Python List Index -1
In Python, lists are an important data structure that allows you to store and manipulate collections of items. One useful feature of lists is the ability to access individual elements using indexing. Indexing allows you to retrieve specific elements from a list based on their position.
Positive Indexing:
In Python, lists are zero-indexed, meaning that the first element of a list has an index of 0, the second element has an index of 1, and so on. Positive indexing refers to accessing elements from the start of a list using positive integers as indices.
For example, consider the following list:
numbers = [10, 20, 30, 40, 50]
To access the element at index 2 (which is the third element in the list), you can use positive indexing like this:
second_element = numbers[2]
In this case, second_element will be assigned the value 30, as it corresponds to the element at index 2 in the list.
Negative Indexing:
In addition to positive indexing, Python also supports negative indexing. Negative indexing allows you to access elements from the end of a list using negative integers as indices. This can be particularly useful when working with lists whose length is not known in advance.
Using negative indexing, the last element in a list has an index of -1, the second-to-last element has an index of -2, and so on.
For example, let’s consider the same list as before:
numbers = [10, 20, 30, 40, 50]
To access the last element in the list, you can use negative indexing like this:
last_element = numbers[-1]
In this case, last_element will be assigned the value 50, as it corresponds to the last element in the list.
Using -1 to Access the Last Element of a List:
One of the most common use cases for negative indexing is to access the last element of a list. By using -1 as the index, you can easily retrieve the last element regardless of the list’s length.
For example:
numbers = [10, 20, 30, 40, 50]
last_element = numbers[-1]
In this case, last_element will be assigned the value 50, as it corresponds to the last element in the list.
Applying -1 with Slicing:
In addition to accessing individual elements, Python also allows you to use negative indexing in conjunction with slicing to extract sublists from a list.
Slicing allows you to retrieve a portion of a list by specifying a range of indices. By combining negative indexing with slicing, you can easily extract elements from the end of a list.
For example, consider the following list:
numbers = [10, 20, 30, 40, 50]
To extract the last three elements of the list, you can use the following syntax:
last_three_elements = numbers[-3:]
In this case, last_three_elements will be assigned a new list containing the elements [30, 40, 50].
Common Mistakes with -1 Indexing:
While -1 indexing can be a powerful tool in Python, it can also lead to some common mistakes if not used correctly. Here are a few issues to watch out for:
1. Off-by-one errors: Since Python lists are zero-indexed, it’s important to remember that using -1 as an index will always refer to the last element, not the second-to-last. Be cautious when performing calculations or iterations with negative indices.
2. Out of range errors: Using negative indices that are too large or negative in value will result in an “index out of range” error. Remember that the valid range of negative indices for a list of length n is from -n to -1.
3. Confusion with positive indexing: Mixing positive and negative indexing can lead to confusion and bugs in your code. Always be consistent with your indexing approach and use comments or clear variable names to indicate the rationale behind your choices.
4. Misunderstanding the concept: Some beginners may struggle to grasp the concept of negative indexing at first. Practice using negative indices with simple examples to build a solid understanding of their behavior.
Python developers commonly utilize positive indexing with lists; however, negative indexing offers a convenient way to access elements from the end of the list without knowing its length in advance.
FAQs:
Q: Can I use negative indexing with other Python data structures?
A: Negative indexing is supported in strings and tuples as well, allowing you to access elements from the end in a similar fashion.
Q: Are negative indices the only way to access the last element of a list?
A: No, another way to access the last element of a list is by using positive indexing with the index -1. However, negative indexing is generally more intuitive in this scenario.
Q: Can I use negative indices with a step value in slicing?
A: Yes, negative indices can be combined with a step value in slicing to extract sublists in reverse order.
Q: Can I use negative indices in a for loop to iterate over a list?
A: Yes, negative indices can be used in a for loop to access elements in reverse order. However, keep in mind that the loop variable will store the values, not the indices themselves.
Q: How do I access the second-to-last element of a list using negative indexing?
A: You can use the index -2 to access the second-to-last element of a list.
In conclusion, Python’s list indexing feature allows for easy access to individual elements within a list. Negative indexing is particularly useful when working with lists of unknown length or when accessing elements from the end. By understanding the correct usage of -1 indexing and avoiding common mistakes, you can effectively manipulate lists in Python.
