dependency in the constructor parameter. By declaring the dependencies in the constructor signature, Angular’s DI system automatically resolves and injects the dependencies.
Q4: How to perform unit testing for a service constructor in Angular?
To test a service constructor in Angular, create an instance of the service using `TestBed`. Then, assert the expectations by checking the state or behavior of that service instance.
Q5: How to inject a service in a dynamic component in Angular?
To inject a service in a dynamic component, use the `Injector` provided by Angular’s DI system. Pass the `Injector` instance to the dynamic component’s factory function, which injects the required service into the component.
Q6: Can we extend a class with dependency injection in Angular?
Yes, Angular supports extending classes with dependency injection. The extended class can utilize the parent’s dependencies or introduce its own. The DI system handles the injection process based on the class hierarchy.
Conclusion
Dependency Injection is a powerful concept that promotes modular, maintainable, and testable code. However, constructors that are not compatible with DI can hinder these benefits. By understanding the reasons for incompatibility and implementing the suggested solutions, developers can overcome these challenges and fully leverage the advantages of DI in Angular. Remember to utilize Angular concepts like `routertestingmodule`, create unit tests for services with dependencies, and configure DI efficiently to ensure optimal development practices and code quality.
Spring Core: Why Constructor Based Dependency Injection Is Recommended?
Is Constructor Is Not Compatible With Angular Dependency Injection Because Its Dependency At Index 0 Of The Parameter List Is Invalid?
Angular Dependency Injection Overview:
Angular is a popular framework for building web applications. One of its key features is dependency injection, which allows components to request dependencies from an injector. The injector then provides these dependencies to the component when it is being created. This helps manage a component’s dependencies and promotes loose coupling between different parts of an application.
When a component is created, Angular looks for its dependencies in a predefined hierarchy of injectors. Each injector provides a certain set of dependencies, and if a component’s dependency is not found in the current injector, Angular continues to look for it in the parent injector until it reaches the root injector. If the dependency is still not found, Angular throws an error indicating that the dependency is not available for injection.
Constructor Compatibility with Dependency Injection:
In Angular, a component’s dependencies are declared as parameters in its constructor. When the component is created, Angular uses reflection to inspect the constructor’s parameters and determine the dependencies it needs to provide.
To be compatible with Angular’s dependency injection system, a constructor needs to meet certain criteria. Firstly, the constructor must be a valid TypeScript constructor, meaning it should have the same number and order of parameters as its type definition. This ensures that the injector can provide the correct dependencies to the component.
Additionally, if a constructor has a dependency at index 0 that is invalid, it can cause compatibility issues with Angular’s dependency injection. By invalid, we mean a dependency that is not defined or cannot be resolved by the injector. This could happen if the required dependency is not declared in the module’s providers array or if there is a typo or mistake in the dependency’s declaration.
For example, let’s say we have a component called “UserService” that requires an instance of the “HttpClient” service to make HTTP requests. The constructor of the “UserService” would look like this:
constructor(private httpClient: HttpClient) { }
In this case, if the “HttpClient” service is not provided in the module’s providers array, Angular will throw an error stating that the dependency at index 0 (i.e., the “HttpClient” parameter) of the “UserService” constructor is invalid.
FAQs:
Q: What are some common reasons for an invalid dependency at index 0?
A: Some common reasons include forgetting to import the required module or service, misspelling the dependency’s name in the module’s providers array, or failing to declare the dependency altogether. Another possible reason could be that the required module or service is not properly imported into the current module.
Q: How can I fix the issue of an invalid dependency at index 0?
A: To fix the issue, you need to make sure the required dependency is properly declared and imported. Double-check the spelling and ensure that the module or service is added to the providers array in the component’s module.
Q: Can I have multiple dependencies in a constructor?
A: Yes, you can have multiple dependencies in a constructor. Angular will attempt to resolve all dependencies based on their index in the parameter list. However, if any dependency is invalid or cannot be resolved, it will cause compatibility issues.
Q: How can I avoid compatibility issues with constructor dependencies?
A: To avoid compatibility issues, always make sure that your constructor’s parameters match the expected dependencies and that those dependencies are correctly declared and imported. Follow good coding practices and regularly check for any errors or mistyped declarations.
