Retrieve Multiple Records From Crm Javascript
Querying the CRM Data
Before diving into the different retrieval methods, let’s first understand how to query the CRM data using JavaScript. JavaScript provides various query options for CRM, allowing you to filter, sort, and retrieve specific records based on your requirements. These query options include FetchXML, OData, and the Web API.
FetchXML is a powerful XML-based query language for CRM, which offers flexibility and the ability to retrieve complex data. By constructing a FetchXML query in JavaScript, you can specify conditions, select attributes, and even perform aggregates and joins as needed.
OData is a standardized protocol that allows you to access data from different sources, including CRM. It provides simple and consistent syntax for querying and manipulating data. With OData, you can filter, sort, and retrieve records based on specific criteria, making it a useful option for retrieving multiple records from CRM using JavaScript.
The Web API, also known as the Dynamics 365 Web API, is a feature-rich API provided by Microsoft for working with CRM data. It offers a wide range of functionalities for record retrieval, including support for querying, filtering, sorting, and paging through large result sets.
Using FetchXML to Retrieve Records
Now let’s dive into using FetchXML to retrieve records from CRM using JavaScript. FetchXML provides a structured and versatile way to query CRM data, making it an excellent choice for retrieving multiple records. Here are the steps to construct a FetchXML query in JavaScript:
1. Define the entity name and attributes you want to retrieve.
2. Specify any conditions or filters using the FetchXML syntax.
3. Optionally, include sorting instructions if needed.
4. Use the SDK.REST.js library, a popular JavaScript library for CRM development, to execute the FetchXML query and retrieve the records.
The SDK.REST.js library provides a set of functions and utilities for interacting with CRM using JavaScript. It simplifies the process of making HTTP requests and handling responses, making it easier to work with the CRM Web API.
Leveraging OData to Retrieve Records
Another method for retrieving multiple records from CRM using JavaScript is by leveraging OData. OData provides a standardized way to query and manipulate data, making it a popular choice for CRM integrations. Here’s how you can implement and execute OData queries in JavaScript:
1. Construct the OData query URL, specifying the entity set, select attributes, filter conditions, and any other options required.
2. Use JavaScript’s XMLHttpRequest or a library like axios to make an HTTP GET request to the OData endpoint of your CRM system.
3. Parse the response data and retrieve the desired records.
OData offers a wide range of query options, including filtering, sorting, paging, and expanding related records. This flexibility makes it a powerful tool for retrieving multiple records from CRM using JavaScript.
Working with Web API to Retrieve Records
The Web API is a comprehensive API provided by Microsoft for working with CRM data. It offers a rich set of functionalities specifically designed for record retrieval. Here are some examples of using the Web API to retrieve records in JavaScript:
1. Use the `RetrieveRecord` function to retrieve a single record based on its unique identifier.
2. Use the `RetrieveMultipleRecords` function to retrieve multiple records based on specific criteria.
3. Utilize the `UpdateRecord` function to update existing records in CRM.
To make API requests to the Web API, you can use JavaScript’s XMLHttpRequest or a library like axios. The Web API provides detailed documentation and examples for each function, allowing you to easily retrieve multiple records from CRM using JavaScript.
Handling Pagination and Large Result Sets
When dealing with large result sets in CRM data retrieval, it’s crucial to handle pagination effectively. Pagination allows you to retrieve records in chunks rather than retrieving all records at once, which can be resource-intensive and affect performance. Here are some techniques to handle pagination in JavaScript when retrieving multiple records:
1. Use the `top` and `skip` OData query options to retrieve a specific number of records and skip a certain number of records, respectively.
2. Keep track of the `nextLink` property in the response data when retrieving records using the Web API. This property contains the URL for the next page of results.
3. Implement a recursive function or loop to iterate through all pages of results until all records are retrieved.
By implementing these techniques, you can efficiently retrieve large result sets without impacting the performance of your CRM system.
Error Handling and Exception Management
When working with CRM data retrieval in JavaScript, it’s essential to handle errors and exceptions effectively. Common errors and exceptions that you may encounter include network failures, authorization issues, or invalid query syntax. Here are some techniques for error handling and exception management in JavaScript:
1. Use try-catch blocks to catch and handle specific exceptions thrown during record retrieval.
2. Implement error handling mechanisms, such as displaying meaningful error messages to users or logging errors for further investigation.
