Executable_Path Has Been Deprecated Please Pass In A Service Object
Background on executable_path deprecation:
The use of executable_path in Selenium WebDriver has been a common way to specify the path of the browser driver executable. By providing the executable path, WebDriver is able to locate and launch the corresponding browser for testing purposes. However, due to various reasons, the executable_path feature has been deprecated and the use of service objects is now recommended.
What is executable_path in Selenium WebDriver?
Executable_path is a parameter that allows WebDriver to locate and execute the browser driver executable file. This executable file is responsible for establishing a connection between WebDriver and the chosen web browser for automation. By specifying the executable_path, WebDriver knows where to find the driver and bind it with the selected browser.
How was executable_path traditionally used?
Traditionally, executable_path was used by WebDriver users to set the path to the browser driver binary. For example, when using ChromeDriver, users would specify the path to the chromedriver executable file on their system using the executable_path parameter. This allowed WebDriver to find the appropriate driver and launch the Chrome browser for automated testing.
Why has executable_path been deprecated?
The deprecation of executable_path in Selenium WebDriver is attributed to several factors. One of the main reasons is the need for a more flexible and modular approach to managing WebDriver instances. The executable_path approach lacked the necessary abstraction and made it difficult to switch between different browsers and platforms without modifying the code.
Introduction of service objects in Selenium WebDriver:
To address the limitations of executable_path, Selenium WebDriver introduced the concept of service objects. These service objects provide a more robust and flexible way to manage WebDriver instances and their associated browser drivers. Instead of directly specifying the executable path, developers now pass in a service object that is responsible for launching and managing the WebDriver instance.
What are service objects?
Service objects in Selenium WebDriver act as intermediaries between the automation code and the browser drivers. They encapsulate the logic required to start, stop, and manage the WebDriver instances. Each browser driver (e.g., ChromeDriver, GeckoDriver, etc.) has its own corresponding service object that handles the specific operations related to that particular browser.
How do service objects differ from executable_path?
The major difference between service objects and executable_path lies in the level of abstraction and control they provide. While executable_path was a direct parameter to the WebDriver constructor, service objects allow for more fine-grained control over the WebDriver instances. They handle the lifecycle of the drivers, provide configuration options, and handle compatibility issues.
Benefits of using service objects over executable_path:
1. Improved Code Modularity and Reusability: Service objects promote better code organization by separating the concerns of WebDriver instantiation and browser driver management. This leads to more maintainable and reusable code.
2. Enhanced Flexibility and Control over WebDriver Instances: Service objects provide a higher level of control over the WebDriver instances, allowing developers to configure various options, such as specifying command-line arguments or setting browser preferences.
3. Simplified Management of WebDriver Services: Service objects simplify the management of WebDriver services by handling the startup and shutdown of browser drivers automatically. They ensure that the WebDriver instances are properly initialized and terminated, reducing the chances of resource leaks.
Migration process from executable_path to service objects:
To migrate from using executable_path to service objects, the following steps can be followed:
1. Replace the executable_path parameter with the appropriate service object for the desired browser (e.g., ChromeDriverService for Chrome).
2. Update the code to utilize methods provided by the service object, such as starting and stopping the service.
3. Modify any configuration options that were previously set using the executable_path.
4. Address any compatibility issues that may arise during the migration. For example, some command-line arguments or preferences might need to be set through the service object instead of directly through the executable_path.
Advantages of using service objects:
1. Improved code modularity and reusability: Service objects allow for cleaner and more modular code, making it easier to reuse and maintain.
2. Enhanced flexibility and control over WebDriver instances: Service objects provide more control over WebDriver instances, allowing for advanced configuration options and customizations.
3. Simplified management of WebDriver services: Service objects handle the lifecycle of WebDriver instances, ensuring proper startup and shutdown of browser drivers.
Common scenarios for using service objects:
1. Implementing different WebDriver service types: With service objects, it is easier to switch between different browser drivers by simply using the corresponding service object for the desired browser.
2. Running WebDriver on different platforms and browsers: Service objects provide a unified interface to interact with different browser drivers, making it easier to run tests on various platforms and browsers.
3. Managing multiple instances of WebDriver: Service objects allow for easy management and control of multiple WebDriver instances, enabling parallel test execution and distributed testing.
Best practices for utilizing service objects effectively:
1. Encapsulating service object initialization and configuration: It is recommended to encapsulate the initialization and configuration of service objects within a separate class or module to promote code reusability.
2. Proper error handling and cleanup of WebDriver services: Ensure that WebDriver services are properly stopped and cleaned up after test execution to prevent resource leaks and conflicts.
3. Extending service object functionality with custom logic: Service objects can be extended to include custom logic or additional functionality, such as custom browser options or handling specific scenarios during test execution.