Q: Are there any tools available to help identify constructor dependency issues?
A: Yes, Angular provides tools like static analyzers and linters, such as TSLint, that can help identify potential issues with constructor dependencies. These tools can catch common mistakes like misspelled dependencies or missing imports before you run your application.
In conclusion, constructor compatibility with Angular dependency injection is crucial for a smooth functioning of an application. An invalid dependency at index 0 in the parameter list can lead to compatibility issues and cause Angular to throw errors. It is important to ensure that the required dependencies are properly declared, imported, and added to the module’s providers array to avoid such issues. Regular code review and the use of tools like linters can greatly help in identifying and fixing these dependency-related problems.
What Is Constructor Dependency Injection C#?
Constructor Dependency Injection, also abbreviated as CDI, is a design pattern commonly used in C# programming to achieve loose coupling between classes. It is a form of dependency injection where the dependencies of a class are provided through its constructor. This approach is based on the principle of inversion of control, allowing dependencies to be resolved and injected by an external entity rather than the class itself.
In C#, a constructor is a special method that is called when an object of a class is created. It is responsible for initializing the object’s state and providing it with any required dependencies. By utilizing constructor dependency injection, classes become more maintainable, testable, and flexible.
How does Constructor Dependency Injection work?
Constructor Dependency Injection entails passing the required dependencies as parameters to a class constructor. When an instance of the class is created, the dependencies are automatically resolved and provided by an external component, usually a dependency injection container.
Let’s consider an example to understand this concept better. Assume we have two classes, `Car` and `Engine`, where the `Car` class requires an `Engine` object to function properly. Instead of creating an `Engine` object within the `Car` class, we can inject it through the constructor. Here’s how it would look in code:
“`csharp
public class Car
{
private readonly Engine _engine;
public Car(Engine engine)
{
_engine = engine;
}
// Car methods and properties…
}
public class Engine
{
// Engine methods and properties…
}
“`
In the above example, the `Car` class has a constructor that takes an `Engine` instance as a parameter. The constructor assigns the provided `Engine` object to the `_engine` field, making it available for use within the class. By using this approach, the `Car` class is no longer responsible for instantiating its own dependencies, making it easier to replace or mock the `Engine` object during testing or runtime.
Benefits of Constructor Dependency Injection:
1. Loose Coupling: CDI promotes loose coupling by separating the creation of dependencies from their usage within a class. This enables classes to be more independent and easier to maintain.
2. Testability: Constructor dependency injection facilitates unit testing, as dependencies can be easily replaced with mock objects during testing. This allows for isolated testing of individual components without the need for complex setup.
3. Flexibility and Extensibility: CDI makes it easier to extend or modify the behavior of a class by injecting different implementations of dependencies. This promotes code reuse and allows for seamless integration of new components.
FAQs:
Q1. Does constructor dependency injection require a dependency injection container?
A1. No, it is not mandatory to use a dependency injection container. While a container provides automated resolution of dependencies, you can manually instantiate and inject dependencies without a container.
Q2. Can a class have multiple constructors for dependency injection?
A2. Yes, a class with multiple constructors can support dependency injection. Having multiple constructors allows for flexibility in creating instances with different sets of dependencies.
Q3. What is the difference between constructor injection and property injection?
A3. Constructor injection supplies dependencies through a class constructor, while property injection sets dependencies through public properties. Property injection is considered less desirable as it allows for optional dependencies and may lead to a lack of clarity regarding required dependencies.
Q4. Can constructor dependency injection be used in legacy code?
A4. Yes, constructor dependency injection can be applied to existing codebases, including legacy code. It enables gradual refactoring and improves the maintainability and testability of legacy systems.
Q5. Are there any disadvantages to constructor dependency injection?
A5. Constructor dependency injection may introduce additional complexity, especially when dealing with classes that have a large number of dependencies. However, this can be mitigated by using a dependency injection container or employing other techniques like the facade or factory patterns.
In conclusion, constructor dependency injection is a powerful technique in C# programming that promotes loose coupling and enhances the testability, maintainability, and flexibility of classes. By relying on external components to resolve and inject dependencies, classes become decoupled, allowing for easier modifications, testing, and code reuse.