3. Utilize debugging tools and techniques, such as browser console logs or remote debugging, to troubleshoot and identify the cause of retrieval issues.
By adopting these techniques, you can ensure that your CRM data retrieval process is robust and reliable, providing a smooth user experience and minimizing disruptions.
FAQs
Q1: What is the difference between fetching a single record and retrieving multiple records in CRM using JavaScript?
A1: When fetching a single record, you typically use the `RetrieveRecord` function in the Web API, passing the unique identifier of the record as a parameter. On the other hand, retrieving multiple records involves using query options such as `RetrieveMultipleRecords`, FetchXML, or OData to retrieve records that meet specific criteria.
Q2: Can I update records retrieved from CRM using JavaScript?
A2: Yes, you can update records retrieved from CRM using JavaScript. The Web API provides the `UpdateRecord` function, allowing you to modify the attributes of existing records. You can also use FetchXML or OData to retrieve records, make the necessary modifications, and then update them using the Web API.
Q3: Are there any limitations or unsupported functionalities when retrieving multiple records from CRM using JavaScript?
A3: While JavaScript offers numerous options for retrieving multiple records from CRM, there are certain limitations and unsupported functionalities to be aware of. For example, not all query functions supported by the Web API are compatible with standard OData. It’s important to refer to the documentation provided by Microsoft to ensure compatibility and functionality when retrieving records from CRM using JavaScript.
In conclusion, retrieving multiple records from CRM using JavaScript requires a good understanding of various query options such as FetchXML, OData, and the Web API. By leveraging these methods, handling pagination efficiently, and adopting effective error handling techniques, you can retrieve records from CRM smoothly and reliably. Whether you’re a developer building custom CRM applications or an administrator managing data exports, these techniques will prove invaluable in your CRM data retrieval endeavors.
Lesson272 – Model Driven App Javascript Retrieve Multiple Record- Power Apps 1000 Videos
How To Retrieve Multiple Records In Plugin?
Plugins are essential tools for enhancing the functionality of software applications. One common requirement when developing plugins is the need to retrieve multiple records from a database or external source. This article will guide you through the process of retrieving multiple records in plugins, providing insights into best practices and potential challenges you may encounter along the way.
Understanding the Basics
When retrieving multiple records in a plugin, the first step is to identify the source of the data. This can be a database table, a web service, or any other data store. Once you have determined the source, you can proceed with retrieving the records using a suitable method.
Methods for Retrieving Multiple Records
1. SQL Queries: If your data is stored in a relational database, SQL queries are an efficient way to retrieve multiple records. You can construct a SELECT statement with appropriate filters and sorting conditions to retrieve the desired records. It is essential to sanitize and validate user inputs to prevent SQL injections.
2. Web Service Calls: In scenarios where data is available through an external API or web service, you can utilize methods provided by the service to retrieve multiple records. These APIs often offer parameters for filtering, sorting, and pagination, enabling you to fetch specific datasets as needed.
3. RESTful Endpoints: If your plugin operates in a web environment, you can leverage RESTful endpoints to retrieve multiple records. These endpoints expose resources that can be queried to access the desired data in a structured manner.
4. File System Access: Sometimes, the records you need may be stored in files rather than a database. In such cases, you can use file system access methods provided by your programming language to retrieve the necessary records.
Filtering and Sorting Records
Retrieving multiple records often involves applying filters and sorting criteria to narrow down the dataset. This allows you to fetch only the required records, improving performance and reducing resource usage. Common filtering techniques include searching by keywords, applying date-based filters, or querying specific data fields. Furthermore, sorting records by one or more fields can help present the data in a meaningful order.
Iterating Through Retrieved Records
Once you have retrieved the records, you’ll typically need to process them one by one or in batches, depending on your specific requirements. This can be done using loops or by applying functional programming techniques, such as utilizing lambda expressions or callbacks.
Best Practices and Considerations
1. Optimizing Performance: To improve performance, consider retrieving only the necessary fields rather than fetching the entire record. This minimizes unnecessary data transfer and processing. Additionally, if you are dealing with a vast number of records, implementing pagination techniques can help limit retrieval sizes and reduce processing times.