The First Lists Index Is 0
What Is List [- 1 In Python?
Python is a versatile programming language that offers a wide range of features and functionalities. Python lists are one of the fundamental data structures provided by the language, allowing users to store and manipulate collections of items. In Python, it is possible to access and retrieve items from a list using various indexing techniques. One such indexing technique is the use of a negative index, which allows us to access elements from the end of a list. The syntax to access the last element of a list using a negative index is list[-1].
In Python, the indexing of lists starts from 0, with the first element being accessed using list[0], the second using list[1], and so on. Conversely, when using negative indexes, the last element of the list can be accessed using list[-1], the second-to-last using list[-2], and so forth. So, by using negative indexing, we can easily retrieve elements from the end of a list without knowing its length or using list length functions.
Let’s consider an example to better illustrate the concept:
“`
my_list = [1, 2, 3, 4, 5]
last_element = my_list[-1]
print(last_element)
“`
The output of this code will be:
“`
5
“`
In the above example, we have a list called `my_list` containing the integers 1 to 5. By using `my_list[-1]`, we are able to retrieve the last element of the list, which is 5. This showcases the utility of negative indexing in Python when working with lists.
FAQs:
Q: Can we use any negative integer as an index?
A: While it is possible to use any negative integer as an index, it is important to keep in mind that it should not exceed the length of the list. For example, using list[-6] when the list only has 5 elements will result in an `IndexError` since there is no element at the specified index.
Q: Are there any performance implications when using negative indexes?
A: When using negative indexes, Python internally converts them to their corresponding positive indexes. Therefore, there should not be any noticeable performance difference between using positive or negative indexes to access list elements.
Q: Can we modify list elements using negative indexes?
A: Yes, list elements can be modified using negative indexes just like positive indexes. For instance, `my_list[-1] = 10` will update the last element of the list to have a value of 10.
Q: Can we use negative indexes with other Python data structures?
A: Negative indexing is specific to lists and does not work with other data structures such as tuples or strings. Only lists support negative indexing out of the box in Python.
Q: How can we access multiple elements from the end of a list using negative indexes?
A: To access multiple elements from the end of a list, you can use a range of negative indexes. For example, `my_list[-3:]` will retrieve the last three elements of the list. Similarly, `my_list[:-2]` will retrieve all elements except the last two.
In conclusion, list[-1] in Python allows us to easily access the last element of a list using negative indexing. This feature is particularly useful when the length of the list is unknown or when performing operations relative to the end of the list. By understanding and utilizing negative indexing, Python programmers can efficiently retrieve and manipulate elements from the end of a list without explicitly knowing its length.
Is Python List Index 0 Or 1?
Python is a widely used and versatile programming language that is known for its simplicity and readability. When it comes to indexing elements in a list, Python follows a convention where the index starts at 0. This means that the first element in a list is accessed using the index 0, not 1.
Understanding how Python lists are indexed can be crucial for writing correct and efficient code. Let’s delve deeper into this topic and explore why the indexing starts at 0 in Python lists.
In programming, an index is used to uniquely identify elements within a data structure such as an array, list, or string. The concept of zero-based indexing, which Python adopts, has its roots in mathematics and computer science. Early programming languages like C and Fortran also followed this convention to make memory management and array operations more efficient.
One of the main reasons behind zero-based indexing in Python lists is the simplicity it offers. When starting the index at 0, it aligns the indices directly with the memory addresses of the elements. This allows for more predictable and intuitive code, as it closely resembles the way data is stored in the computer’s memory.
Another advantage of zero-based indexing is that it simplifies the calculation of indices across programming languages. Since many programming languages follow the zero-based convention, it becomes easier for developers to switch between them without needing to adjust their mindset regarding indexing.
To demonstrate this, let’s consider a simple Python list. Suppose we have a list of fruits:
fruits = [‘apple’, ‘banana’, ‘mango’, ‘orange’]
To access the first element of the list, we would use the index 0:
first_fruit = fruits[0] # ‘apple’
Similarly, to access the second element, we use the index 1:
second_fruit = fruits[1] # ‘banana’
This consistent indexing pattern goes on for all elements in the list. By starting at 0, we can easily access any element precisely by specifying its index.
Frequently Asked Questions:
Q: Why does Python use zero-based indexing?