Keywords searched by users: this constructor was not compatible with dependency injection. angular dependency injection, routertestingmodule, angular unit test service with dependencies, angular dependency injection with parameters, angular unit test service constructor, This constructor was not compatible with Dependency Injection, angular dynamic component inject service, angular extend class with dependency injection
Categories: Top 15 This Constructor Was Not Compatible With Dependency Injection.
See more here: nhanvietluanvan.com
Angular Dependency Injection
Introduction
Angular is one of the most popular and widely used JavaScript frameworks for building web applications. One of the key features that make Angular highly extensible and flexible is its dependency injection (DI) system. In this article, we will dive deep into the concept of Angular dependency injection, understand its importance, and explore how it works within the framework. So, let’s get started!
Understanding Dependency Injection
Dependency injection is a design pattern used to develop loosely coupled software components, where dependencies between these components are not directly instantiated or managed by the component itself. Instead, the necessary dependencies are provided externally by an inversion of control container. Angular’s DI system simplifies this process by automatically resolving and injecting dependencies when required.
Why use Dependency Injection in Angular?
Using dependency injection in Angular offers numerous advantages. Firstly, it promotes loose coupling between components, making the codebase more modular and maintainable. It also enhances code reusability, as components can be easily substituted or extended with different implementations without affecting the overall structure of the application.
Additionally, DI facilitates unit testing. By injecting mocks or stubs of dependencies, developers can isolate and test individual components independently. It also makes the code more readable and easier to understand, as dependencies are explicitly defined in a single location.
How does Dependency Injection work in Angular?
Angular’s DI system functions based on three core concepts: providers, injectors, and tokens.
1. Providers: Providers define how instances of dependencies are created and configured. They can be defined at the component, module, or application level. Providers are typically accompanied by a token that uniquely identifies the dependency.
2. Injectors: Injectors are responsible for resolving dependencies and injecting them into the requesting components. Angular’s hierarchical injector tree ensures that dependencies are resolved from the nearest provider up to the root injector. This tree structure enables scoping and managing dependencies at different levels of the application.
3. Tokens: Tokens are used to identify and request dependencies. They can be either a class or an opaque value (such as a string or a symbol) created by the developer. Tokens are associated with providers, bridging the gap between dependencies and the requesting components.
Example of Dependency Injection in Angular
Let’s take a simple example to illustrate how dependency injection works in Angular.
Consider a hypothetical scenario where we have a component called “UserService” that requires an instance of a “HttpClient” to interact with a backend API. Here’s how you would define and use the dependency injection system to fulfill this requirement:
“`typescript
import { Component } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
@Component({
selector: ‘app-user’,
template: ‘
User Component
‘,
providers: [UserService]
})
export class UserService {
constructor(private http: HttpClient) {
// Use the httpClient instance to perform API requests
}
}
“`
In the example above, we declared the UserService as a provider in the component’s metadata, ensuring that the same instance is shared within the component and its descendants. The constructor of UserService expects an instance of HttpClient, which is automatically resolved and injected by Angular’s DI system.
FAQs (Frequently Asked Questions)
1. Is DI exclusive to Angular?
No, dependency injection is a general software development concept that can be
implemented in several programming languages and frameworks.
2. What are the alternatives to Angular’s DI system?
Some alternatives include manual dependency management, service locators, and
other DI frameworks like InversifyJS or NestJS.
3. Can I inject multiple instances of the same dependency?
Yes, Angular supports multiple instances of a dependency, known as multi-providers.
They are useful when you need to provide different implementations for the same token.
4. Can I inherit providers from parent components?
Yes, Angular’s DI system allows child components to access and use providers defined
in their parent components, propagating dependencies downwards.
Conclusion
Dependency injection is a powerful concept that brings flexibility, modularity, and testability to Angular applications. By relying on Angular’s DI system, developers can easily manage and resolve dependencies, resulting in more maintainable and scalable codebases. Understanding the three core concepts of providers, injectors, and tokens is crucial for leveraging the benefits of dependency injection in your Angular projects. So go ahead, embrace Angular’s dependency injection and level up your web development skills!
Routertestingmodule
Introduction
In the digital age, where the internet plays a vital role in our daily lives, having a high-performance router is crucial. However, it can be challenging to determine which router best suits your needs. Fortunately, router testing modules have emerged as a reliable solution by providing comprehensive evaluations of router performance. In this article, we will delve into the world of router testing modules, exploring their purpose, benefits, and the key factors to consider when choosing a module.