Conclusion and considerations:
The deprecation of executable_path in Selenium WebDriver highlights the need for a more flexible and modular approach to managing WebDriver instances. By utilizing service objects, developers gain improved control, flexibility, and code maintainability. Migrating from executable_path to service objects may require some code changes and compatibility handling, but the benefits outweigh the effort. By embracing service objects, WebDriver users can ensure better test automation practices and adapt to future advancements in Selenium 4 and beyond.
Selenium: How To Resolve \”Deprecationwarning: Executable_Path Has Been Deprecated\” + زیرنویس فارسی
Keywords searched by users: executable_path has been deprecated please pass in a service object Fix DeprecationWarning executable_path has been deprecated, please pass in a Service object, deprecationwarning: use options instead of chrome_options, deprecationwarning: executable_path has been deprecated, please pass in a service object firefox, selenium.common.exceptions.webdriverexception: message: unknown error: cannot find chrome binary, Selenium 4, Selenium don t close browser, ChromeDriver, Selenium Python
Categories: Top 52 Executable_Path Has Been Deprecated Please Pass In A Service Object
See more here: nhanvietluanvan.com
Fix Deprecationwarning Executable_Path Has Been Deprecated, Please Pass In A Service Object
Introduction:
When writing automation scripts or web scrapers using Selenium, one common practice is to use the executable_path argument to specify the path to the browser’s executable file. However, starting from Selenium version 4.0.0, this approach has been deprecated, and developers are encouraged to pass in a Service object instead. In this article, we will take an in-depth look at this deprecation warning, understand why it was deprecated, and learn how to fix it in different programming languages.
Why was executable_path deprecated?
The deprecation of executable_path and the introduction of the Service object is aimed at improving the maintainability and extensibility of Selenium. By passing in a Service object instead of a raw executable file path, it allows for better customization and flexibility when dealing with various browser providers.
The executable_path approach tightly couples the application code with the specific browser’s executable path. This can be problematic when switching between different browsers or when browser updates require a change in the executable path. With the Service object, you can create a more abstract and portable solution to handle these scenarios.
How to fix it:
To fix the deprecated executable_path warning, you need to pass a Service object to the browser driver instance instead. The Service object is responsible for starting the browser’s service and is specific to each browser provider.
Below, we will demonstrate how to fix the warning in Python, Java, and JavaScript:
Python:
1. Import the necessary libraries:
“`python
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
“`
2. Replace the executable_path parameter with the service parameter:
“`python
service = ChromeService(executable_path=’/path/to/chromedriver’)
driver = webdriver.Chrome(service=service)
“`
Java:
1. Import the necessary libraries:
“`java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeDriverService;
“`
2. Replace the executable_path parameter with the service parameter:
“`java
System.setProperty(“webdriver.chrome.driver”, “/path/to/chromedriver”);
ChromeDriverService service = new ChromeDriverService.Builder().build();
WebDriver driver = new ChromeDriver(service);
“`
JavaScript (Node.js):
1. Install the necessary dependencies:
“`bash
npm install selenium-webdriver chromedriver
“`
2. Require the libraries and create a Service object:
“`javascript
const { Builder, Service } = require(“selenium-webdriver”);
const chrome = require(“chromedriver”);
const service = new Service.Builder()
.usingChromeDriverPath(chrome.path)
.build();
“`
3. Replace executable_path with the service parameter when creating the driver:
“`javascript
const driver = new Builder().forBrowser(“chrome”).setChromeService(service).build();
“`
FAQs:
Q1. What is the purpose of the Service object?
The Service object is responsible for starting and managing the browser’s service, such as starting the browser process and managing its lifecycle.
Q2. Can I still use the executable_path parameter?
While it is still possible to use the executable_path parameter in Selenium 4.0.0, it is deprecated, and its usage is discouraged. It is recommended to switch to the Service object approach for better maintainability and future compatibility.
Q3. Does this change affect all browsers?
Yes, the deprecation of executable_path and the introduction of the Service object apply to all supported browsers, including Chrome, Firefox, Safari, and Internet Explorer.
Q4. What if I don’t pass a Service object?
If you don’t pass a Service object, Selenium will use the default behavior, which may not provide the necessary flexibility and customization options. It is highly recommended to pass a Service object to ensure a consistent and future-proof solution.
Q5. How do I update my existing scripts to use the Service object?
To update your existing scripts, follow the language-specific examples provided earlier and replace the occurrences of executable_path with the appropriate Service object initialization and usage.
Q6. Can I still set additional options for the Service object?
Yes, the Service object provides methods to set additional options specific to each browser provider. Consult the documentation of the respective browser to learn more about the available options.
Conclusion:
The deprecation of executable_path and the introduction of the Service object in Selenium version 4.0.0 mark a significant change in how browser executables are handled. By embracing the Service object approach, developers can write more maintainable, flexible, and extensible automation scripts and web scrapers. Remember to update your existing code to remove the deprecated executable_path and replace it with the appropriate Service object initialization. With this change, you’ll be well-prepared for future browser updates and avoid the DeprecationWarning.