Python uses zero-based indexing to align closely with how data is stored in the computer’s memory. It also simplifies index calculations across multiple programming languages that follow the same convention.
Q: How do I access the last element of a list in Python?
To access the last element of a list, you can use the -1 index. In Python, -1 represents the last element, -2 represents the second last, and so on. For example:
last_fruit = fruits[-1] # ‘orange’
Q: Is it possible to change the index of a list in Python?
No, the index of a list in Python is fixed and cannot be changed. It always starts with 0 and increments by 1 for each subsequent element.
Q: Are other data structures in Python also zero-based?
In addition to lists, other data structures in Python such as strings and tuples also follow the zero-based indexing convention.
Q: How can I find the index of a specific element in a list?
In Python, you can use the index() method to find the index of a specific element in a list. The index() method returns the first occurrence of the element’s index. For example:
fruit_index = fruits.index(‘mango’) # 2
In conclusion, Python lists use zero-based indexing, meaning the first element in a list is accessed using the index 0. This indexing convention not only simplifies code and memory operations but also allows for easier transition between programming languages. Understanding this fundamental aspect of Python lists will help you write more efficient and accurate code.
Keywords searched by users: python list index -1 Python list 1, Index 1 Python
Categories: Top 18 Python List Index -1
See more here: nhanvietluanvan.com
Python List 1
Python, a highly versatile and popular programming language, offers a wide range of data structures, each designed to serve specific purposes. One of the most commonly used data structures in Python is a list. In this article, we will dive deep into Python List 1, exploring its various features, applications, and providing a comprehensive usage guide.
What is Python List 1?
A list, in Python, is an ordered collection of items, where each item is assigned an index value that represents its position within the list. Python List 1 is the initial version of a list, available since the first release of Python. It provides essential functionalities and serves as a foundation for more advanced list implementations in subsequent Python versions.
Creating a Python List 1
To create a list in Python, the items are enclosed within square brackets ([]), and each item is separated by a comma. Let’s look at a simple example of creating a Python List 1:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’, ‘date’]
“`
In this example, we have created a list called “my_list” containing four items. The items are strings representing different fruits.
Accessing and Modifying List Elements
Python List 1 allows easy access to individual elements by their index values. Indexing starts from 0, where the first element has an index of 0, the second has an index of 1, and so on. To access an element, we use square brackets and specify the index position. For example:
“`python
print(my_list[0]) # Output: ‘apple’
“`
Here, we access the first element of “my_list” using its index value of 0.
Lists in Python are mutable, which means their elements can be modified after creation. Let’s modify an element in our list:
“`python
my_list[2] = ‘grape’ # ‘cherry’ has been replaced with ‘grape’
“`
Now, our list will contain ‘apple’, ‘banana’, ‘grape’, and ‘date’.
Basic List Operations
Python List 1 supports several fundamental operations that make it versatile and convenient to work with. Some of these operations include:
1. Concatenation: We can combine two or more lists into a single list using the concatenation operator (‘+’).
“`python
combined_list = my_list + [‘fig’, ‘guava’]
print(combined_list) # Output: [‘apple’, ‘banana’, ‘grape’, ‘date’, ‘fig’, ‘guava’]
“`
2. Repetition: We can create a new list by repeating an existing list multiple times, using the repetition operator (‘*’).
“`python
repeated_list = my_list * 3
print(repeated_list) # Output: [‘apple’, ‘banana’, ‘grape’, ‘date’, ‘apple’, ‘banana’, ‘grape’, ‘date’, ‘apple’, ‘banana’, ‘grape’, ‘date’]
“`
3. Slicing: We can extract a portion of a list using slicing, which allows us to specify a range of indices.
“`python
sliced_list = my_list[1:3]
print(sliced_list) # Output: [‘banana’, ‘grape’]
“`
These are just a few of the many operations and functionalities that Python List 1 offers.
Frequently Asked Questions (FAQs)
Q1. Can we mix different data types in a Python List 1?
Yes, Python lists can contain elements of different data types. For example, a list can contain a mix of strings, numbers, and even other lists.
Q2. How can we check if an item exists in a Python List 1?
We can use the ‘in’ operator to check if an item is present in a list. It returns a boolean value of True if the item is found, and False if it is not.
Q3. Is it possible to sort a Python List 1?