What is a Router Testing Module?
A router testing module is a piece of software or hardware that assists in evaluating the performance of a router. It utilizes various metrics and tests to assess the speed, reliability, and stability of a router’s functions. The module ensures that the router meets specific standards, user expectations, and can handle network traffic effectively.
Key Benefits of Using a Router Testing Module
1. Accurate Performance Evaluation: A router testing module utilizes multiple tests to assess the speed, latency, and throughput of the router accurately. This information helps users gauge if the router is capable of handling their network demands effectively.
2. Network Compatibility: With the vast array of routers available in the market, determining the compatibility of a router with a particular network can be challenging. A router testing module simplifies this process by testing network compatibility and ensuring seamless integration with existing systems.
3. Stability Analysis: Stability is a vital aspect of any router, particularly for those used in business settings. A router testing module identifies potential performance issues, such as dropped connections or intermittent signal fluctuations, which can disrupt productivity.
4. Security Assessment: With the increasing presence of cyber threats, it is crucial to ensure that a router has robust security features. A router testing module assesses the security protocols and encryption capabilities of the router, providing valuable insights to enhance network safety.
Key Factors to Consider When Choosing a Router Testing Module
1. Test Coverage: Evaluate the range of tests offered by the module. Ideally, it should include tests focusing on throughput, latency, data packet loss, and network stability. A wider range of tests ensures a more comprehensive evaluation of router performance.
2. Scalability: Consider the scalability of the router testing module to accommodate future growth. It should be capable of handling higher network volumes and have the ability to simulate real-world scenarios.
3. User-Friendly Interface: A router testing module should have a user-friendly interface that allows users, regardless of their technical expertise, to easily navigate through the testing procedures, interpret the results, and generate reports.
4. Compatibility: Ensure that the router testing module is compatible with a wide range of router models and firmware versions. This flexibility ensures the module’s long-term usability, even if you decide to switch routers in the future.
FAQs (Frequently Asked Questions)
Q1. Is a router testing module necessary for home users?
A1. While it is not essential for all home users, if you require a reliable and consistent internet connection, a router testing module can help you identify potential performance issues, improve Wi-Fi coverage, and enhance overall network stability.
Q2. How often should I test my router using the testing module?
A2. It is recommended to conduct regular tests every few months, especially if you notice any significant changes in your network performance or experience persistent connection issues.
Q3. Can a router testing module fix my router’s performance issues?
A3. No, a router testing module primarily serves to identify issues and provide insights into the router’s performance. It is not designed to fix the underlying problems with the router itself. However, armed with the module’s analysis, you can take appropriate measures to improve your router’s performance.
Q4. Are router testing modules compatible with all operating systems?
A4. Most router testing modules are compatible with major operating systems such as Windows, MacOS, and Linux. However, it is essential to check the module’s specifications to ensure compatibility with your specific operating system.
Q5. Can a router testing module improve my internet speed?
A5. A router testing module does not directly improve your internet speed. Its purpose is to assess the router’s performance and help you identify factors that may affect the speed. If the module highlights any issues, addressing them can potentially enhance your internet speed.
Conclusion
With the increasing importance of a robust and reliable internet connection, a router testing module has become an invaluable tool. It provides accurate evaluations of router performance, identifies potential issues, and enhances network stability. By considering the key factors mentioned above, you can choose a router testing module that best suits your specific needs, ensuring a seamless internet experience for personal or business use.
Images related to the topic this constructor was not compatible with dependency injection.