Deprecationwarning: Use Options Instead Of Chrome_Options
The development landscape is constantly evolving, and as technologies advance, certain methods and practices become outdated. One such instance is the deprecation of chrome_options in the popular web automation library, Selenium. In this article, we will explore what this deprecation means, why it is happening, and how it affects developers who utilize Selenium for automated browser testing.
What is Deprecation?
Deprecation is a software development term used to indicate that a particular feature, method, or practice will be removed or replaced in future versions of a framework or library. This is usually done to improve code quality, enhance security, or promote better practices. Deprecation serves as a warning to developers that they should transition away from the deprecated feature, as its continued usage may result in issues or even break their code in the future.
DeprecationWarning in Selenium
One of the most significant deprecations in Selenium is the warning to start using options instead of chrome_options. ChromeOptions is a class in Selenium’s WebDriver API that allows developers to specify various settings and preferences when launching the Chrome browser through Selenium. However, as the Selenium project has evolved, the developers have decided to move away from the term chrome_options and rebrand it as options to provide greater flexibility and uniformity across different browser drivers.
Why Use options Instead of chrome_options?
There are a few compelling reasons why developers should start using options instead of chrome_options when working with Selenium.
1. Improved Flexibility: By using options, developers can ensure their code remains compatible across multiple browser drivers, rather than being limited to Chrome only. This improves code reusability and allows for easier switching between different browsers during the development and testing phases.
2. Code Readability: The term options is more intuitive and self-explanatory than chrome_options. It makes the code more readable and understandable for both new and experienced developers who work with Selenium.
3. Better Future Support: As developers transition to using options, they align themselves with the ongoing improvements and developments in Selenium. Opting for the newer and recommended approach ensures compatibility and support with future versions of Selenium and its associated browser drivers.
FAQs
Q: Will my existing code using chrome_options break?
A: In most cases, existing code utilizing chrome_options should continue to work without any issues. The deprecation warning is primarily to encourage developers to adopt the newer and recommended practice of using options instead.
Q: How do I transition my code from chrome_options to options?
A: The transition process is straightforward. Replace all occurrences of chrome_options with options in your codebase. Additionally, make sure to update any relevant import statements to reflect the change in class name.
Q: Are there any differences in functionality between chrome_options and options?
A: No, both chrome_options and options serve the same purpose of providing settings and preferences for launching the Chrome browser through Selenium. The deprecation is aimed at ensuring uniformity and compatibility across different browser drivers.
Q: Can I continue using chrome_options in older versions of Selenium?
A: Yes, chrome_options will still work in older versions of Selenium. However, it is highly recommended to update your code to use options for better support and compatibility with the latest versions.
Q: Are there any alternatives to options for launching browsers in Selenium?
A: Yes, options is not limited to Chrome only. For other browsers, such as Firefox or Safari, different options classes or profiles can be utilized. These classes provide similar functionalities in specifying browser preferences, just like options does for Chrome.
In conclusion, the deprecation of chrome_options in Selenium brings a more unified and flexible approach to launching browsers using Selenium’s WebDriver API. By transitioning to options, developers can ensure better compatibility, code readability, and future support. While existing code using chrome_options will continue to function, it is strongly recommended to update your codebase to leverage the advancements and improvements in Selenium.
Deprecationwarning: Executable_Path Has Been Deprecated, Please Pass In A Service Object Firefox
If you have experience with web scraping or automated testing using Selenium and Python, you may have come across the DeprecationWarning message stating, “executable_path has been deprecated, please pass in a service object Firefox.” This warning has caused confusion among developers and leaves many wondering how to properly handle it. In this article, we’ll delve into the topic, explaining what this deprecation warning means, why it was made deprecated, and how to address it with the newest implementation.
What is the executable_path in Selenium?
In the context of Selenium, the executable_path is the path to the WebDriver executable file, which is used by Selenium to interface with different browsers. It is a crucial part of Selenium setup as it helps locate and execute the browser driver for automated browser control.
The WebDriver executable file is specific to each browser, such as ChromeDriver for Google Chrome, GeckoDriver for Mozilla Firefox, or EdgeDriver for Microsoft Edge. By providing the correct executable_path, Selenium knows where to find the necessary driver executable.
Why was executable_path deprecated?
The deprecation of executable_path is a result of changes in the underlying GeckoDriver implementation for Firefox. Up until now, developers had to provide the path to the GeckoDriver executable manually. However, with the newer versions of Selenium, it is recommended to pass in a service object instead.
The service object encapsulates the configuration and behavior of the WebDriver service, making it more manageable and abstracted. By introducing this change, Selenium aims to provide a more consistent API across different browser drivers and simplify the setup process.
How to address the DeprecationWarning?
To get rid of the DeprecationWarning, you need to pass in a service object for Firefox instead of the executable_path.