2. Error Handling: It is crucial to handle possible exceptions and errors that may occur during record retrieval. This ensures that your plugin gracefully handles any unexpected issues and provides meaningful error messages to users. Proper error handling also helps in debugging and resolving problems quickly.
3. Data Validation: When working with user input, it is essential to validate and sanitize data to avoid security risks, such as SQL injections or cross-site scripting attacks. Always validate inputs, sanitize filtering criteria, and use parameterized queries to prevent such vulnerabilities.
Common Challenges and Solutions
Q: What should I do if I encounter a large dataset that exceeds memory limitations?
A: If the dataset is too large to retrieve and process at once, you can implement paging techniques using offsets and limits. Fetch a smaller subset of records at a time and iterate through them in batches.
Q: How can I efficiently retrieve related records from multiple tables or sources?
A: In scenarios where data is distributed among multiple tables or sources, you can use appropriate JOIN statements in SQL or combine data using programming constructs to retrieve and link related records.
Q: What if the external source I need to retrieve records from does not provide a suitable API or interface?
A: In such cases, you may need to develop custom adapters or connectors to establish a connection with the external source and retrieve the required records. This requires understanding the source’s protocols, data formats, and APIs.
Conclusion
Retrieving multiple records in a plugin is a crucial task in several software development scenarios. By understanding the fundamentals, utilizing appropriate methods, and following best practices, you can efficiently retrieve the desired data and enhance the functionality of your plugins. Remember to handle errors, optimize performance, and validate data to ensure the security and reliability of your plugins.
How To Get Record Id Using Javascript In Dynamics 365?
Dynamics 365 is a powerful customer relationship management (CRM) and enterprise resource planning (ERP) solution offered by Microsoft. It allows businesses to manage their customer data, sales processes, and various other operations efficiently. JavaScript is a widely used scripting language that can be utilized to extend the functionality of Dynamics 365. In this article, we will explore how to get the record ID using JavaScript in Dynamics 365 and provide a comprehensive guide to help you with the process.
Getting the record ID is essential when you need to perform specific actions or retrieve data associated with a specific record in Dynamics 365. By using JavaScript, you can easily extract the record ID and utilize it in your customizations. To achieve this, we will discuss the recommended approach and provide step-by-step instructions.
Here’s how you can get the record ID using JavaScript in Dynamics 365:
Step 1: Understand the page context
Before diving into the JavaScript code, it’s crucial to understand the page context where you intend to retrieve the record ID. Dynamics 365 provides various entity forms and views where you can interact with records. Identifying the appropriate context will help you determine the most suitable method for retrieving the record ID.
Step 2: Access the Xrm object
In the JavaScript code, you will need to access the Xrm object, which provides a set of functions and properties to interact with Dynamics 365. The Xrm object is available globally and can be accessed from any part of the page.
Step 3: Retrieve the record ID
Once you have accessed the Xrm object, you can use the available methods to retrieve the record ID. The method that you choose will depend on the page context and where you want to extract the ID from. Here are three commonly used methods:
1. Xrm.Page.data.entity.getId(): This method retrieves the ID of the currently loaded record on the form.
2. Xrm.Page.data.entity.getEntityReference(): This method returns an object containing the ID, logical name, and name of the currently loaded record.
3. Xrm.Utility.getGlobalContext().getQueryStringParameters().id: This method extracts the record ID from the URL parameters.
Step 4: Utilize the retrieved record ID
Once you have successfully obtained the record ID, you can utilize it in various ways according to your business requirements. For example, you can use it to update related records programmatically, perform data validations, or even redirect to another form or view.
FAQs:
Q1: Can I use the same JavaScript code to retrieve the record ID in different parts of Dynamics 365?
A: Yes, the JavaScript code for retrieving the record ID can be used across different forms and views within Dynamics 365. However, you might need to adjust the code slightly depending on the context and where you want to extract the ID from.
Q2: Is it possible to retrieve the record ID of a record that is not currently loaded on a form?
A: Yes, you can retrieve the record ID of a record that is not currently loaded on a form by utilizing the available APIs in Dynamics 365. For example, you can use the Web API or FetchXML to query the system and retrieve the required record ID.