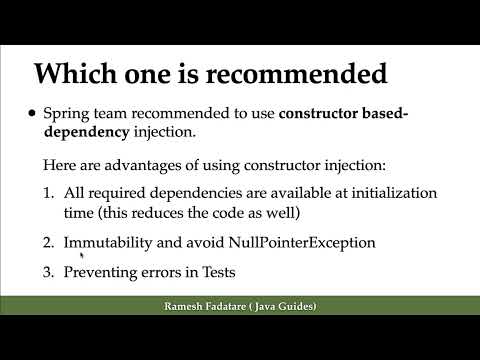
Found 13 images related to this constructor was not compatible with dependency injection. theme
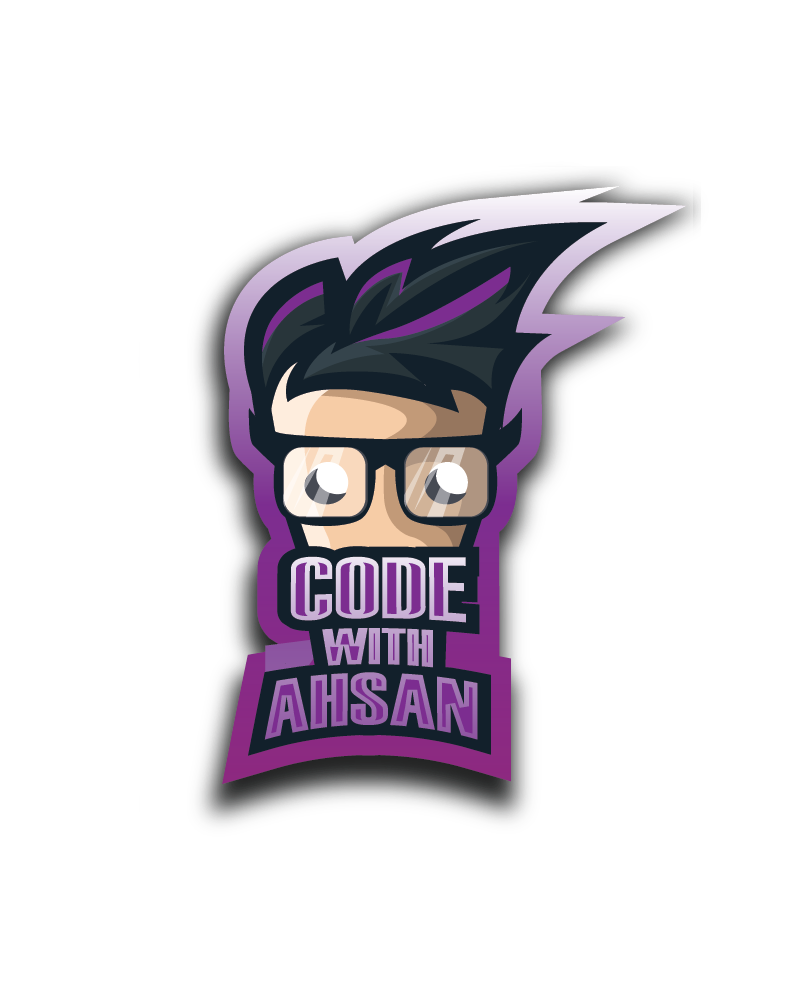

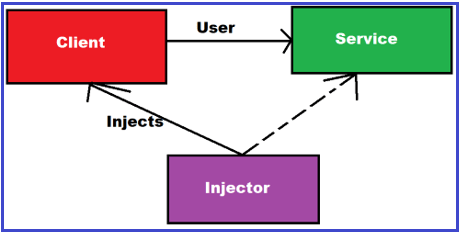
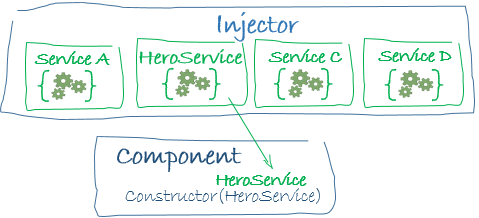
Article link: this constructor was not compatible with dependency injection..
Learn more about the topic this constructor was not compatible with dependency injection..
- Angular 9 “Error: This constructor was not compatible with …
- This constructor is not compatible with Angular Dependency …
- Error :This constructor is not compatible with Angular …
- Constructor parameter in service is not compatible … – Lightrun
- Angular Unit Tests with Jest – This constructor is not …
- This constructor is not compatible with Angular Dependency …
- Implementation of Dependency Injection Pattern in C# – DotNetTricks
- A Complete Guide To Angular Dependency Injection – Simplilearn
- Understanding dependency injection – Angular
- Understanding dependency injection – Angular
- This constructor was not compatible with Dependency Injection.
- Ivy compatibility examples – Angular Hispano
See more: nhanvietluanvan.com/luat-hoc