Here’s how you can modify your code:
“`python
from selenium import webdriver
from selenium.webdriver.firefox.service import Service
# Specify the path to the GeckoDriver executable (old approach)
# driver = webdriver.Firefox(executable_path=’/path/to/geckodriver’)
# Create a service object for Firefox
service = Service(‘/path/to/geckodriver’)
# Pass in the service object
driver = webdriver.Firefox(service=service)
“`
In the provided code snippet, we import the necessary modules, including the Service class from selenium.webdriver.firefox.service. Then, we create a Service object by specifying the path to the GeckoDriver executable.
Finally, when creating the Firefox WebDriver instance, we pass in the service object using the `service` parameter. This allows Selenium to handle the browser and driver setup accordingly.
FAQs:
Q1: Is it mandatory to switch from executable_path to the service object for Firefox?
A1: While it is not strictly mandatory, it is highly recommended to switch to the service object to ensure compatibility and future-proof your code. The deprecation warning serves as a reminder to update your implementation accordingly.
Q2: Does this change affect only Firefox or other browsers as well?
A2: As of now, this change is specific to Firefox. Other browsers, such as Chrome or Edge, still require the usual executable_path approach. However, Selenium may introduce similar changes in the future for other browser drivers.
Q3: How can I ensure my code is compatible with various versions of Selenium?
A3: To ensure compatibility, it is essential to regularly update your Selenium package. Check the official Selenium documentation and release notes for any breaking changes. Additionally, running your code against various Selenium versions in a test environment can help catch any potential compatibility issues.
Q4: Can I pass additional arguments or options to the service object for Firefox?
A4: Yes, you can pass additional arguments or options when creating the service object. For example, specifying the log_path parameter allows you to define a log file for capturing browser and driver logs.