Q3: What are the possible use cases for retrieving the record ID using JavaScript?
A: There are several use cases where retrieving the record ID can be beneficial. Some common examples include performing custom validations, triggering specific business logic based on the record ID, and creating custom navigation functionalities.
Q4: Can I retrieve the ID of multiple records using JavaScript at once?
A: Yes, it is possible to retrieve the IDs of multiple records using JavaScript. However, you might need to customize the code according to your specific requirements. You can either iterate through a list of records or use appropriate APIs to fetch the desired record IDs in bulk.
Q5: Are there any security considerations when using JavaScript to retrieve the record ID in Dynamics 365?
A: While using JavaScript, it’s important to consider security aspects. Make sure to properly validate and sanitize the record ID before using it in any sensitive operations. Avoid exposing any confidential information through JavaScript code and adhere to the best practices recommended by Microsoft.
In conclusion, retrieving the record ID using JavaScript in Dynamics 365 is a straightforward process that can be accomplished by accessing the Xrm object and utilizing the appropriate methods. By following the steps mentioned in this article, you can seamlessly extract the record ID and incorporate it into your customizations. Consider the FAQs section for additional insights and precautions for a successful implementation.
Keywords searched by users: retrieve multiple records from crm javascript Retrieverecord, retrieve multiple records from crm c#, Update record dynamics 365 javascript, Dynamics 365 js reference, Getvalue crm js, Setvalue crm js, Get lookup value dynamics 365 javascript, Which query function that the Web API is not support standard OData string
Categories: Top 81 Retrieve Multiple Records From Crm Javascript
See more here: nhanvietluanvan.com
Retrieverecord
In today’s era of digital transformation, data has undoubtedly become the most valuable asset for businesses across industries. However, managing and utilizing this vast amount of information can be a daunting task without the right tools and strategies in place. This is where Retrieverecord comes into play – a cutting-edge data management software designed to revolutionize the way companies handle and leverage their data.
What is Retrieverecord?
Retrieverecord is an advanced data management platform that offers businesses a comprehensive and efficient solution for organizing, storing, and retrieving their crucial information assets. Its sophisticated database architecture and intuitive features provide users with the ability to streamline their data management processes, ensuring data accuracy, security, and accessibility at all times.
Key Features and Benefits
1. Data Organization: Retrieverecord enables businesses to consolidate all their data into a centralized repository, eliminating scattered or duplicate information. This helps companies maintain data integrity by minimizing errors resulting from inconsistent or outdated data.
2. Data Security: With the increasing threat of cybercrime, protecting sensitive business data is of utmost importance. Retrieverecord offers robust security measures, including encryption, user access controls, and regular backups, ensuring that confidential information remains safe and protected from unauthorized access.
3. Enhanced Accessibility: An efficient data management system should offer easy access to information. Retrieverecord excels in this aspect, providing users with quick and seamless retrieval of data through advanced search functionalities and customizable filters. This enables businesses to make informed decisions based on real-time and accurate data.
4. Integration Capabilities: Retrieverecord seamlessly integrates with various existing systems, such as CRMs or ERPs, enabling businesses to synchronize their data across multiple platforms without any hassle. This eliminates the need for manual data transfer and promotes data consistency across different departments.
5. Streamlined Workflows: By automating repetitive tasks and processes, Retrieverecord significantly reduces the time and effort spent on manual data management. This allows employees to focus on more value-added activities, leading to increased productivity and efficiency within the organization.
Frequently Asked Questions (FAQs):
Q: Can Retrieverecord be customized to our specific business needs?
A: Yes, Retrieverecord offers extensive customization options to tailor the software according to individual business requirements. Whether it’s data fields, workflows, or user access privileges, the platform can be configured to align with your unique needs.
Q: Does Retrieverecord work with both structured and unstructured data?
A: Absolutely! Retrieverecord is designed to handle a wide range of data formats, including structured data (such as spreadsheets or databases) and unstructured data (such as documents or multimedia files). This ensures that businesses can manage and retrieve all types of data within a single platform.
Q: How does Retrieverecord ensure data privacy and security?