Yes, Python provides the ‘sort’ method to sort a list in ascending order. By default, it sorts in ascending order, but we can reverse the order using the ‘reverse’ parameter.
Q4. Can we merge two lists without using the ‘+’ operator?
Yes, Python provides the ‘extend’ method that allows us to append elements from another list to the end of the current list, without using the ‘+’ operator.
Q5. Are the operations on Python List 1 time-efficient?
Python List 1 operations such as accessing elements by index, modifying elements, and appending elements are very efficient and have constant time complexity, which makes them highly efficient.
In conclusion, Python List 1 is a fundamental and versatile data structure that serves as the backbone of list implementations in Python. It provides various operations and functionalities that facilitate efficient handling of data. By understanding the basics and exploring its applications, you can leverage the power of Python lists to enhance your programming capabilities.
Index 1 Python
Python, one of the most popular programming languages today, offers a wide range of powerful features that make it a favorite among developers. One of these features is indexing, which allows users to access specific elements within data structures like lists, strings, and tuples.
Indexing in Python starts at 0, meaning the first element of a collection is accessed using index 0. However, an interesting capability of Python indexing is the concept of Index 1. In this article, we will explore the power of Index 1, how to use it effectively, and its applications in real-world scenarios.
Understanding Index 1 in Python:
By default, Python uses Index 0 to access the first element of a collection. However, Index 1 allows a more intuitive way of accessing elements, where the index directly corresponds to the position of the element in the collection. This feature provides convenience and simplicity, especially for beginners who are more accustomed to counting from 1 rather than 0.
To utilize Index 1 in Python, you need to employ the slice notation. This is done by adding 1 to the desired index value. Let’s see an example to understand it better:
“`
my_list = [‘apple’, ‘banana’, ‘orange’, ‘watermelon’]
print(my_list[1]) # Output: ‘banana’
“`
In the above code snippet, we are using Index 1 to access the second item in the list, which is ‘banana’. This is accomplished by adding 1 to the desired index value.
Applications of Index 1:
Index 1 can be useful in various scenarios, such as when dealing with human-readable data or when working with APIs that use 1-based indexing. Let’s explore a few use cases where Index 1 can be particularly handy.
1. Working with CSV or spreadsheet data:
CSV (comma-separated values) files are commonly used to store tabular data. When reading a CSV file, the first row often contains the column headers. With Index 1, developers can access the data more naturally, since columns are usually labeled starting from 1.
2. Dealing with natural language processing:
In some natural language processing tasks, such as sentiment analysis or named entity recognition, we need to identify specific words or phrases in a sentence. Using Index 1 allows us to refer to words based on their position as they naturally occur in the text, rather than using the somewhat counterintuitive Index 0.
Frequently Asked Questions about Index 1:
Q1. What is the advantage of using Index 1 in Python?
Using Index 1 can make code more intuitive, especially for beginners. It aligns with how we count items in everyday life, where the first item is considered to be at position 1 rather than 0.
Q2. Can I use Index 1 with any data structure in Python?
Index 1 can be used with any data structure that supports indexing, such as lists, strings, and tuples. However, it’s important to note that it is not the default behavior in Python, so you need to explicitly use the slice notation to access elements using Index 1.
Q3. Are there any performance implications when using Index 1?
No, there are no significant performance differences between using Index 0 or Index 1 in Python. The choice between the two is purely based on personal preference and the requirements of the particular use case.
Q4. Can I mix Index 0 and Index 1 in the same code?
Yes, Python allows mixing both indexing methods in the same code. However, it is generally considered good practice to stick to a consistent indexing approach throughout your codebase for clarity and maintainability.
Q5. How can I convert from Index 1 to Index 0 and vice versa?
To convert from Index 1 to Index 0, simply subtract 1 from the desired index value. Likewise, to convert from Index 0 to Index 1, add 1 to the desired index value. These operations can be easily performed in Python.
In conclusion, Index 1 in Python provides a simplified and intuitive way of accessing elements within data structures. While Python’s default Index 0 is widely used, Index 1 can be particularly useful when working with human-readable data or APIs that leverage 1-based indexing. It offers a more natural coding experience, making it easier for beginners to understand and work with. Although Index 0 remains the standard in Python, Index 1 adds versatility and convenience to the language, enriching the programming experience for developers of all skill levels.