In conclusion, the deprecation of executable_path in Selenium for Firefox indicates a shift towards using a service object for better abstraction and consistency. By updating your code and passing in a service object, you can stay updated with the latest Selenium implementation and avoid the DeprecationWarning. Remember to keep an eye on the official Selenium documentation and regularly update your packages to ensure compatibility with future versions of Selenium.
Images related to the topic executable_path has been deprecated please pass in a service object
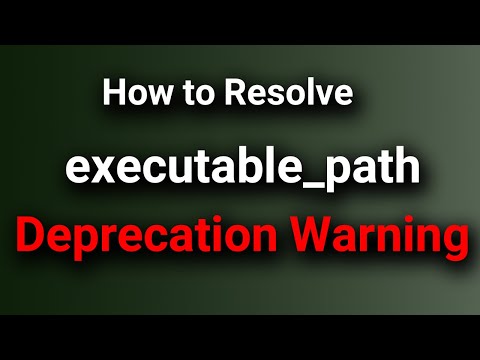
Found 48 images related to executable_path has been deprecated please pass in a service object theme

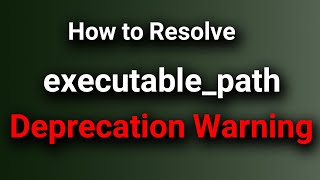
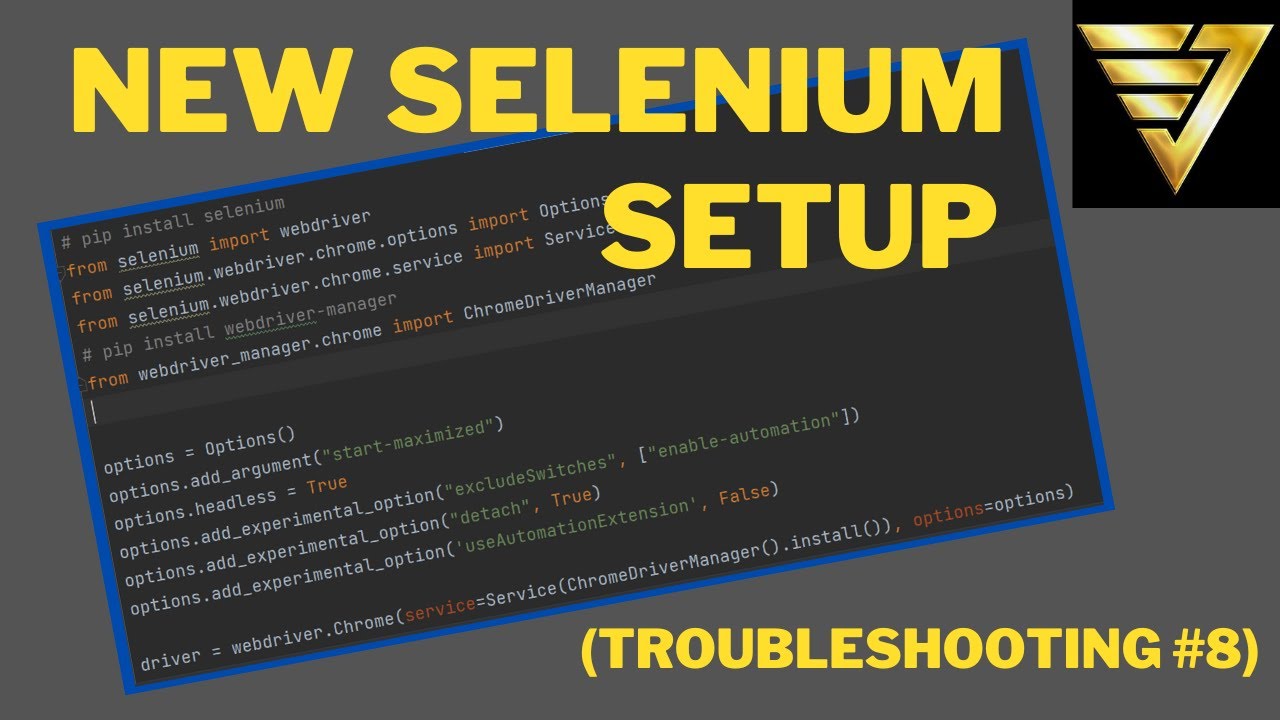
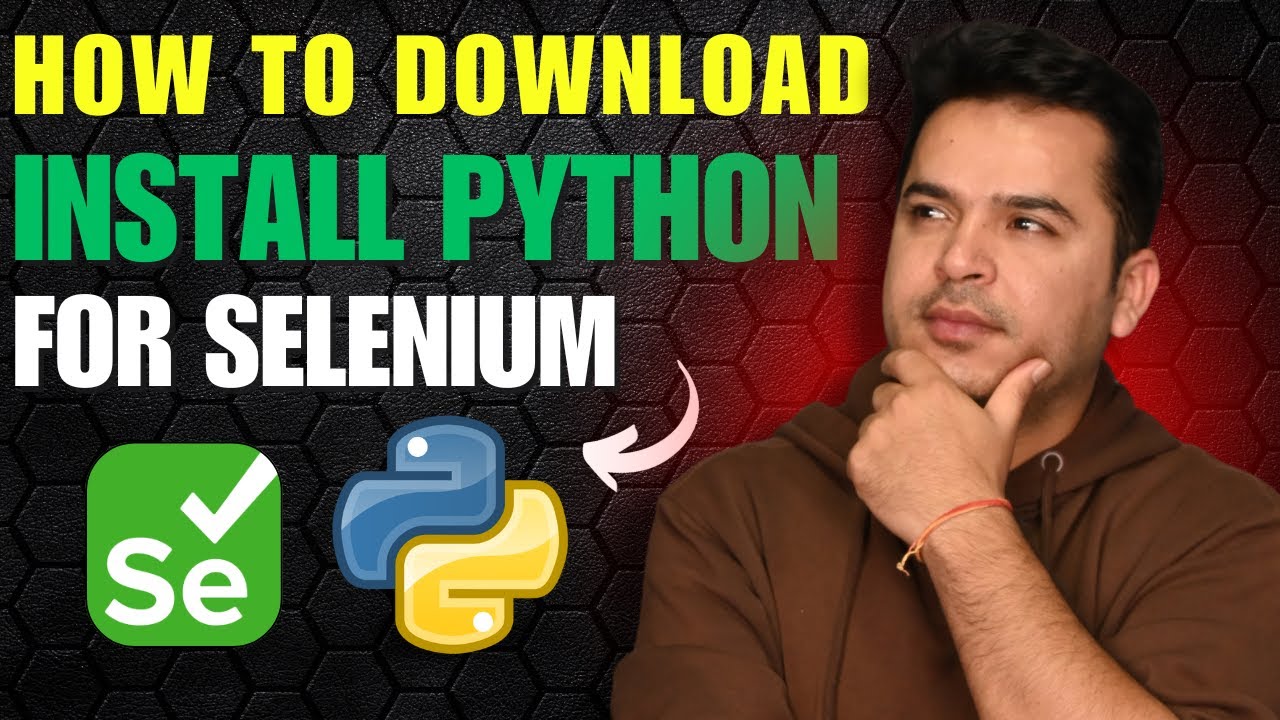
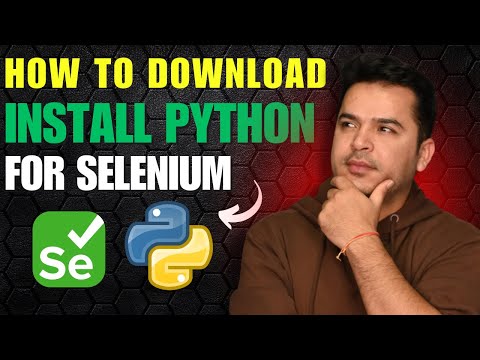
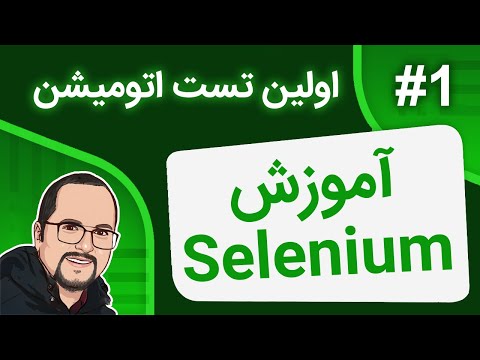