A: Retrieverecord employs various security measures to safeguard your data. These include robust encryption algorithms, user access controls, role-based permissions, and regular backups. Additionally, the software complies with industry standards and regulations to ensure your data remains secure at all times.
Q: Can Retrieverecord be integrated with third-party applications?
A: Yes, Retrieverecord offers seamless integration capabilities with popular third-party applications, such as Salesforce, SAP, or Microsoft Office Suite. This allows businesses to connect their existing systems and synchronize data across multiple platforms, ensuring data consistency and eliminating data silos.
Q: Is Retrieverecord suitable for small businesses?
A: Absolutely! Retrieverecord is designed to cater to businesses of all sizes, including small and medium enterprises. It offers flexible pricing plans, scalable solutions, and user-friendly interfaces, making it accessible and suitable for organizations regardless of their size or level of technical expertise.
Conclusion
Retrieverecord is a game-changer in the field of data management, providing businesses with a powerful platform to efficiently organize, secure, and leverage their valuable information assets. With its advanced features, customizable options, and seamless integration capabilities, Retrieverecord empowers organizations to unlock the full potential of their data, leading to improved decision-making, streamlined workflows, and enhanced productivity. Whether you operate a small business or a large enterprise, Retrieverecord is the ultimate solution to optimize your data management processes and stay ahead in the digital age.
Retrieve Multiple Records From Crm C#
Introduction:
In today’s digital world, customer relationship management (CRM) systems play a vital role in managing and organizing customer data. Microsoft Dynamics CRM is one such popular CRM system that offers a wide range of functionalities to enhance customer interaction and improve business relationships.
One crucial aspect of CRM systems is the ability to retrieve multiple records efficiently and effectively. In this article, we will explore how to retrieve multiple records from CRM using C#, providing you with a comprehensive guide to mastering this essential skill.
Understanding CRM Record Retrieval in C#:
To retrieve multiple records from CRM using C#, we need to utilize the CRM SDK (Software Development Kit). The SDK provides a set of libraries and tools that enable developers to build robust custom solutions and interact with various CRM functionalities programmatically.
Step 1: Setting Up the Development Environment:
Before diving into CRM record retrieval, we need to set up our development environment properly.
1. Install Visual Studio: Download and install Visual Studio, a powerful integrated development environment (IDE) that facilitates C# development.
2. Install CRM SDK: Next, download and install the latest version of the Dynamics 365 SDK from the official Microsoft website. This SDK includes the required assemblies and tools for CRM development.
3. Create a new C# project: Launch Visual Studio, create a new C# project, and choose the appropriate project type based on your requirements.
Step 2: Connecting to the CRM Organization:
In order to retrieve records from CRM, establishing a connection to the CRM organization is crucial. The connection can be established by using the “IOrganizationService” interface provided in the SDK.
Here’s an example of connecting to the CRM organization:
“`csharp
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Tooling.Connector;
// Set the connection string
string connectionString = “AuthType=OAuth;Url=https://yourcrmorg.crm.dynamics.com;ClientId=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx;RedirectUri=app://yoururl;Authority=login.windows.net;[email protected];Password=yourpassword”;
// Create the connection
CrmServiceClient service = new CrmServiceClient(connectionString);
// Verify the connection
if (service.IsReady)
{
// Connection successful
Console.WriteLine(“Connected to CRM!”);
}
“`
Make sure to replace the placeholders in the connection string with your actual CRM organization details.
Step 3: Retrieving Records:
Now that we have established a connection to the CRM organization, we can proceed with retrieving records.
To retrieve multiple records, we use the “QueryExpression” class from the SDK. The QueryExpression class allows us to specify criteria, sorting order, and other parameters for record retrieval.