Images related to the topic python list index -1
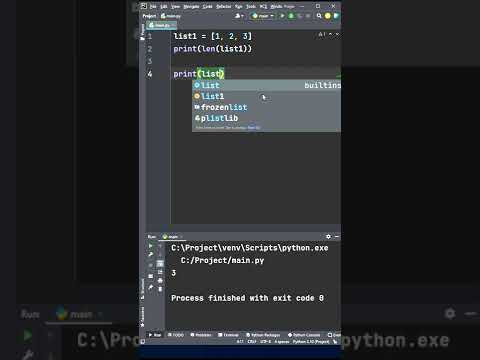
Found 12 images related to python list index -1 theme
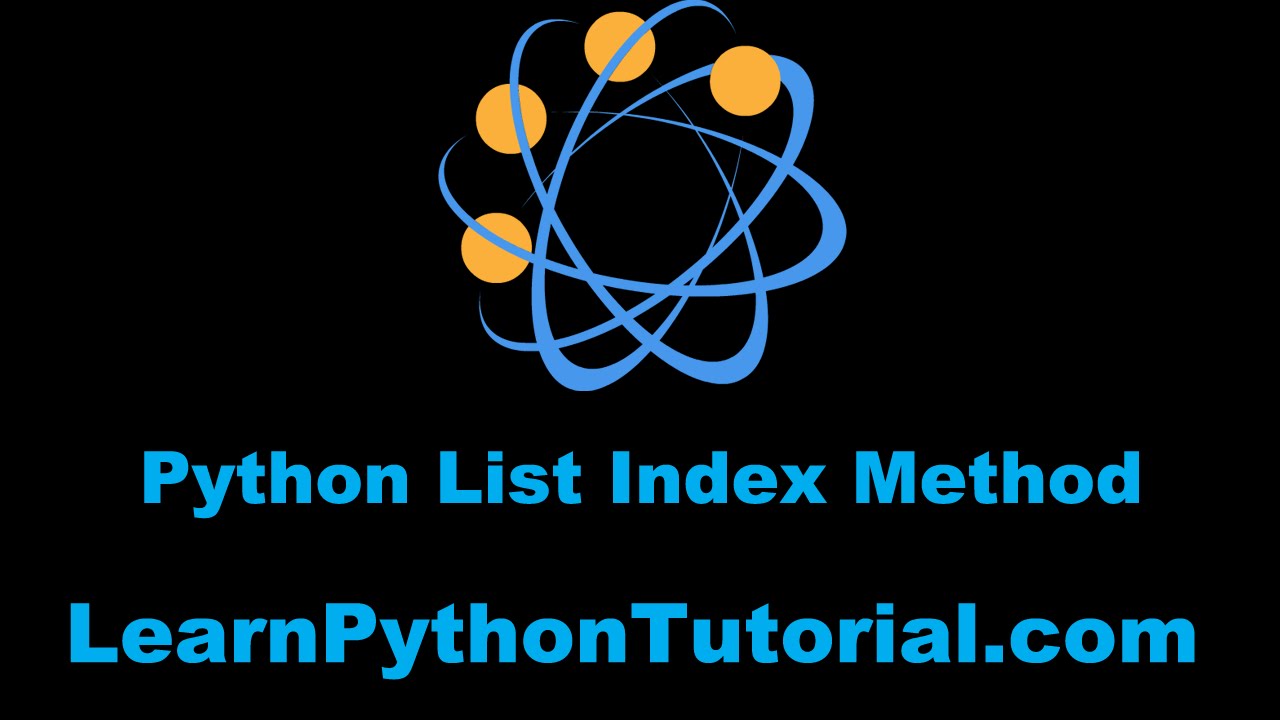



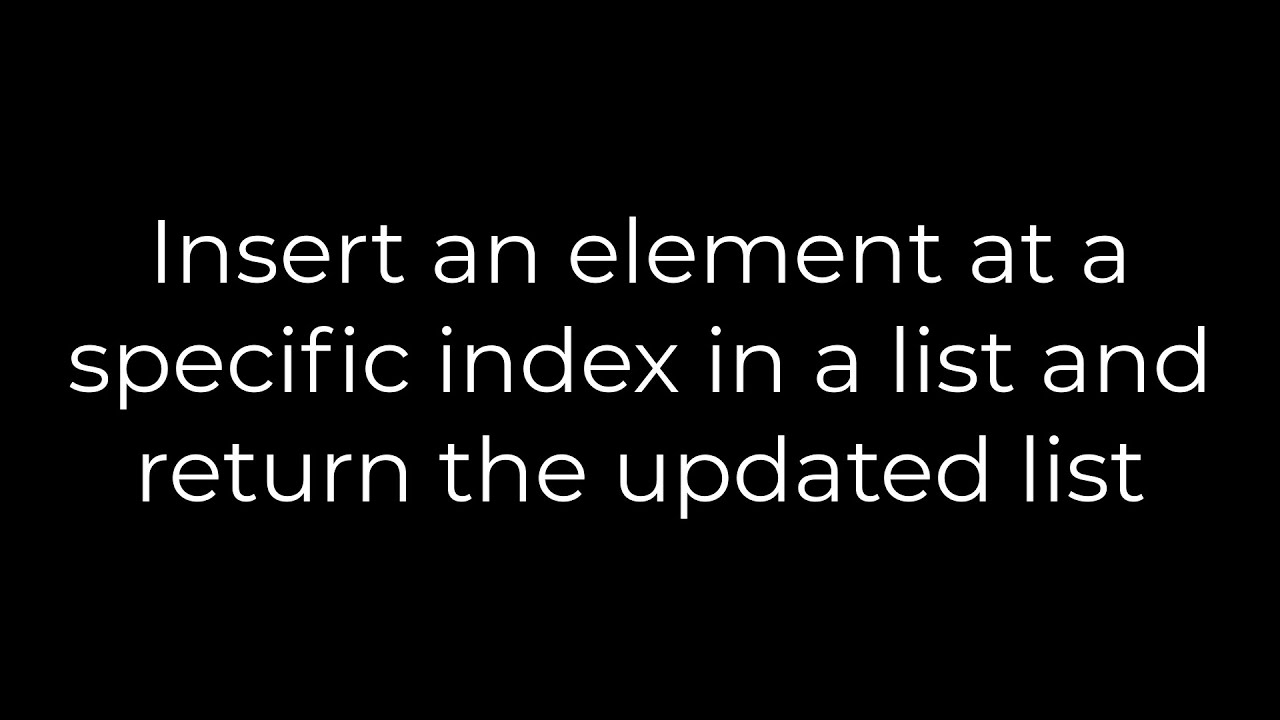




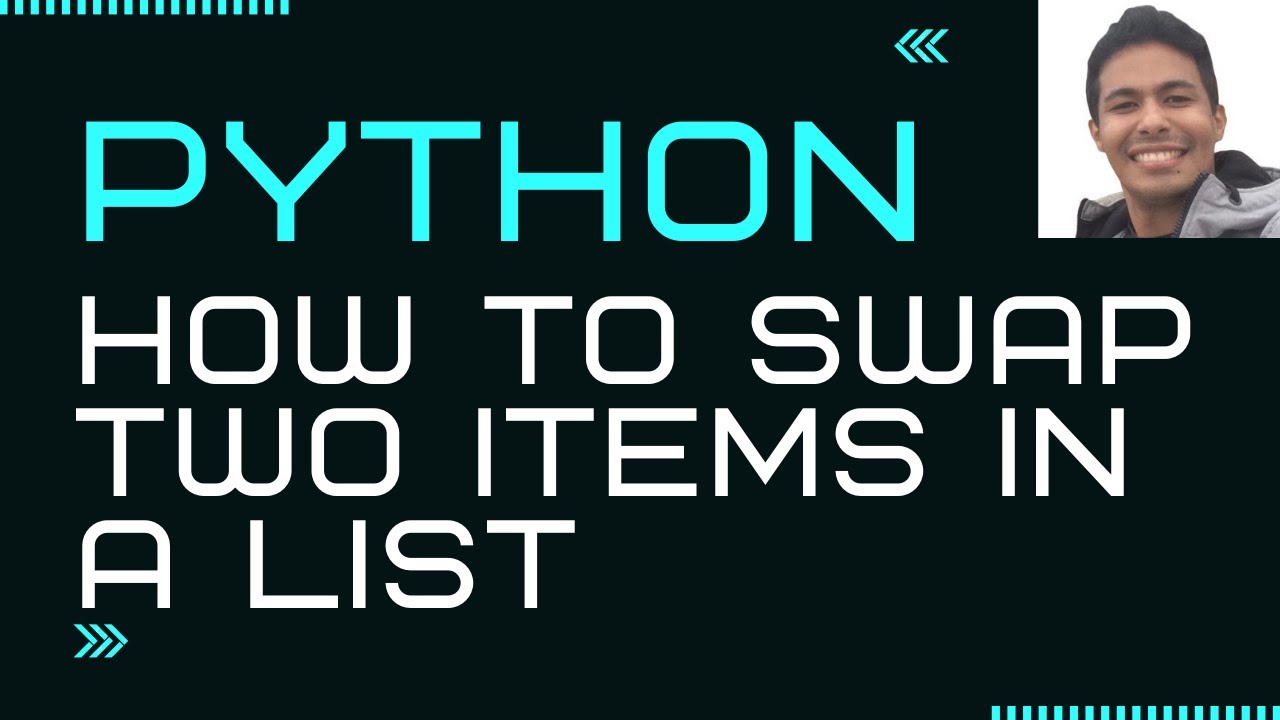
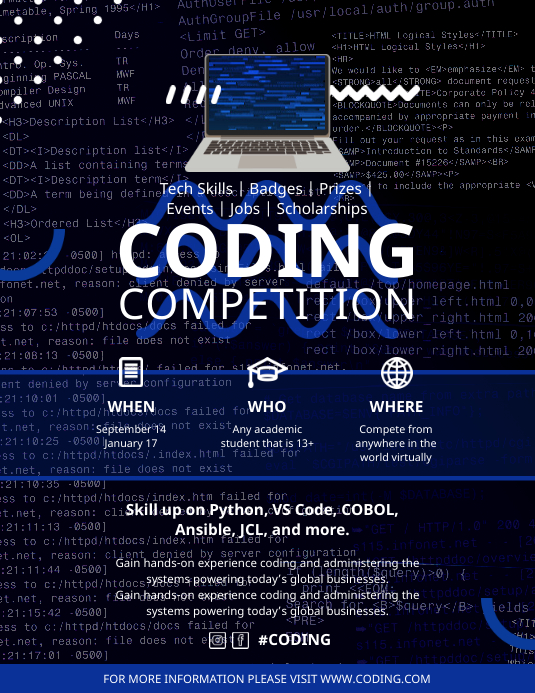
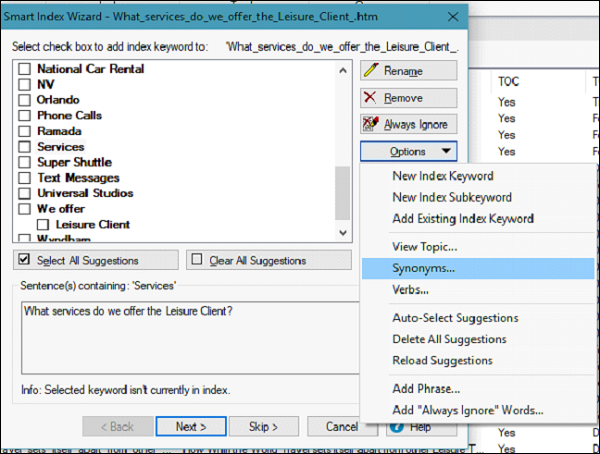

Article link: python list index -1.
Learn more about the topic python list index -1.
- How do I find the index of an element in a list in Python?
- Meaning of list[-1] in Python – Stack Overflow
- A Quick intro to Indexing in Python – HackerEarth
- Negative list index? [duplicate] – python – Stack Overflow
- Python Find in List – How to Find the Index of an Item or Element in a List
- How do I find the index of an item in a list in Python?
- In Python, how to get list element by index from the end?
- How to find the indices of all `True` values in a list of booleans …
- L Slicing Values – Pandas for Everyone: Python Data … – O’Reilly
- Python list index – AiHints
- Codeitmi – Python list index() is an inbuilt function that…
- Python Program that print elements common at specified …
- last item – PythonHow
- List Remove by Index in Python | Delft Stack
See more: nhanvietluanvan.com/luat-hoc