![Selenium] AttributeError: 'WebDriver' object has no attribute 'find_element_by_css_selector' Selenium] Attributeerror: 'Webdriver' Object Has No Attribute 'Find_Element_By_Css_Selector'](https://img1.daumcdn.net/thumb/C176x176/?fname=https://blog.kakaocdn.net/dn/ryNbt/btsglQ298sL/o7t1DkCauDaxRoGhaZuCi1/img.png)
![Python 셀레니움] executable_path has been deprecated, please pass in a Service object 에러 해결 방법 Python 셀레니움] Executable_Path Has Been Deprecated, Please Pass In A Service Object 에러 해결 방법](https://blog.kakaocdn.net/dn/Aavei/btrv4zqmKB6/deLyKm36NhVqff7ZjjIRBK/img.png)


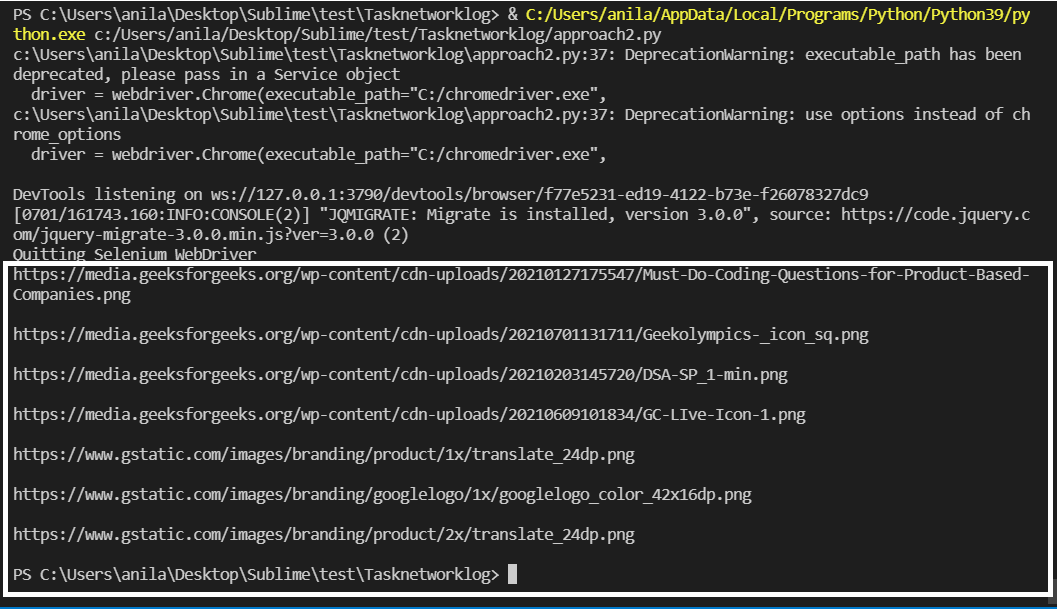
![220118 [코딩공부] DeprecationWarning: executable_path has been deprecated, please pass in a Service object 오류 해결 공부 220118 [코딩공부] Deprecationwarning: Executable_Path Has Been Deprecated, Please Pass In A Service Object 오류 해결 공부](https://blog.kakaocdn.net/dn/bduVpP/btrqX0OAjHS/IHviB6OjvDjTjyzkjUV6HK/img.png)
![Python 셀레니움] executable_path has been deprecated, please pass in a Service object 에러 해결 방법 Python 셀레니움] Executable_Path Has Been Deprecated, Please Pass In A Service Object 에러 해결 방법](https://img1.daumcdn.net/thumb/R800x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2Fneunp%2Fbtrv3f0c2oR%2FzfeqJ2AtX3wpwI4FBhI8Ok%2Fimg.png)