Here’s an example of retrieving multiple records using QueryExpression:
“`csharp
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Query;
// Create a QueryExpression instance
QueryExpression query = new QueryExpression(“account”);
// Set criteria (e.g., retrieving accounts with an active status)
query.Criteria.AddCondition(“statuscode”, ConditionOperator.Equal, 1);
// Set sorting order
query.AddOrder(“name”, OrderType.Ascending);
// Set desired columns
query.ColumnSet = new ColumnSet(“name”, “accountnumber”, “revenue”);
// Retrieve the records
EntityCollection results = service.RetrieveMultiple(query);
// Process the retrieved records
foreach (Entity account in results.Entities)
{
// Access the record fields
string name = account.GetAttributeValue
string accountNumber = account.GetAttributeValue
Money revenue = account.GetAttributeValue
// Perform operations with the retrieved data
// …
}
“`
In the example above, we retrieve account records that have an active status, sort them by name in ascending order, and retrieve specific columns like name, account number, and revenue. The retrieved records are stored in the “results” variable, which can be iterated to access individual record details.
Frequently Asked Questions (FAQs):
Q: Can I retrieve records from custom entities?
A: Yes, you can retrieve records from both standard and custom entities in CRM using the same QueryExpression class. Just replace “account” in the example with the logical name of your custom entity.
Q: How can I paginate the retrieved records?
A: To paginate the results, you can set the “PageInfo” property in the QueryExpression instance. This property allows you to specify the number of records per page and the desired page number.
Q: Is it possible to retrieve records based on complex criteria?
A: Yes, the QueryExpression class provides various condition operators, such as GreaterThan, LessThan, Contains, etc., to build complex criteria for record retrieval.
Q: Can I retrieve related records?
A: Yes, you can retrieve related records using the “LinkEntities” property of the QueryExpression. This allows you to traverse and retrieve records from associated entities.
Conclusion:
Retrieving multiple records from CRM using C# is a crucial skill for CRM developers. By utilizing the CRM SDK and following the steps outlined in this comprehensive guide, you can effectively retrieve and process records from CRM organizations. With this newfound knowledge, you’ll be able to harness the power of CRM systems and build advanced custom solutions to enhance customer relationship management in your organization.
Images related to the topic retrieve multiple records from crm javascript
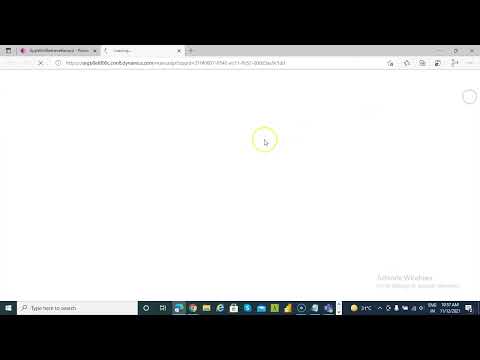
Found 39 images related to retrieve multiple records from crm javascript theme
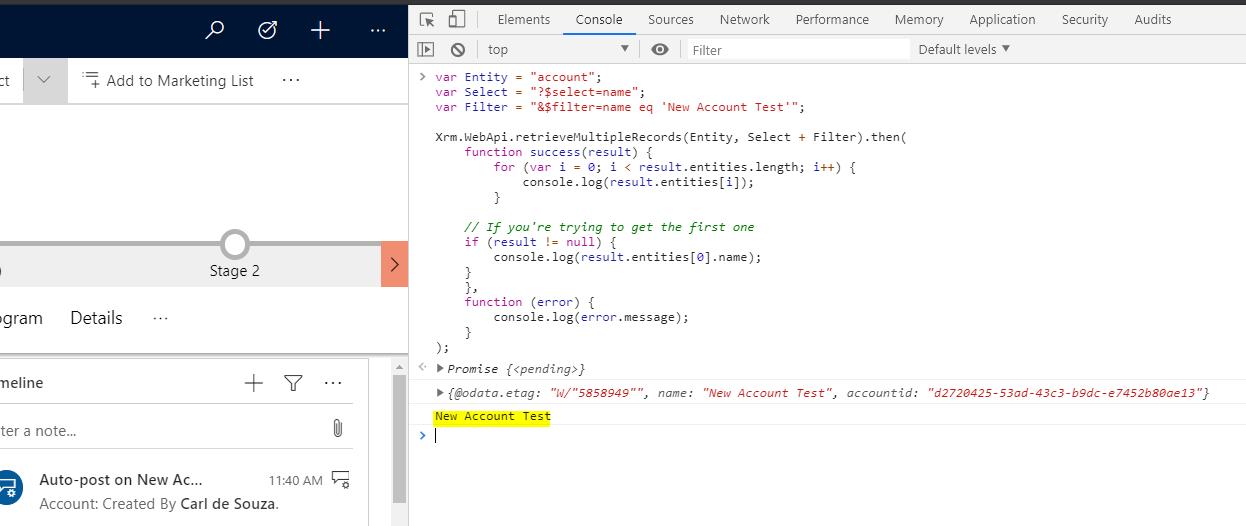

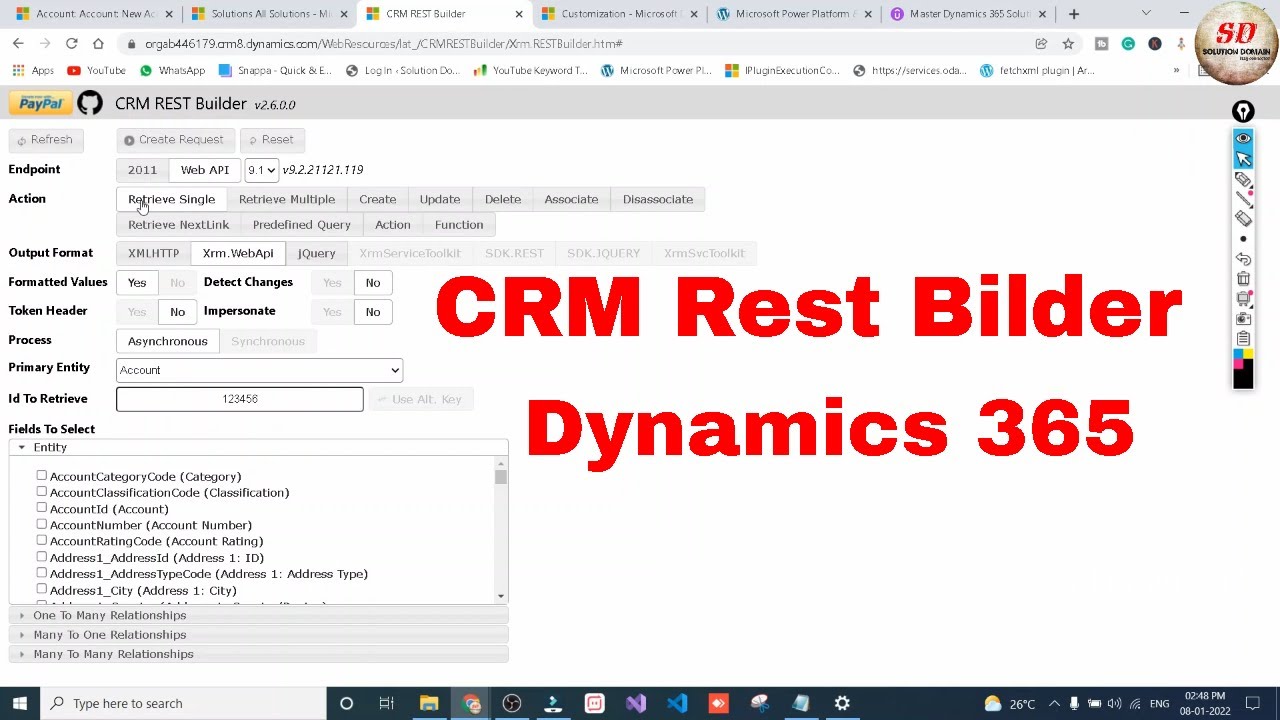
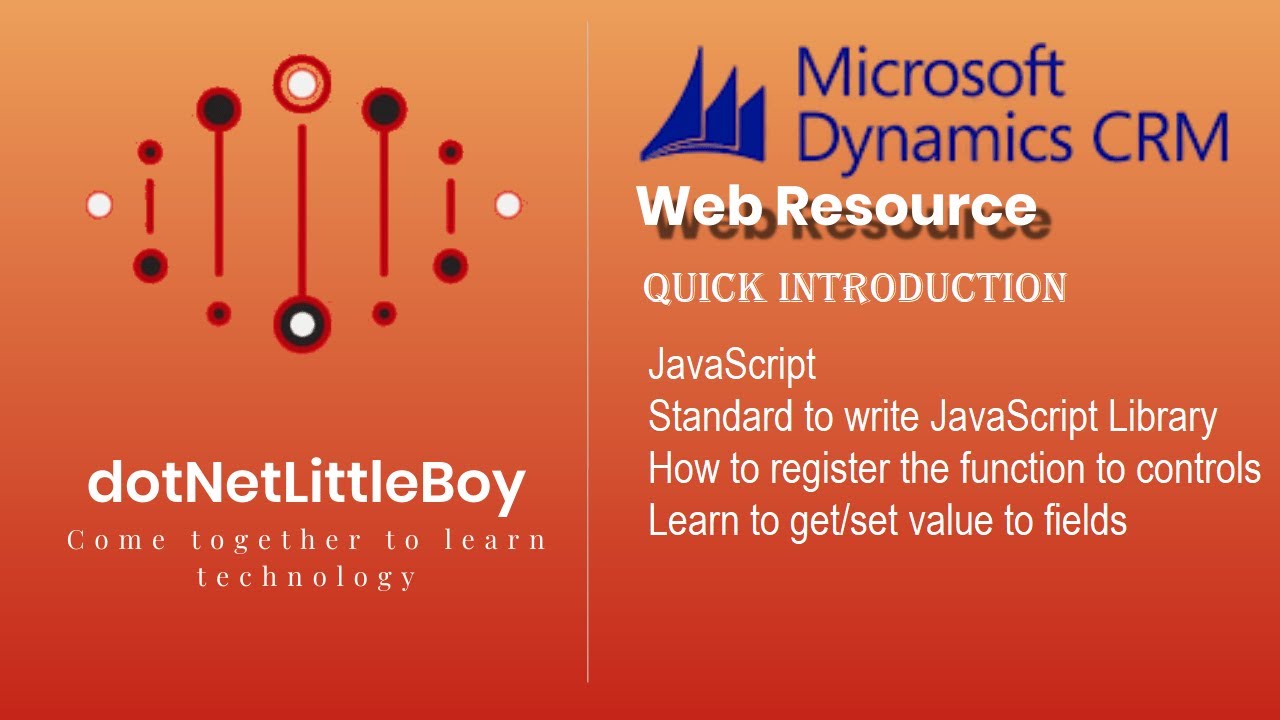







.png)

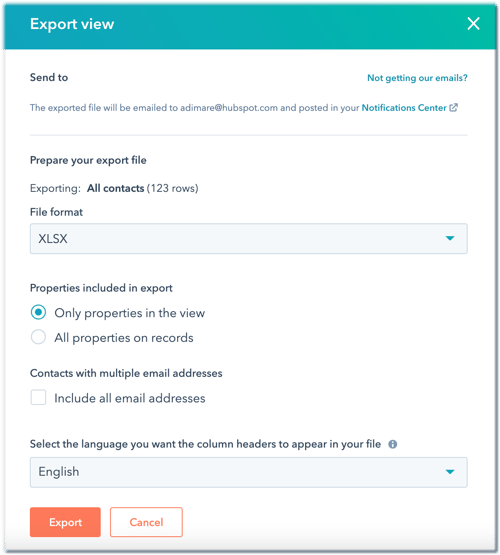
Article link: retrieve multiple records from crm javascript.
Learn more about the topic retrieve multiple records from crm javascript.
- retrieveMultipleRecords (Client API reference) – Microsoft Learn
- Retrieve and RetrieveMultiple JavaScript using Xrm.WebApi
- Dynamics 365 – Retrieve Multiple Records with WebApi and …
- Retrieve multiple records in CRM Dynamics 365 version 8.2 …
- {Dynamics CRM} How to execute RetrieveMultiple request …
- Retrieve Multiple Records using Web API in Dynamics 365 …
- Retrieve Multiple Records- Dynamics 365
- How to retrieve multiple records in plugin using Query Expression
- Get the current record ID using JavaScript in D365 CE – Vblogs
- Dynamics 365 – Retrieve More than 5000 records with FetchXML
- Executing Fetch XML With WebAPI In Dynamics 365 Using JavaScript
- Filter by Lookup with retrieveMultipleRecords JavaScript …
See more: nhanvietluanvan.com/luat-hoc