![220118 [코딩공부] DeprecationWarning: executable_path has been deprecated, please pass in a Service object 오류 해결 공부 220118 [코딩공부] Deprecationwarning: Executable_Path Has Been Deprecated, Please Pass In A Service Object 오류 해결 공부](https://blog.kakaocdn.net/dn/2TwKS/btrqRZbDRvB/3Lu7JUHgGDB9JQcIfj6zoK/img.png)
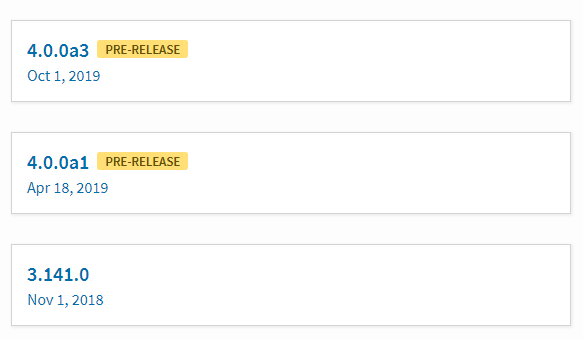







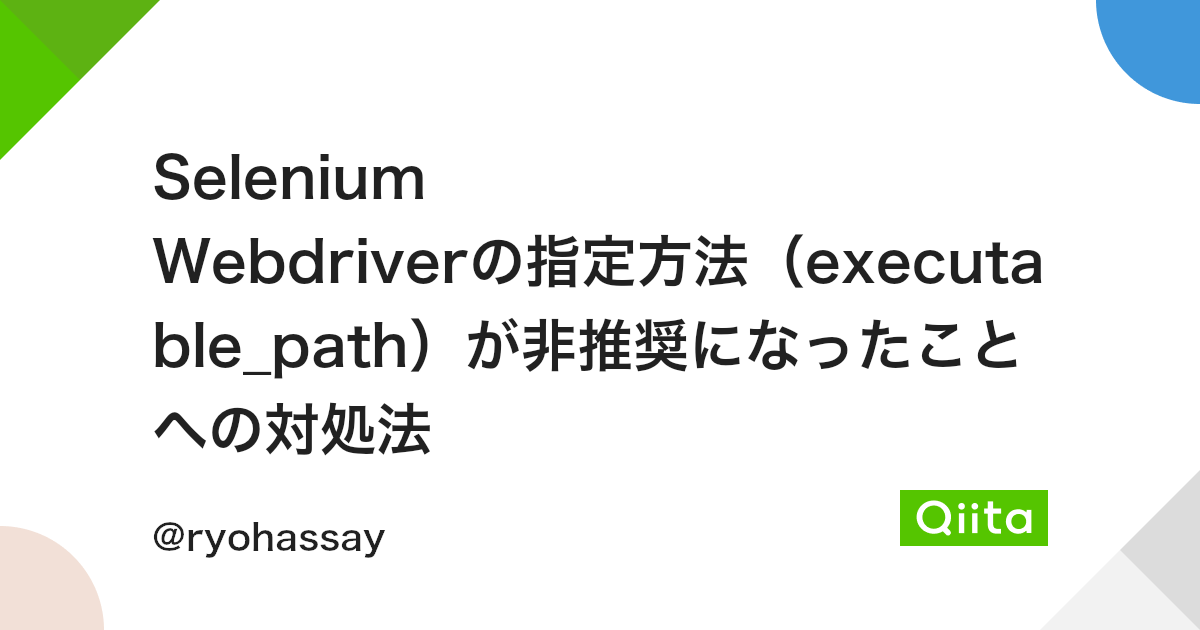


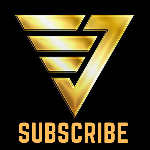
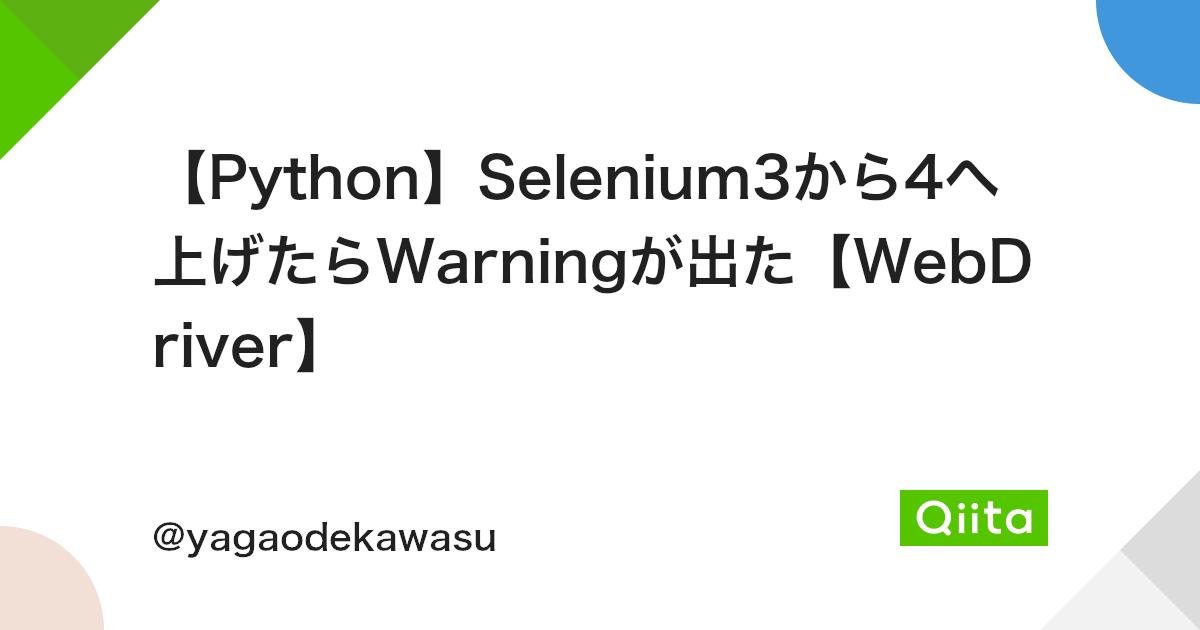
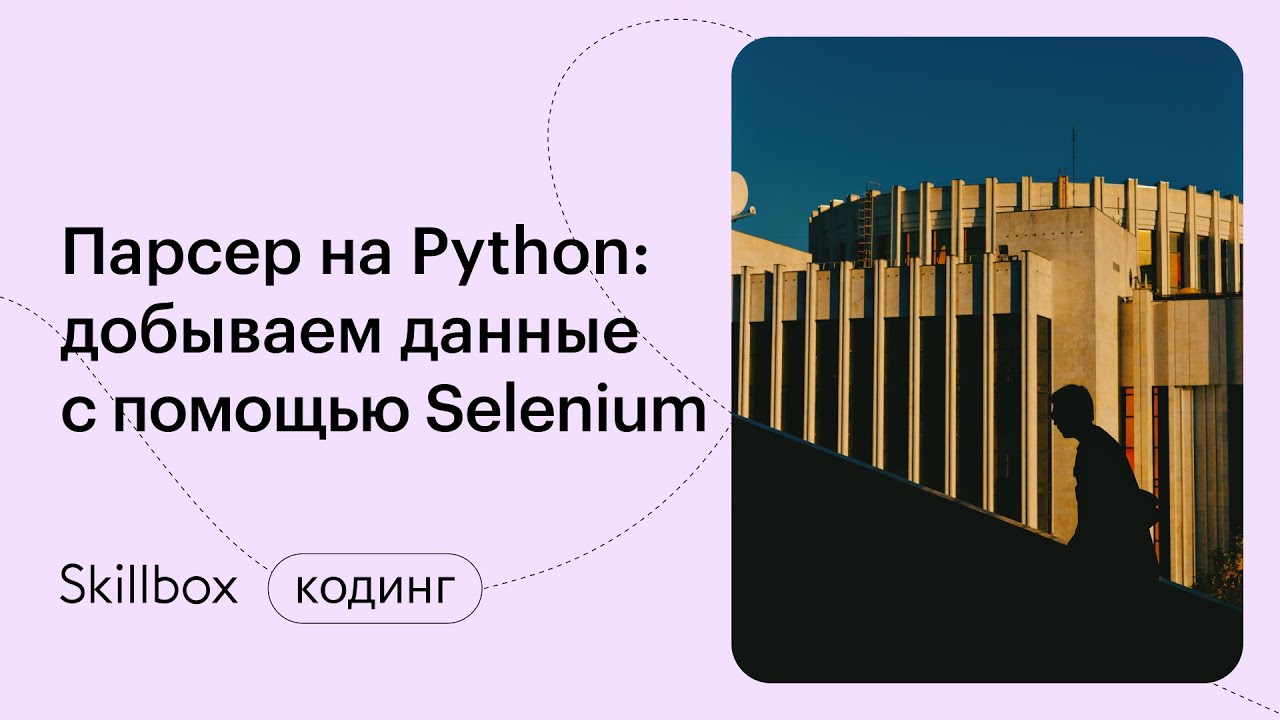

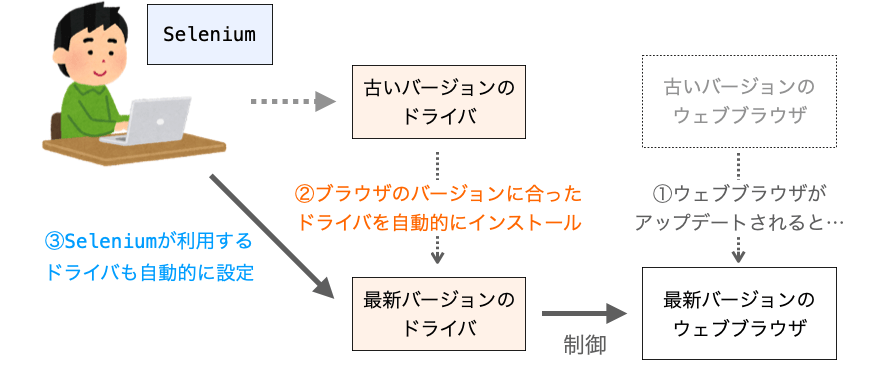
Article link: executable_path has been deprecated please pass in a service object.
Learn more about the topic executable_path has been deprecated please pass in a service object.
- executable_path has been deprecated selenium python
- DeprecationWarning: executable_path has been deprecated
- executable_path has been deprecated, please pass in a …
- executable_path has been deprecated selenium python
See more: nhanvietluanvan.com/luat-hoc